Getting Started With Smart Contracts in Solidity | Web 3.0
Skeptical about the insane blockchain hype? Maybe you don't know what a smart contract does? Are you feeling lost regarding NFTs and how blockchains work? Perhaps you're unsure where to start in the sea of immense knowledge? This is it. Trust me; you're going to want to read and learn why web 3.0 and blockchains are the future-- this is the post for you.
This is the post you are looking for. In this article, you will learn how to create your first-ever smart contract (yay!), compile and then deploy it! For this post, I will use Solidity to write the smart contract (you may also use something like Vyper, which shares its syntax with Python).
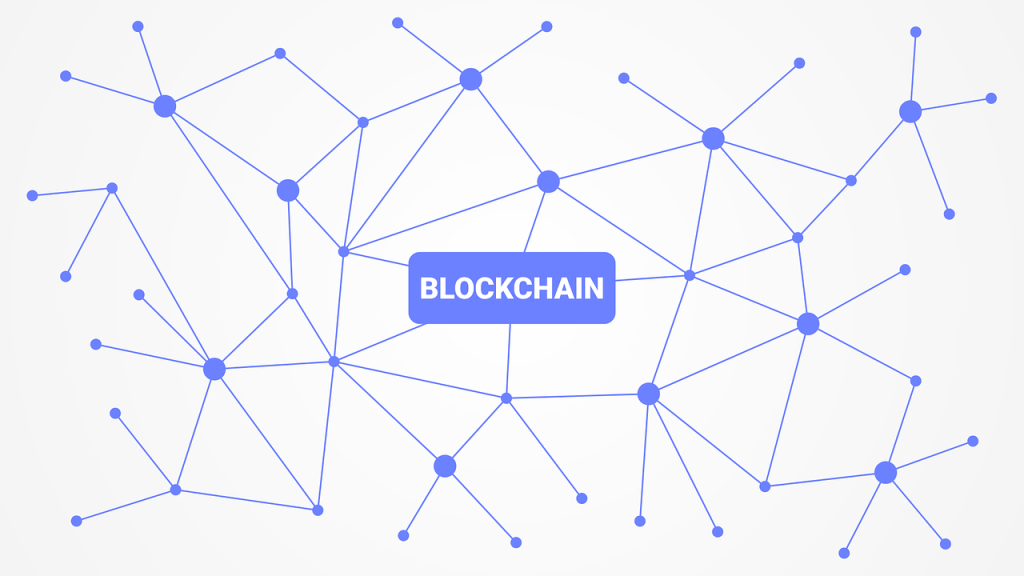
Let's dive right into the technical scope and introduction for blockchain-- the future of the Internet (web 3.0).
What is a Blockchain?
What is a blockchain, you may wonder?
Honestly, I remember being a bit confused about the concept. I wasn't sure what the lingo meant despite understanding what it was about. Until I decided to explore the blockchain world, and I realized the greatness of this idea.
Technically, a blockchain is a decentralized, digitally distributed database to store transactions across a vast network of systems or nodes. A blockchain consists of countless peers of blocks; blocks are a list of mined transactions. In simple terms, a blockchain is an immutable digital ledger.
Why is That Useful For You?
Blockchains are essentially based on these properties:
- Immutable: this means no changes can be made to a block after creation-- its nonce changes once a modification is made to the original data or transactions.
- Secure: immutability and use of consensus helps with security
- Decentralized: no central entity controls access
- Deterministic: the same function run across different nodes returns the same result
- Trustless: contract agreements don't have to be trusted upon to fulfil them; instead, they are always fulfilled when the conditions are met.
Therefore, blockchain technology is not a part of a Ponzi scheme or a scam; this technology is legit revolutionary. Moreover, blockchains operate using either a Proof of Work or Proof of Stake.
What is a Cryptocurrency?
What is a cryptocurrency, and why is it suddenly the most incredible thing? Let's explore this below. Cryptocurrency is a digital payment system that requires verification from a third party, like a bank or other institution. To make transactions, you exchange digital assets with a peer.
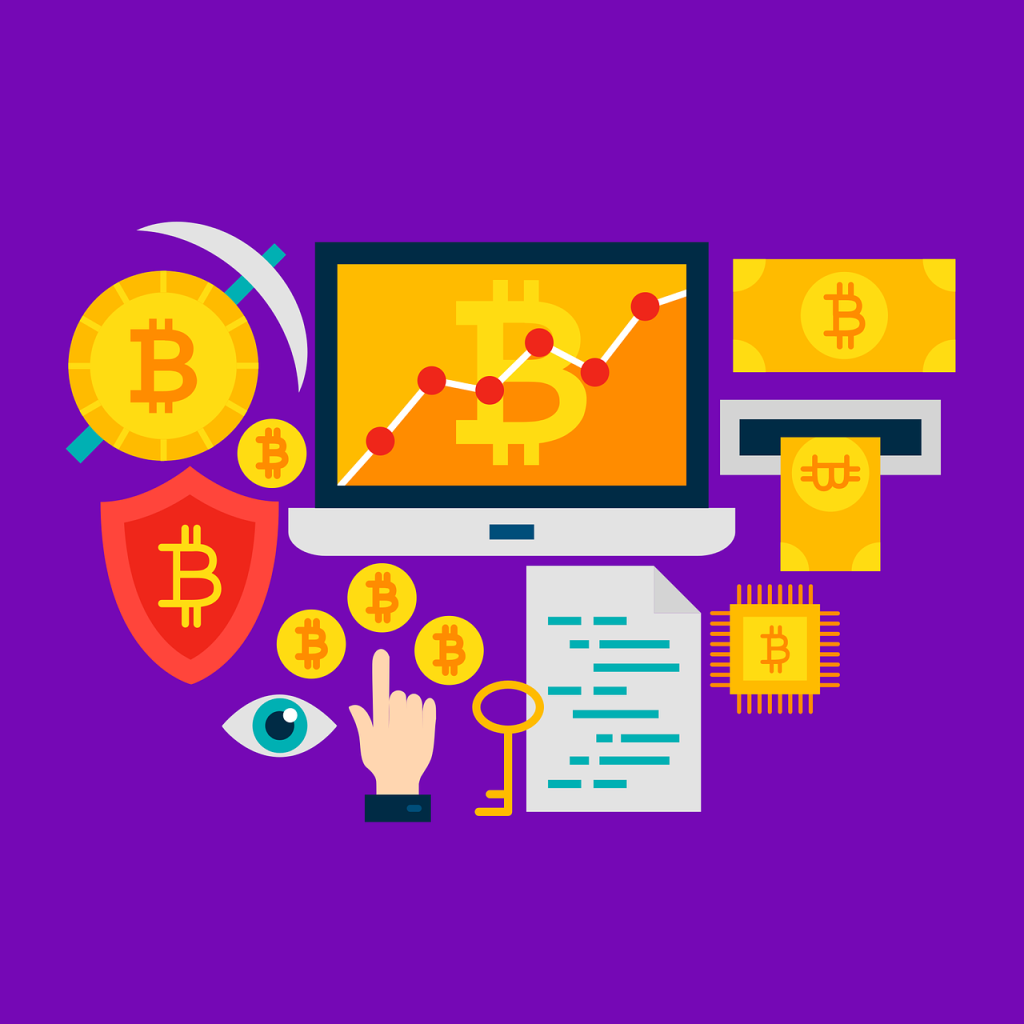
Cryptocurrency is widely hailed as the future currency because of its independent nature. Cryptocurrency consists of many coins or digital currencies like Bitcoin, Ethereum, Dai, USDT, etc.
Bitcoin is worth a whopping $58k, while Ethereum is worth $5k a coin.
The benefits offered by cryptocurrencies consist of:
- Faster international payments
- Freedom of monetary choices
- 24/7 access to your digital assets
- Stronger security
However, some people-- a minority-- still perceive cryptocurrency as a pyramid scheme or a fraud. Its volatile nature is particularly highlighted with coins like Bitcoin that suffer heavy dips and exponentially stark peaks in a short period. This is especially dangerous if you're using a cryptocurrency like Bitcoin for your business as the prices of goods will vary dramatically from day to day-- sometimes even hourly.
What is a Smart Contract?
Smart contracts are akin to real-life contracts; they execute automatically when certain conditions fulfil. They are instrumental and widely used today in blockchain implementation.
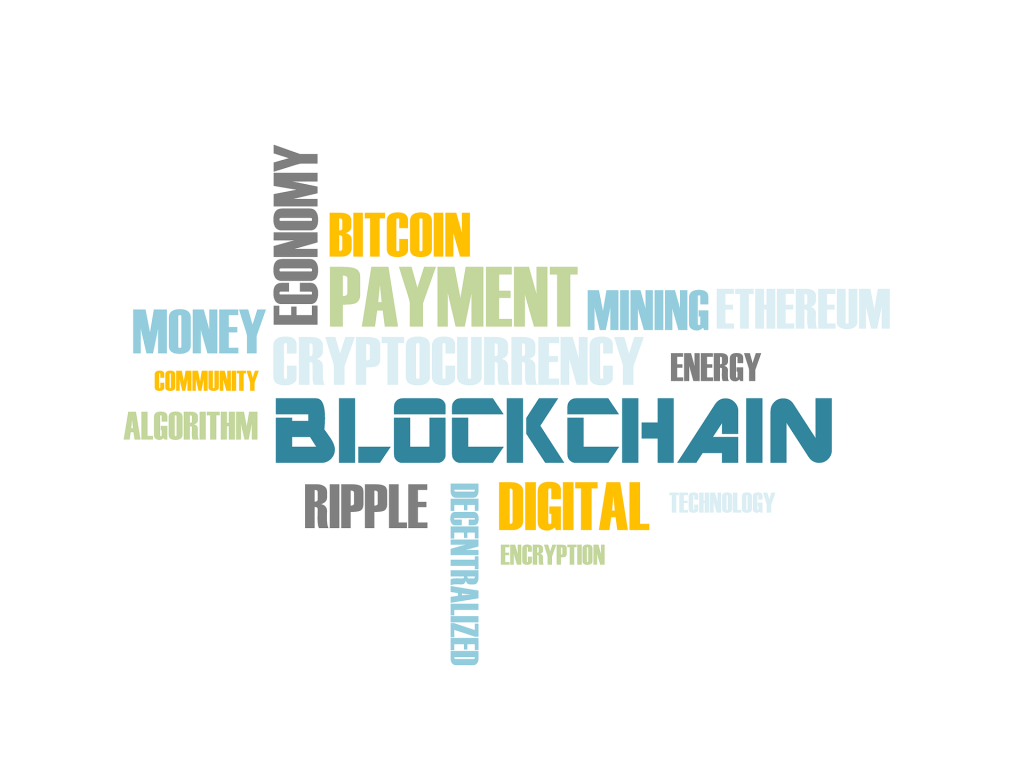
Often, smart contracts pair with an oracle network, an operator that provides off-chain data from the real world securely to the blockchain. The data received is from multiple sources to ensure the blockchain doesn't violate its core principle-- decentralized nature.
Don't be intimidated-- a few months ago, I had no clue about what any of this meant, honestly. Not a blockchain, smart contract. However, I spent the following weeks exploring and learning this fantastic futuristic solution. It can be hard to figure out where to start, but I recommend diving into smart contracts.
Why? Smart contracts are the building blocks of the blockchain world, no pun intended.
In addition, a smart contract is a solution to trust violations from people you expect to hold up their end of the agreement. They ensure you never have to trust someone-- hence blockchain can be trust-less.
Setting Up Your Development Environment For Smart Contract in Solidity
- REMIX: an IDE for Ethereum development.
- Metamask: a digital wallet; commonly used for all Ethereum applications.
One of the first things you need to set up before writing your first smart contract in Ethereum is to get Metamask. It's an app on your phone or a browser extension to create an account. You must never share the secret seed phrase key with anyone else and secure it safely. This is essential if you wish to keep your funds. However, we recommend you do not deposit any real money in this account for development purposes. The beauty of Metamask is that you can create multiple accounts with their private keys secured by your seed phrase key. Create a new account on Metamask and name it something related to development, so you don't forget the purpose and accidentally deposit tangible digital assets to that account.
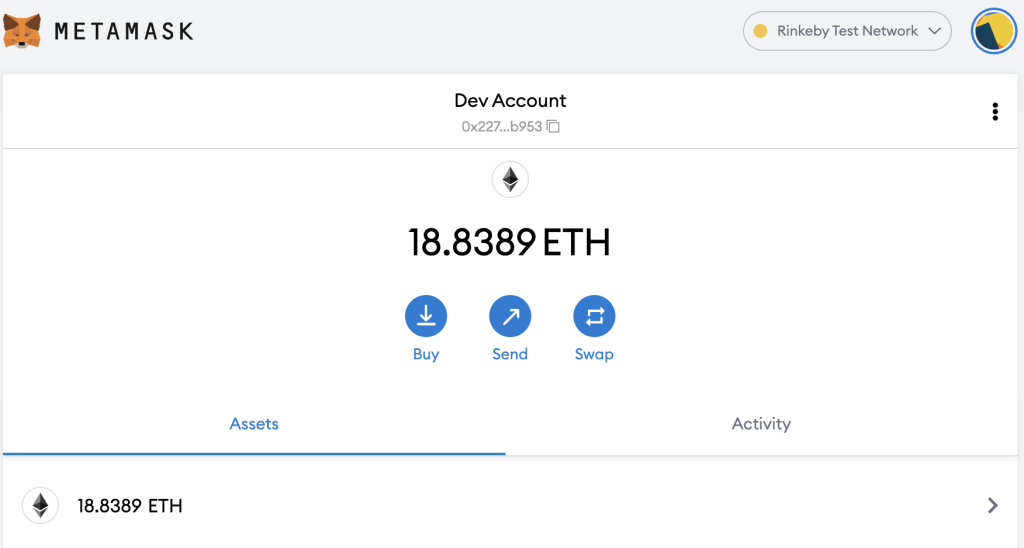
Installing Solidity(MacOS)
Copy and paste this command in your ssh terminal on macOS to install the Solidity Compiler:
brew update
brew upgrade
brew tap ethereum/ethereum
brew install solidity
Prerequisites: Install Homebrew with this command:
mkdir homebrew && curl -L https://github.com/Homebrew/brew/tarball/master | tar xz --strip 1 -C homebrew
After that, do this:
eval "$(homebrew/bin/brew shellenv)"
brew update --force --quiet
That's it! You have successfully begun writing your first smart contract! Pat yourselves if you made it this far. You have earned it!
Setting up REMIX IDE:
REMIX is an Integrated Development Environment (IDE) to code your logic. These logics are necessary to develop to understand the levers of blockchains. In other words, REMIX is a tool you will use to write your smart contracts for various applications such as DAPPs, DEXs, DEFIs, etc.
Hence, you can download the application or open it in your browser to start using Remix. I prefer to download and use it locally.
Test Net
A test net is like the Ethereum main net, but instead of making changes to the actual Ethereum blockchain, you can test the smart contracts safely on a test net first. Consequently, you don't pay gas fees, and you've not incurred any extra cost in case the smart contract doesn't work as intended.
There are various types of test net- Rinkeby, Kovan, etc.
Using a test net is helpful because this is a smart demo contract, and we don't want to post it on the Ethereum blockchain. Instead, a test net allows the freedom to play with the code and learn from it without having to pay the enormously high Ethereum transactional gas fees. Hence, you can deploy the smart contract for free.
Rinkeby Faucet:
For development purposes, it is recommended that you work with a test net not to post any gas fees and prevent making changes to blockchain. Head to a working Rinkeby faucet, and request some Ethereum onto your Rinkeby test net.
Writing Your First Smart Contract
Smart contracts are primarily written in Solidity, but another language is still in infancy-- Vyper, which resembles Python. All Vyper code must be syntactically Pyrhon3 code while the other way is not necessary.
We will use Solidity for writing the smart contract on Remix IDE for this tutorial.
Let's write a simple contract that stores a number to be retrieved later.
How To Write A Smart Contract: Simple Storage
When you open Remix IDE, click on Solidity to select the compiler.
After that, create a new file with a .sol extension (.sol for Solidity; Solidity is used to deploy or execute smart contracts).
Name this "SimpleStorage.sol". Note that the file type for smart contracts written in Solidity is ".sol".
Then, the first thing you need to do before beginning to write the smart contract is declared the license.
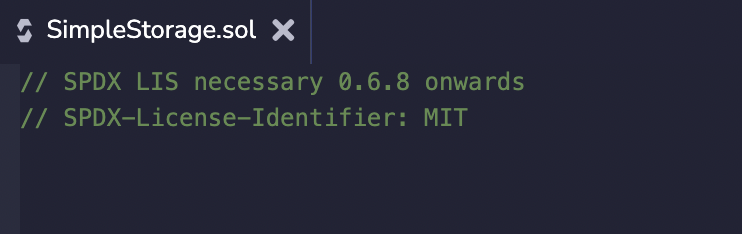
Afterwards, define the solidity version for the smart contract. Usually, in newer versions, some functionalities may break, so it is recommended to strictly define the versions your smart contract will support strictly.
// all solidity versions from 0.6.0 to 0.9.0
pragma solidity >= 0.6.0 <= 0.9.0
// all solidity versions of 0.6.x
pragma solidity ^0.6.0
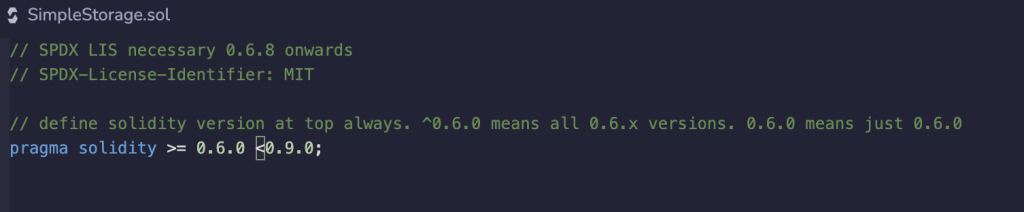
After that, it's time to declare your first contract in Solidity. A contract is similar to a class in Object-Oriented Programming (OOP). Contracts contain data as state variables and functions to modify them.
The use of contract keyword in Solidity is quite similar to how a class may be used; name this contract aptly for readability purposes.
It is good practice to write camel-case for the contract names here.
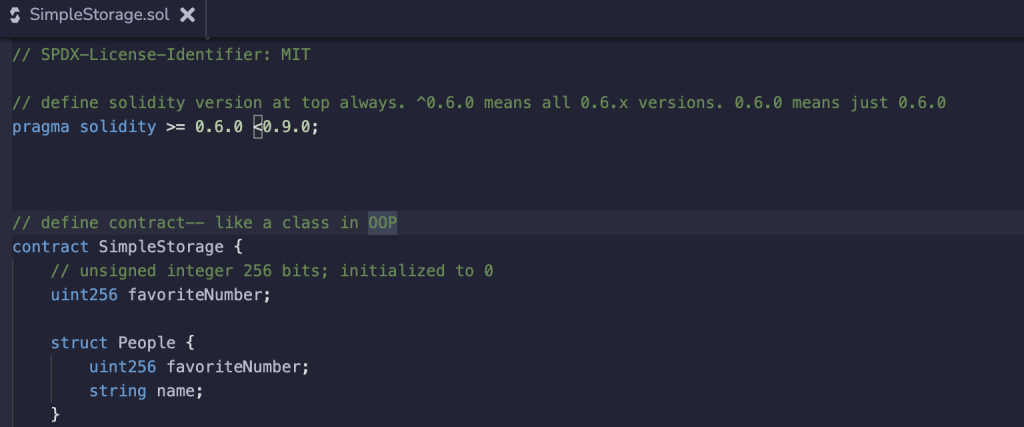
Note: the closing curly braces at the end are for the struct, not contract. The contract will be used till the end of this smart contract.
Variables in Solidity: First Smart Contract
A variable stores the reference to a specific address in the memory to make it easier to reuse. Variables are used to store data; they may be of several types.
General Syntax for Variables in Solidity
The following is the general syntax for defining variables in Solidity:
// data_type scope variable_name
uint256 public favoriteNumber
Moreover, by default, the scope is internal-- if not specified.
Types of Variables Scopes:
The following list contains the types of scopes:
- public: accessed via contract and through messages
- internal: accessed only from inside agreements and contracts derived from it
- private: accessed only from inside contract
- external: accessed only from outside, smart contract
uint256 specifies that the value can only be positive and of the length 256 bits. Moreover, this variable is public, which means the following functions can access it.
The next step is not mandatory unless you want to explore the possibilities of this smart contract, SimpleStorage. In other words, a struct is a user-defined datatype to create records; it's like an array, but instead, you can group items or properties for a particular object. Let me explain with an apt example: imagine you own a library full of books, right? Now, you may wish to note down the title, book id, author name, release year for all books. This is where a strut or structure comes into play. Define a struct named People. Inside this, define two variables; favorite number and name for the people.
struct People {
uint256 favoriteNumber;
string name;
}
Therefore, now we have created a data type defined as People, which will be helpful a little later on.
How will we use this new data type?
Arrays in Solidity
Arrays store lists of objects. In other words, arrays are used to multiple values. The way to link this with struct is by initializing an array or collection of data type People.
People[] public person;
An array of struct data types allows us to store its containing variables for multiple people's data. In turn, this will enable us to store data for the smart contract categorically.
Functions in Solidity
Functions or methods are self-contained modules in Solidity that execute the defined tasks.
This is the general syntax for defining a function in Solidity:
// function func_name(variables passed) scope
Now, it's time to create a function to store numbers from people.
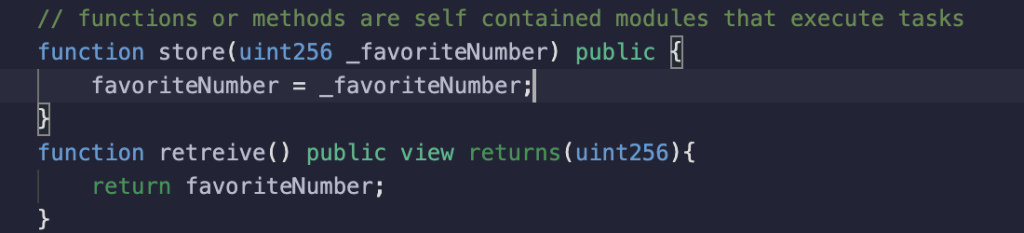
Hence, define the function store (which stores someone's favourite number), passing a variable to store a number input from the user. Assign the favourite number variable created above (with public scope) to the local variable passed in the function.
This is great, but how can we return this number to see if stored correctly?
Retrieve() Function
After that, create another function. Let's call this retrieve since the purpose of this function is to get the stored number. However, there is no need to pass a parameter in this function. Furthermore, it's pretty interesting that this is a view; a view is a non-state changing function. In other words, views don't make state changes and hence don't affect the blockchain. Lastly, a return type of uint256 (same as the datatype stored) must be defined explicitly.
In the body of this function, return the favorite number.
Bonus Content:
Hooray! You have made it this far. Incredible. Doesn't this rush feel great? You wrote your first smart contract. However, if you want to keep going, let's do that-- you can skip it for now if you're going to grab a coffee or something.
After that, create a new function addPerson, while passing two parameters; name and favourite number. Set the scope to public and inside the body of this function, use the "push()" function for arrays to push values into the People array for favourite number and name.

The last line is a mapping; write this code above the function.
mapping (string=> uint256) public nameToFavNum;
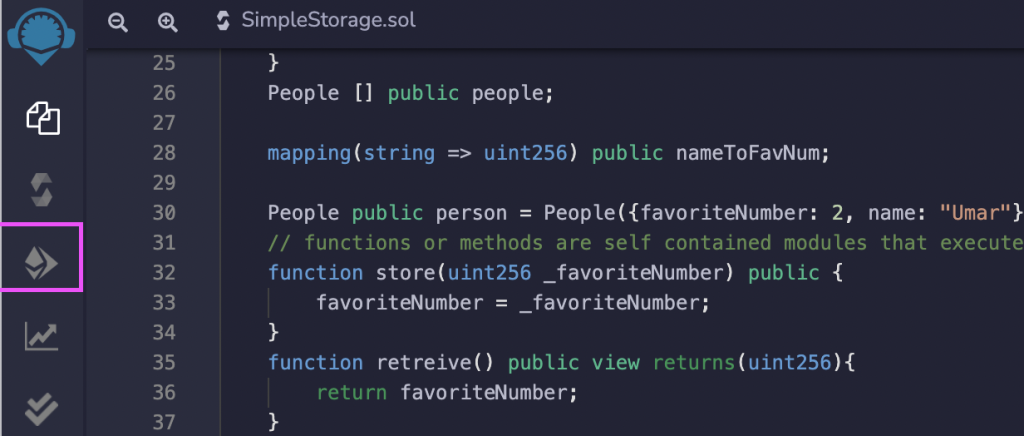
A mapping converts one data type to another, using it for reference.
The line converts the user's name to the favourite number in the smart contract above.
Compiling Smart Contracts in REMIX IDE
Since Solidity is a compiled language, it is essential to compile the smart contract before proceeding. This means converting the code you just wrote into machine code (even containing some assembly language).
This is an essential prerequisite for deploying a smart contract.
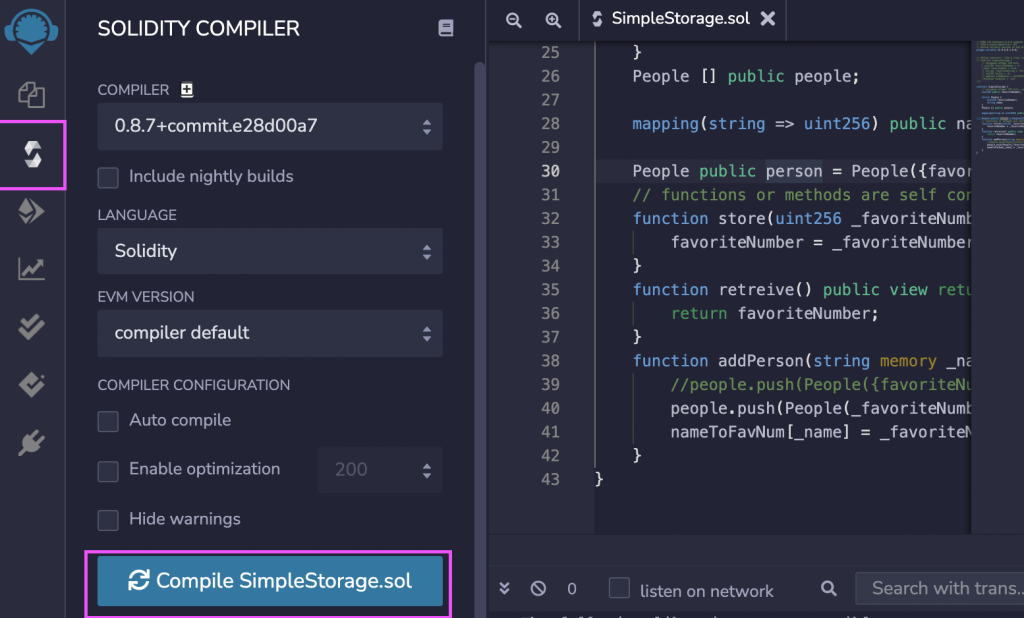
Deploying Smart Contract in EVM
Deploying a smart contract means running it no a VM or live connection. It means the smart contract is enabled, and the user may work with it. Smart contracts are initially deployed on test-nets to check for bugs and avoid unnecessary costs.
To deploy, head over to the sidebar on REMIX IDE and click on the icon in the purple box:
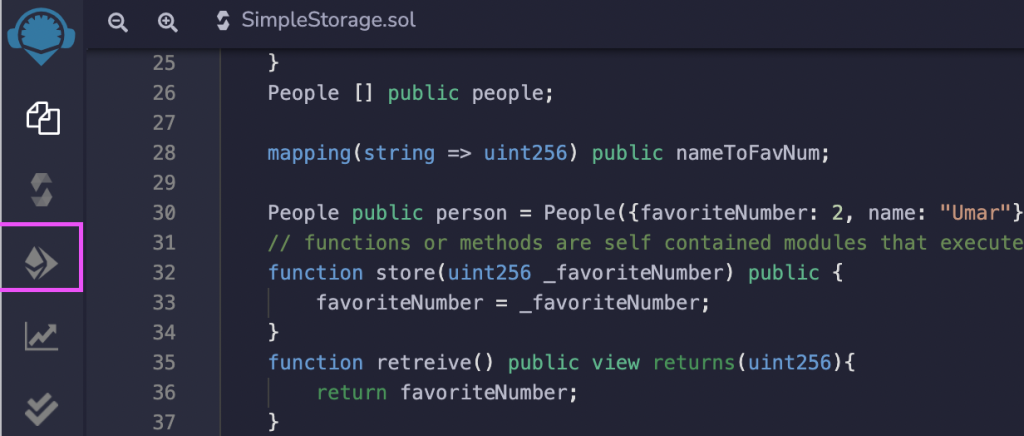
After that, click the deploy button.
Then, scroll down and click on the deployed contracts to see a droppable of all the states.
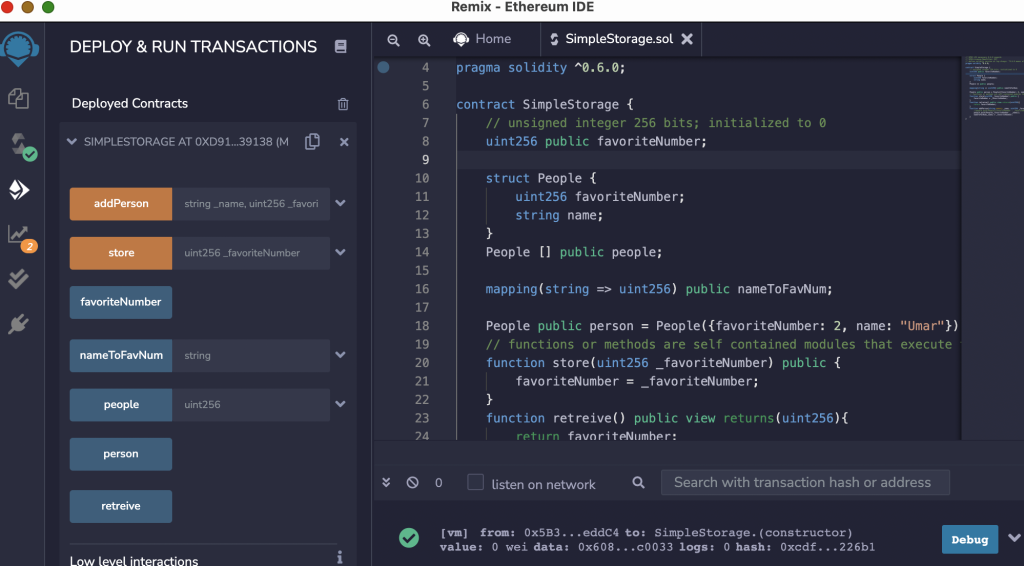
To demonstrate the work, enter a random value in the store field and click on the orange button (orange implies a state change-- a transaction). Subsequently, click on the blue retrieve button (no state change). You will see the same number you entered if you did everything right.
Note: the colors of these buttons are crucial.
- A blue button indicates no state change or no transaction made on the blockchain on a function call.
- An orange button indicates a state change or transaction made on the blockchain on a function call.
There you go! You've successfully managed to write your smart contract!
Conclusion:
I hope you found this post helpful in helping you write your first smart contract in Solidity. Be proud of yourself for accomplishing this. You're one step closer to becoming a blockchain developer.
After following this post, I recommend exploring Solidity and writing a smart contract on your own.
Drop a like and let us know if you like this type of content in the comments so we can create more. We'd love to hear if you want to see similar stuff in the future and explore this fantastic path ahead that we call Web 3.0-- the revolution. If you're going to explore more posts, go here.