Advanced Techniques and Further Optimizations
In our previous post, “Unravelling the Art of Dynamic Programming in Go: Mastering the Fibonacci Sequence”, we explored the basics of dynamic programming and implemented the Fibonacci sequence in Go using a straightforward approach. Now, let’s dive deeper into some advanced techniques and further optimizations to make our solution even more efficient.
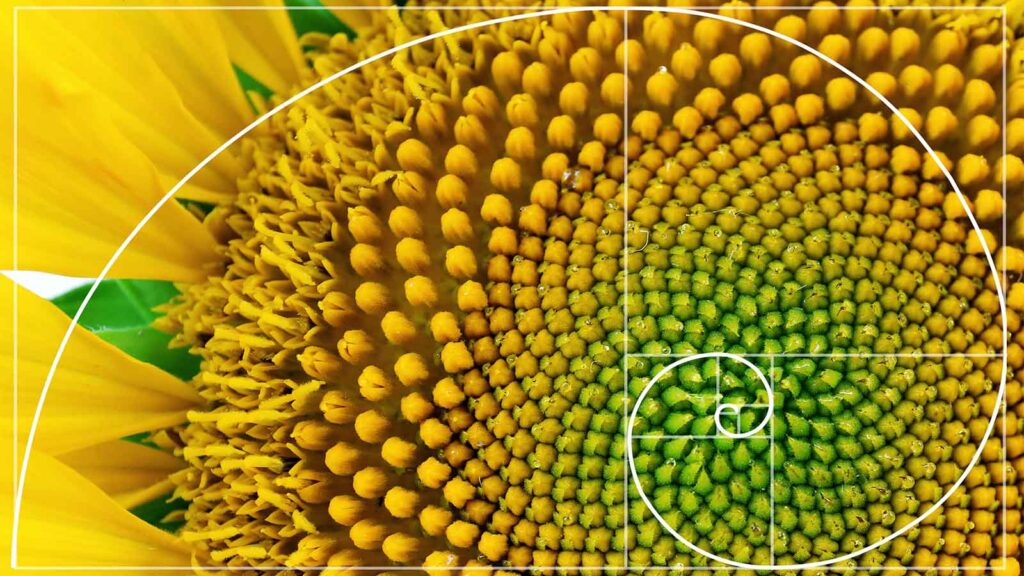
Using Iteration Over Recursion
While recursive solutions can be elegant and easy to understand, they can also be inefficient due to the overhead of multiple function calls. One way to optimize our Fibonacci sequence function is to adopt an iterative approach, which can be significantly faster.
func fib(n int) int {
if n < 2 {
return n
}
a, b := 0, 1
for i := 2; i <= n; i++ {
a, b = b, a + b
}
return b
}
Here, instead of relying on the recursion, we loop until we reach the nth Fibonacci number. The variables `a` and `b` store the last two numbers of the sequence, updating their values iteratively.
Optimizing Space Complexity
Even with the iterative solution, we are storing just two variables (`a` and `b`), which already brings our space complexity down to O(1). But what if you need to store the whole sequence for some reason, like performing additional computations later?
func fibSequence(n int) []int {
if n == 0 {
return []int{0}
}
fibSeq := make([]int, n+1)
fibSeq[0], fibSeq[1] = 0, 1
for i := 2; i <= n; i++ {
fibSeq[i] = fibSeq[i-1] + fibSeq[i-2]
}
return fibSeq
}
This version of the function stores the whole sequence in a slice, making it available for further calculations. It balances both time and space complexity while keeping the entire sequence accessible.
Using Matrix Exponentiation for Fibonacci
One of the lesser-known but highly efficient methods for calculating Fibonacci numbers is matrix exponentiation. This approach brings the time complexity down to O(log n), making it ideal for very large numbers.
func matrixMult(a, b [2][2]int) [2][2]int {
return [2][2]int{
{a[0][0]*b[0][0] + a[0][1]*b[1][0], a[0][0]*b[0][1] + a[0][1]*b[1][1]},
{a[1][0]*b[0][0] + a[1][1]*b[1][0], a[1][0]*b[0][1] + a[1][1]*b[1][1]},
}
}
func matrixPower(matrix [2][2]int, n int) [2][2]int {
result := [2][2]int{{1, 0}, {0, 1}}
for n > 0 {
if n%2 != 0 {
result = matrixMult(result, matrix)
}
matrix = matrixMult(matrix, matrix)
n /= 2
}
return result
}
func fib(n int) int {
if n == 0 {
return 0
}
result := matrixPower([2][2]int{{1, 1}, {1, 0}}, n-1)
return result[0][0]
}
This technique leverages the power of matrices to compute Fibonacci numbers in logarithmic time. Although it’s more complex than previous methods, it is extraordinarily efficient for large values of `n`.
Conclusion and Next Steps
In this follow-up post, we have delved deeper into optimizing the Fibonacci sequence using Go, explored various methods from iterative approaches to advanced matrix exponentiation techniques. We have enhanced both time and space complexities, making our solution as efficient as possible.
If you find yourself excited by these optimizations, the good news is there’s even more to discover! One topic worth exploring next is memoization in other algorithmic challenges like shortest paths, knapsack problems, and more.
Remember, as programmers, our motto is to “optimize till you drop!” Except, in this case, make sure you don’t drop your laptop. It might not be a bug; it’s a feature – of gravity!
Stay tuned for our next post, where we will unravel more complex algorithms and how to efficiently implement them in Go. Happy coding!
For more Go-related articles and tips, visit the official Go documentation.