Object-Oriented Programming in Python: Mastering Modeling Real-World Entities
Hello, tech enthusiasts! Are you ready to dive deep into the world of Python and unlock the magic of Object-Oriented Programming (OOP)? Today, we are going to explore how Python’s OOP capabilities allow us to model real-world entities in a truly intuitive and dynamic way. So, put on your coding hats and let’s get started!
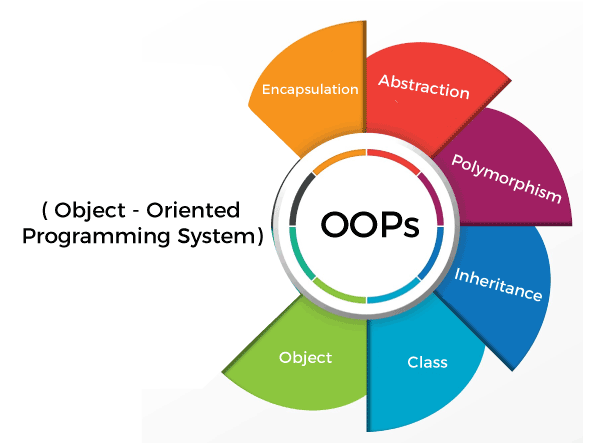
Understanding the Basics of Object-Oriented Programming
Object-Oriented Programming is a paradigm that uses “objects” to design software. These objects are instances of classes, which can be thought of as blueprints for creating entities. Each object can contain both data (attributes) and functions (methods) that describe the behaviors they can perform.
Benefits of OOP
Why should we bother with OOP? Here are some compelling reasons:
- Modularity: Divide your code into clean, manageable sections.
- Reusability: Create reusable code through inheritance and polymorphism.
- Scalability: Build complex, scalable applications with ease.
- Maintainability: Easier to maintain and update your codebase.
Creating Classes and Objects in Python
Let’s start by defining a simple class. Imagine we want to model a real-world entity, like a car. Here’s how we can do it:
class Car:
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
def display_info(self):
return f"{self.year} {self.make} {self.model}"
Here’s what’s happening:
- Class Definition: We define a class named
Car
. - Initializer Method: The
__init__
method initializes the object’s attributes:make
,model
, andyear
. - Method Definition: The
display_info
method provides a way to display the car’s information.
Next, let’s create an instance of this class:
# Creating an instance of the Car class
my_car = Car(make="Tesla", model="Model S", year=2022)
# Using the display_info method
print(my_car.display_info())
This will output:
2022 Tesla Model S
Inheritance: Enhancing Your Classes
One of the wonders of OOP is inheritance, which allows a new class to inherit attributes and methods from an existing class. Let’s say we also want to model electric cars. We can create a subclass ElectricCar
that inherits from Car
.
class ElectricCar(Car):
def __init__(self, make, model, year, battery_size):
super().__init__(make, model, year)
self.battery_size = battery_size
def display_info(self):
return f"{self.year} {self.make} {self.model}, Battery Size: {self.battery_size} kWh"
In this example:
- Subclass Definition: We define
ElectricCar
as a subclass ofCar
using the syntaxclass ElectricCar(Car)
. - Superclass Initialization: The
super()
function is used to call the initializer of the superclassCar
. - Overriding Methods: The
display_info
method is overridden to provide additional information about the battery size.
Now, let’s create an instance of ElectricCar
:
# Creating an instance of the ElectricCar class
my_electric_car = ElectricCar(make="Tesla", model="Model S", year=2022, battery_size=100)
# Using the display_info method
print(my_electric_car.display_info())
This will output:
2022 Tesla Model S, Battery Size: 100 kWh
Encapsulation: Hiding Data
Encapsulation is a principle that aims to restrict direct access to some of an object’s components, which can prevent the accidental modification of data. We achieve this using private attributes or methods designated by a leading underscore (_).
class Car:
def __init__(self, make, model, year):
self._make = make
self._model = model
self._year = year
def display_info(self):
return f"{self._year} {self._make} {self._model}"
Here, the attributes _make
, _model
, and _year
are intended to be private, signaling that they should not be modified directly.
Polymorphism: Flexibility at Its Best
Polymorphism allows us to define methods in a child class that have the same name as methods in the parent class. Let’s implement this with our Car
and ElectricCar
classes.
class Car:
def fuel(self):
return "Refueling with gasoline"
class ElectricCar(Car):
def fuel(self):
return "Charging the battery"
Both classes have a method named fuel
, but their implementations differ. This is the essence of polymorphism!
Real-World Application Example
Let’s see how all these concepts come together in a real-world example. Suppose you’re developing a software solution for a car rental service. Here’s how you might set this up:
class CarRental:
def __init__(self):
self.cars = []
def add_car(self, car):
self.cars.append(car)
def show_inventory(self):
for car in self.cars:
print(car.display_info())
# Creating instances
my_car = Car(make="Toyota", model="Corolla", year=2020)
my_electric_car = ElectricCar(make="Nissan", model="Leaf", year=2021, battery_size=40)
# Using CarRental
rental_service = CarRental()
rental_service.add_car(my_car)
rental_service.add_car(my_electric_car)
rental_service.show_inventory()
Running the code will output:
2020 Toyota Corolla
2021 Nissan Leaf, Battery Size: 40 kWh
And there you have it! This simple yet powerful demonstration illustrates how OOP can model real-world entities and make your code more modular, reusable, and maintainable.
Conclusion
Embracing Object-Oriented Programming in Python opens up a whole new way of thinking about code. By modeling real-world entities through classes and objects, you bring clarity and efficiency to your development process. From inheritance and polymorphism to encapsulation, OOP is packed with features that can supercharge your applications.
Ready to harness the power of OOP in Python? Whether you’re a beginner looking to understand the basics or an advanced developer aiming to refine your skills, continuing to explore and practice these concepts is the key to mastering them. Happy coding!
For more detailed insights into Python’s OOP capabilities, you can visit the official Python documentation.
Until next time, stay curious and keep innovating!