Deep Dive into Control Structures in GoLang
In our previous posts, we explored the basics of control structures in GoLang, covering the essentials of if-else, for loops, and switch statements. If you missed them, check out the [first part of the series](https://sesamedisk.com/mastering-control-structures-in-golang-guide-to-directing-program-flow/). Today, we’re diving deeper into more advanced control structures that will help you master GoLang and write clean, efficient, and readable code.
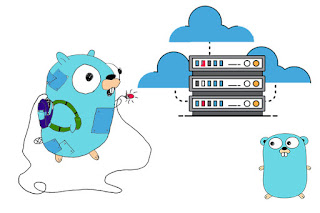
Exploring Nested Control Structures
Nested control structures are a staple in programming, providing a way to create more complex decision-making logic. In GoLang, you can nest if statements within loops, loops within switch statements, and so on. Here’s an example:
Nested Loops
package main
import "fmt"
func main() {
for i := 1; i <= 3; i++ {
for j := 1; j <= 3; j++ {
fmt.Printf("i = %d, j = %d\n", i, j)
}
}
}
In this example, the outer loop controls the variable i, while the inner loop controls the variable j. The loop will print every combination of i and j from 1 to 3.
Switch Within a Loop
package main
import "fmt"
func main() {
for i := 1; i <= 5; i++ {
switch i {
case 1, 2:
fmt.Println("Small number:", i)
case 3, 4:
fmt.Println("Medium number:", i)
case 5:
fmt.Println("Large number:", i)
}
}
}
Here, the for loop iterates through numbers 1 to 5 and the switch statement categorizes each number as small, medium, or large.
Using the `break` Statement Effectively
The break statement in GoLang is used to immediately exit a loop or switch structure. This can be useful for terminating loops early or optimizing the search within data structures.
Breaking Out of Loops
package main
import "fmt"
func main() {
for i := 1; i <= 10; i++ {
if i == 6 {
fmt.Println("Breaking out of loop at i =", i)
break
}
fmt.Println(i)
}
}
In the example above, the loop will terminate when the variable i equals 6, effectively breaking out of the loop.
Leveraging the `continue` Statement
The continue statement skips the current iteration of the loop and proceeds with the next iteration. This is particularly handy for bypassing certain conditions within a loop.
Skipping Even Numbers
package main
import "fmt"
func main() {
for i := 1; i <= 10; i++ {
if i%2 == 0 {
continue
}
fmt.Println(i)
}
}
Here, the continue statement skips even numbers, thus only printing odd numbers from 1 to 10.
Utilizing the `goto` Statement
The goto statement provides a way to jump to another line in your code. While it’s often best to avoid goto for the sake of readability, there are some specific cases where it might be useful.
Simple `goto` Example
package main
import "fmt"
func main() {
fmt.Println("This is the start.")
goto skip
fmt.Println("This code will be skipped.")
skip:
fmt.Println("This code will run.")
}
This example illustrates how goto can skip over certain lines of code to execute others.
Conclusion
By mastering these control structures in GoLang, you’re well on your way to becoming a proficient GoLang developer. Understanding how and when to use nested control structures, as well as effectively leveraging break, continue, and goto statements, can significantly improve your code’s performance and readability.
Stay tuned for our next post where we will explore GoLang’s error handling and how it compares to other languages. As always, remember to practice and experiment with these examples. The key to mastery is persistence!
Don’t forget to share your thoughts and questions in the comments below. Happy coding!