Exploring Advanced Data Types in Python
Welcome back, Python enthusiasts! In our previous post, we laid the groundwork by diving deep into some basic data types in Python. Today, we’re going to expand on that knowledge and explore more complex data types. Buckle up for an adventurous journey into the world of tuples, lists, dictionaries, and sets!
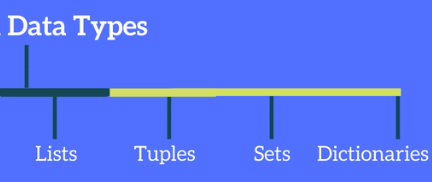
Tuples: Immutable Collections
A tuple is an ordered, immutable collection of items. Think of it as a list that you cannot modify after creation. This makes tuples particularly useful when you want to ensure that a sequence of values remains constant throughout the execution of your program.
my_tuple = (1, 2, 3, 'hello', 5.7)
Once created, you cannot change the values inside the tuple:
my_tuple[0] = 9 # This will raise a TypeError
Why Use Tuples?
Tuples come in handy when you need a collection of items that should not change. They also allow for efficient memory usage. Plus, you can use them as keys in dictionaries, unlike lists!
Lists: Versatile and Mutable
Lists are the Swiss Army knife of Python data types. They are ordered, mutable collections of items, which means you can modify their contents at will.
my_list = [1, 'hello', 3.14, True]
You can add, remove, or change items:
my_list[1] = 'world'
print(my_list) # Output: [1, 'world', 3.14, True]
my_list.append(42)
print(my_list) # Output: [1, 'world', 3.14, True, 42]
Why Use Lists?
Lists are perfect when you need a dynamic collection of items. They are particularly useful for tasks such as iterating over a sequence, modifying elements, or even sorting and filtering.
Dictionaries: Key-Value Pairs
Dictionaries are Python’s implementation of hash tables, and they store data in key-value pairs. Dictionaries are unordered, mutable, and indexed by keys.
my_dict = {'name': 'Alice', 'age': 25, 'location': 'Wonderland'}
You can easily add or modify entries:
my_dict['age'] = 26
my_dict['occupation'] = 'Adventurer'
You can even nest dictionaries within other dictionaries, which can be incredibly useful for complex data representations.
Why Use Dictionaries?
Dictionaries shine when you need a logical association between a key and a value. They are excellent for tasks like database management, querying user information, and storing configurations.
Sets: Unordered Collections of Unique Items
Sets are unordered collections that store unique elements. They are mutable, but every element in a set must be unique.
my_set = {1, 2, 3, 4, 5}
my_set.add(6)
Trying to add an element that already exists in the set has no effect:
my_set.add(3) # No change, 3 is already in the set
Why Use Sets?
Sets are fantastic when you need a collection of unique items. They allow for efficient membership testing and operations like union, intersection, and difference.
Conclusion
And there you have it! We’ve covered the fundamental advanced data types in Python: tuples, lists, dictionaries, and sets. Each of these types offers unique features and advantages, making Python a versatile language for various programming needs.
✨ Stay tuned for more deep dives into Python in upcoming posts, where we’ll explore functions, classes, and more. Keep coding, stay curious, and never stop learning! ?