Continuing the Journey: Advanced Memory Management Techniques in Python
Welcome back, readers! In our previous posts, we delved into Python’s memory allocation and the magic of garbage collection. If you missed those, check out our introductory guide here. Today, we’re strapping on our boots and trekking deeper into advanced techniques to keep our Python programs both memory-efficient and high-performing.
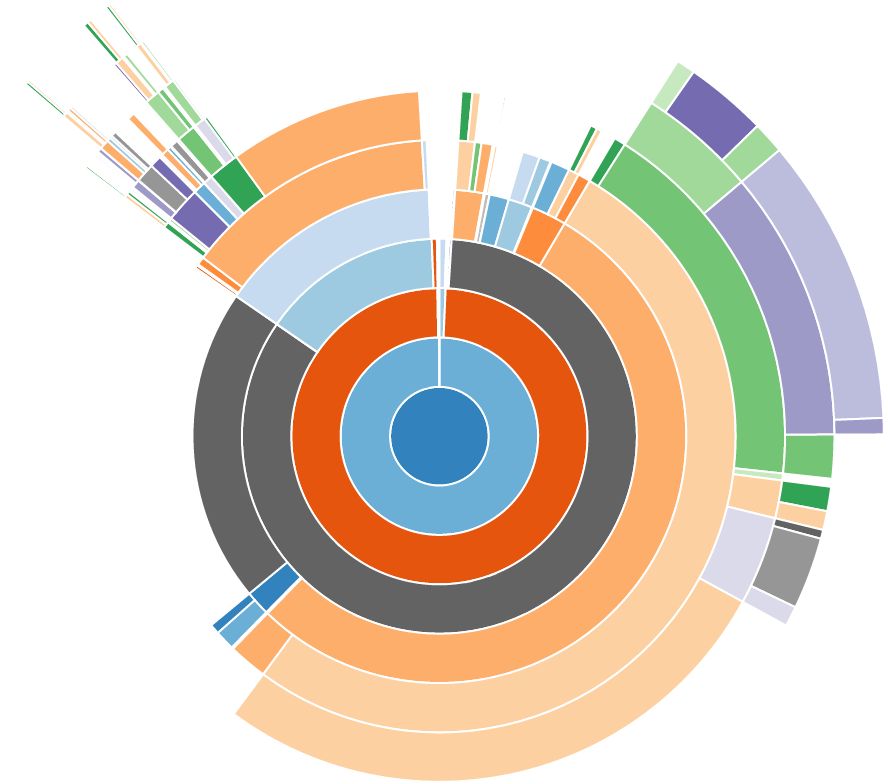
Understanding Python’s Memory Allocation Internals
Now that we know Python automates memory allocation and deallocation, it’s prudent to understand the mechanisms behind the scenes. Did you know that Python uses multiple layers of memory management? Let’s demystify this.
Python divides memory management into the following layers:
- Object-specific allocators (e.g., integers, strings)
- General-purpose allocators (e.g., pymalloc for small objects)
- System allocators (e.g., malloc from C)
Let’s illustrate how Python handles these processes:
# Allocate memory for a simple list
numbers = [1, 2, 3, 4]
In the code above, Python’s object-specific allocator first assigns memory for the list object. The pymalloc allocator handles the memory for the list’s elements. Finally, if larger allocations are needed, Python calls the system allocator.
Memory Profiling and Debugging
But what if you want to understand how much memory an object consumes or identify memory leaks? Python has some useful tools. Let’s explore memory profilers and debuggers.
Using the sys
Module
The sys
module provides insights into the memory usage of Python objects. Here’s how you can analyze an object’s size:
import sys
numbers = [1, 2, 3, 4]
print(sys.getsizeof(numbers)) # Outputs the memory size of the list object
This will give you an idea of how much memory the list is consuming without its elements. Each element will also have its own memory footprint.
Memory Profiling with memory_profiler
If you need a more comprehensive memory analysis, the memory_profiler
package is your go-to tool. You can install it via pip:
pip install memory_profiler
After installation, here’s how you can profile a function:
from memory_profiler import profile
@profile
def my_func():
numbers = [i for i in range(10000)]
return numbers
my_func()
When you run this script, you’ll get a detailed report showing memory usage line-by-line within the function. It’s an indispensable tool for identifying memory-intensive sections of your code. You can refer to the official documentation here.
Preventing Memory Leaks
So, you’ve made it this far and you’re wondering, “How do I prevent memory leaks in Python?” Well, let’s look at some common causes and solutions.
Unintentional Global Variables
Global variables can remain in memory for the lifetime of the program. A best practice is to keep variables’ scopes limited. Check this out:
# Not recommended
global_list = []
def add_elements():
global global_list
for i in range(1000):
global_list.append(i)
add_elements()
A better approach:
# Recommended
def add_elements():
local_list = []
for i in range(1000):
local_list.append(i)
return local_list
numbers = add_elements()
This keeps our memory footprint minimal and easier to manage.
Circular References
Circular references can lead to memory leaks as the reference count never drops to zero. Our trusty garbage collector has cyclic garbage collection to handle these, but it’s always best to avoid such scenarios:
# Circular reference example
class Node:
def __init__(self, value):
self.value = value
self.ref = None
node1 = Node(1)
node2 = Node(2)
node1.ref = node2
node2.ref = node1 # Circular reference
To avoid, one approach is using weak references:
import weakref
class Node:
def __init__(self, value):
self.value = value
self.ref = None
node1 = Node(1)
node2 = Node(2)
node1.ref = weakref.ref(node2)
node2.ref = weakref.ref(node1)
Advanced Garbage Collection Controls
If you like having more control over the garbage collector, Python allows you to fine-tune its parameters:
import gc
# Configure garbage collector thresholds
gc.set_threshold(700, 10, 10)
By manipulating these thresholds, you can control how often garbage collection is triggered. This can be useful for optimizing performance in memory-intensive applications.
Conclusion: Becoming a Memory Management Maestro
Understanding Python’s memory management, from basic allocation and deallocation to advanced profiling and manual management, equips you with the tools to write efficient, high-performing programs. By leveraging Python’s built-in mechanisms like garbage collection and utilizing advanced tools such as memory profilers, you can fine-tune the memory usage of your applications down to the byte.
As we wrap up, remember that while Python adeptly manages memory for you, a deeper understanding enables you to write cleaner, more efficient code. And isn’t that why we persist through debugging and code reviews? To make Pythonic art? If you have further queries or jokes about Python memory managers, feel free to leave a comment or refer to the Official Python Documentation for more deep dives.
Happy coding, and may your memory be ever in your favor!