Mind the Security
Protect Against Common Vulnerabilities
No matter how sleek or sophisticated your SPA looks, if it’s got more vulnerabilities than a straw house in a hurricane, you’re in trouble.
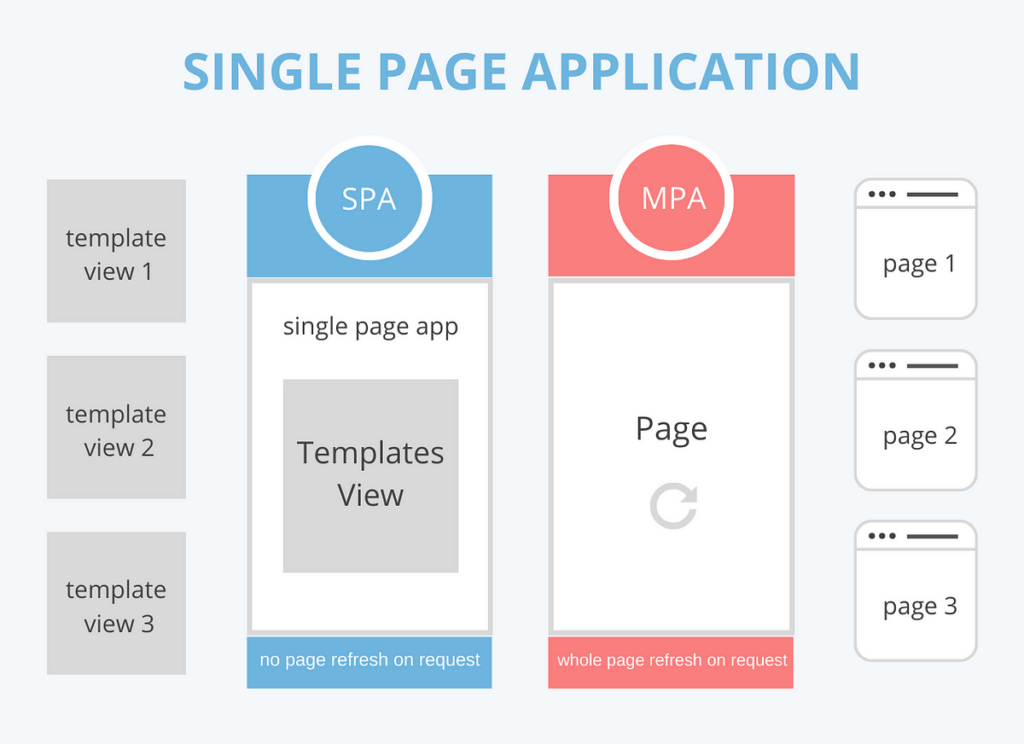
Always ensure your application is safe from common web vulnerabilities such as:
- Cross-Site Scripting (XSS)
- Cross-Site Request Forgery (CSRF)
- SQL Injection (yes, it still exists)
Use libraries and frameworks that help mitigate these risks, and never trust user input unless you’ve verified it. Tools like npm packages validator
and helmet
can be your best friends.
Here’s a basic example of setting up Helmet in a Node.js application:
// Example of using Helmet for basic security
const express = require('express');
const helmet = require('helmet');
const app = express();
app.use(helmet());
// Your app routes here
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
Use HTTPS
If you’re serving your SPA over HTTP, it’s like locking your door but leaving the window wide open. Always use HTTPS to protect data in transit. Most hosting services provide easy ways to get SSL certificates. Let’s Encrypt is a popular choice for free SSL certificates.
Ensure Accessibility
Follow Web Content Accessibility Guidelines (WCAG)
Building accessible SPAs is not just a nice-to-have; it’s a must-have. Follow the Web Content Accessibility Guidelines (WCAG) to make your application usable for everyone, including people with disabilities.
- Use semantic HTML
- Ensure that all interactive elements are keyboard accessible
- Provide text alternatives for non-text content
Remember: Good accessibility practices are like good jokes; everyone should get them!
Test Accessibility
Testing your web application’s accessibility is as crucial as testing its functionality. Use tools like Axe or WAVE to validate the accessibility of your SPAs.
Set Up a Robust Testing Environment
Write Unit Tests
It’s important to test your components individually to ensure they perform as expected. Tools like Jest and Enzyme for React or Jasmine for Angular can help you write unit tests efficiently.
// Example of a simple unit test using Jest in React
import React from 'react';
import { render } from '@testing-library/react';
import App from './App';
test('renders learn react link', () => {
const { getByText } = render(<App />);
const linkElement = getByText(/learn react/i);
expect(linkElement).toBeInTheDocument();
});
Joke: Why don’t developers test their own code? Because they think it works!
Write Integration and End-to-End Tests
Testing individual components is great, but ensuring they all work together is just as crucial. Tools like Cypress and Selenium can help automate integration and end-to-end (E2E) tests.
// Example of an E2E test using Cypress
describe('My First Test', function() {
it('Visits the Kitchen Sink', function() {
cy.visit('https://example.cypress.io') // Change URL as needed
cy.contains('type').click()
cy.url().should('include', '/commands/actions')
cy.get('.action-email').type('[email protected]').should('have.value', '[email protected]')
})
})
These tests help ensure that your entire application workflow works as intended.
Monitor and Maintain
Set Up Monitoring and Logging
Your job isn’t over once your SPA is live. Use monitoring tools like Google Analytics, New Relic, or Sentry to track performance, user interactions, and errors. This data is invaluable for continuous improvement and troubleshooting.
// Example of integrating Sentry with React
import * as Sentry from '@sentry/react';
import { Integrations } from '@sentry/tracing';
Sentry.init({
dsn: 'https://[email protected]/your-dsn',
integrations: [new Integrations.BrowserTracing()],
tracesSampleRate: 1.0,
});
Continuously Update Dependencies
Always keep your dependencies up-to-date to ensure you benefit from the latest features, improvements, and security patches. Tools like npm-check-updates
can be very useful.
// How to use npm-check-updates
npx npm-check-updates -u
npm install
However, be cautious with updates and always test thoroughly before deploying.
Conclusion
Building scalable and maintainable Single-Page Applications (SPAs) is not a walk in the park, but it’s definitely a rewarding journey. By adhering to best practices, staying updated with current trends, and continually optimizing and testing your application, you create a robust and user-friendly product that stands the test of time.
For more advanced tips and tricks, please refer to our previous posts. Keep coding, keep smiling, and happy developing!
Joke: Why do programmers prefer dark mode? Because light attracts bugs!
Until next time!