Diving Deeper: Binary Search Trees
If you remember from our previous discussions, a Binary Search Tree (BST) is designed in a hierarchical structure, which consists of nodes, each having at most two children. We delved into the essence of BSTs earlier (you can check out the detailed post here), but let’s now take a closer look at some advanced concepts and common operations.
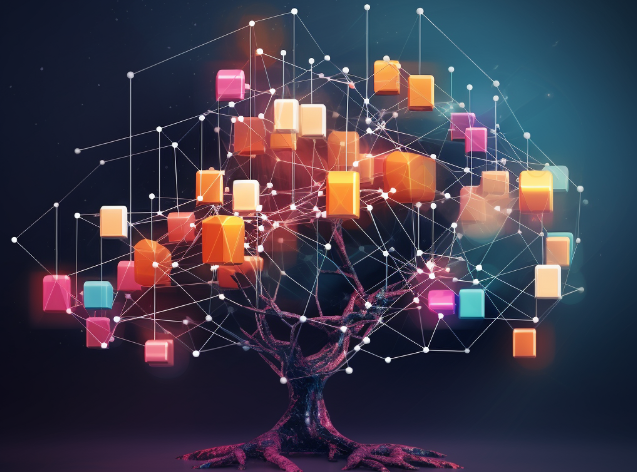
Advanced Concepts in BSTs
One of the key attributes of BSTs that makes them powerful is their ability to maintain their properties through various insertions and deletions. This characteristic ensures the tree remains balanced and efficient for search operations.
Balancing the BST
To prevent a BST from degenerating into a linear structure (which would be akin to a linked list), various balancing techniques are employed, such as AVL Trees and Red-Black Trees. These self-balancing BSTs ensure that the tree’s height remains logarithmic relative to the number of nodes, promoting efficient operations.
class TreeNode:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
class AVLTree:
# AVL Tree specific methods go here
Operations on BSTs
BSTs support several operations including insertion, deletion, and traversal. Let’s quickly summarize these:
- Insertion: Inserting a node involves comparing the new value with the current nodes and placing it appropriately, ensuring the BST property is maintained.
- Deletion: Removing a node can be complex. It involves three cases: deleting a leaf node, deleting a node with one child, and deleting a node with two children.
- Traversal: In-order, pre-order, and post-order traversals are the common methods for BSTs, each serving different use cases.
def inorder_traversal(root):
if root:
inorder_traversal(root.left)
print(root.value)
inorder_traversal(root.right)
Exploring Hash Tables
While BSTs offer an ordered structure, Hash Tables provide a different approach to store and retrieve data efficiently. We had an initial overview of Hash Tables in our previous post, but let’s delve deeper into some advanced features and operations.
Understanding the Hashing Function
A core component of Hash Tables is the hashing function, which transforms a given key into an index in the hash table. A good hashing function needs to be quick and should uniformly distribute keys to minimize collisions.
Collision Handling
Despite the best hashing function, collisions—where two keys hash to the same index—are unavoidable. Collisions are typically handled using techniques like chaining and open addressing:
- Chaining: This involves maintaining a list of all elements that hash to the same index, hence implementing a linked list at each array index.
- Open Addressing: This technique finds another empty slot when a collision occurs, typically using methods like linear probing, quadratic probing, or double hashing.
class HashTable:
def __init__(self):
self.table = [None] * 10 # a hash table of size 10
def hash_function(self, key):
return key % len(self.table)
def insert(self, key, value):
index = self.hash_function(key)
if not self.table[index]:
self.table[index] = [(key, value)]
else:
self.table[index].append((key, value))
Performance Considerations
Hash Tables have an average time complexity of O(1) for search, insert, and delete operations. However, this efficiency is highly dependent on maintaining a low load factor, which is the ratio of the number of elements to the number of slots in the table. High load factors can degrade performance, making it essential to resize the hash table appropriately.
hash_table = HashTable()
hash_table.insert(10, 'value1')
hash_table.insert(20, 'value2')
print(hash_table.table)
When to Use BSTs vs. Hash Tables
Choosing between a BST and a Hash Table depends on your specific use case and requirements:
- Use BSTs When:
- You need ordered data.
- You require a balanced tree structure to ensure efficient search, insert, and delete operations.
- You prefer hierarchical data representations.
- Use Hash Tables When:
- Unordered data is sufficient.
- Fast access times are critical, and average O(1) operations are desired.
- Memory is not a major constraint, allowing for spare space in the hash table.
Wrapping Up and Looking Ahead
Both Binary Search Trees and Hash Tables are indispensable data structures in Python, each with its strengths. BSTs are fantastic for situations where ordered data and balanced search times are essential. On the other hand, Hash Tables provide rapid access to data with average constant time complexity, making them perfect for applications needing quick lookups.
Please read more about the subject in our previous posts. You can also look here for a nice comparison of Binary Search Trees vs. Hash Tables.
Don’t forget to dive further into our previous posts on this topic if you haven’t already. There’s always more to explore, and we’ll continue bringing you the latest insights and deeper dives into the fascinating world of data structures and beyond. Keep coding, keep exploring, and stay cheerful!