Blockchain technology has gained increasing popularity and adoption over recent years. This new distributed database technology offers much potential, especially within financial services, supply chain management, healthcare, etc. Hence, this post will discuss how to get started as a blockchain developer with C#.
Developers are starting to take notice and explore its possibilities. However, understanding what blockchain is and how it works, along with being able to code using C#, remains a challenge for many.
In addition to secure transactions via decentralization, blockchains offer speed, transparency, and immutability. The emergence of smart contracts built upon blockchains is further enhancing their capabilities.
If you’re a C# developer that wants to get started with blockchain, continue reading the article, and we will help you through the journey.
Blockchain Developer vs. Blockchain Engineer
First, you need to know the difference between a blockchain developer and a blockchain engineer. A blockchain developer creates apps on top of a blockchain platform for end-users. In contrast, a blockchain engineer builds the underlying infrastructure, the genesis block, the hash function, chain of blocks, peer network, etc.
The blockchain developer focuses on application development such as building dApps (decentralized apps), managing wallets, smart contract development, interacting with APIs, and so forth.
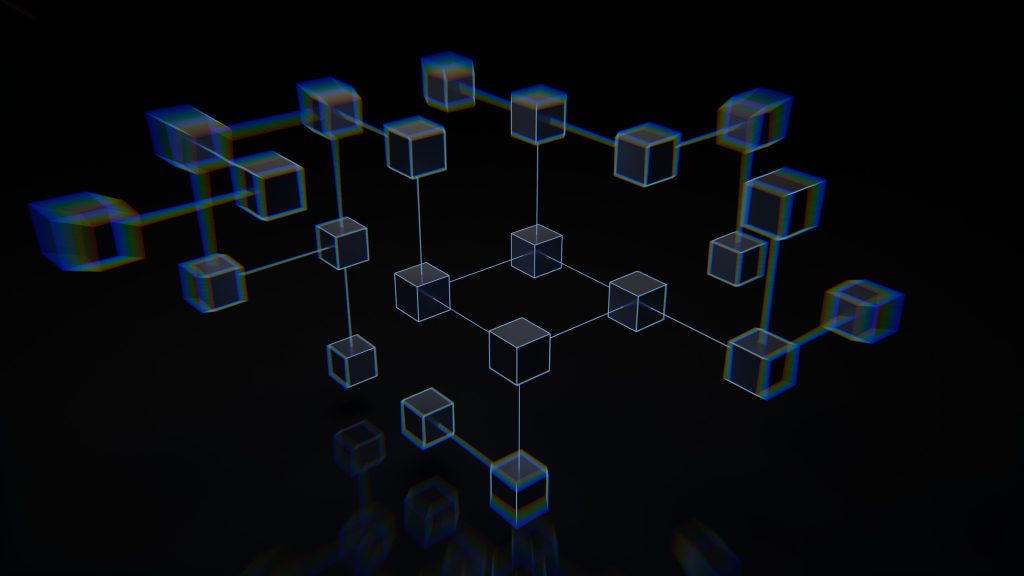
A blockchain developer is among the most highly paid jobs in the software industry. The average annual salary for an experienced blockchain engineer is anywhere from $150,000 to $175,000+. Hence, the demand has risen in recent years, and it is a lucrative career choice.
A blockchain engineer develops the core technologies required by the blockchain network, including consensus mechanisms, cryptography, transaction validation, previous block calculations, private and public blockchains, central authority, source code, and previous hashes.
The tools/resources you need to know about to succeed in this field include:
- Solidity – an Ethereum-based programming language or EVM-compatible (Read our post about https://sesamedisk.com/write-first-smart-contract-in-solidity-blockchain-web/)
- Cryptography
- Distributed systems
- Networking
- Programming languages like C#, Python, and Javascript
How To Get Started As A Blockchain Developer?
To start out, you’ll need a good understanding of computer science fundamentals. It would be best to have at least some experience developing software before getting into blockchain development.
Building a blockchain from scratch is not hard; you need to know how to use programs like VS Code, public-key cryptography, etc. You’ll also need to learn about cryptocurrencies and blockchain development technology. Plenty of online resources can help you learn.
Once you’ve got your basics down, you can start being a blockchain developer yourself. But it does not stop there. You’ll also want to focus on learning one or more of the following:
Why Choose C#?
You might be wondering why I chose C# (C Sharp) for this tutorial. Well, mostly because this is the language I am most proficient in, and also because it is a highly versatile and efficient language. It offers an easy learning curve and includes the object oriented principles necessary for a modern language.
How to Become a Self-Taught Blockchain Developer
There are various ways to become a self-taught blockchain developer. Some people prefer to go straight to the source and learn from scratch. Others choose to read blogs or watch videos.
However, if you don’t want to spend money on courses or books, you can still learn from other developers who already know the concepts. This is an underrated but effective way to learn.
Once you start learning about blockchain development, you need to apply yourself and dedicate time to improving your skills. Becoming proficient in any new skill set takes time. However, once you master the basics, you will be able to build anything.
In summary, here are some tips for how to get started as a blockchain developer:
- Learn about the basics of computer science.
- Read up on cryptocurrency and blockchain technology.
- Find a project to work on.
- Build something cool!
- Share what you learned with others.
- Keep learning!
The Best Blockchain Language and Libraries to Learn in 2022
Several popular programming languages and libraries are used to develop decentralized applications (dApps). Some of these include:
- Solidity – Ethereum-specific programming language designed specifically for writing smart contracts.
- C# – Microsoft’s general-purpose programming language.
- Python – Interpreted scripting language with dynamic typing. Python is extremely useful and versatile.
- Go – General-purpose language developed by Google.
- Rust – General-purpose language from Mozilla.
- Javascript – Versatile language which is useful for off-chain programming and for developing dApps
How To Start As A Blockchain Developer
Start by reading up on the different types of blockchains and cryptocurrencies available. This will give you a better idea of what you’re working towards. There are countless online blogs and resources to help you with this.
Next, read through tutorials on using the most common programming languages to create dApp. For example, there are many examples of using Solidity with Remix IDE.
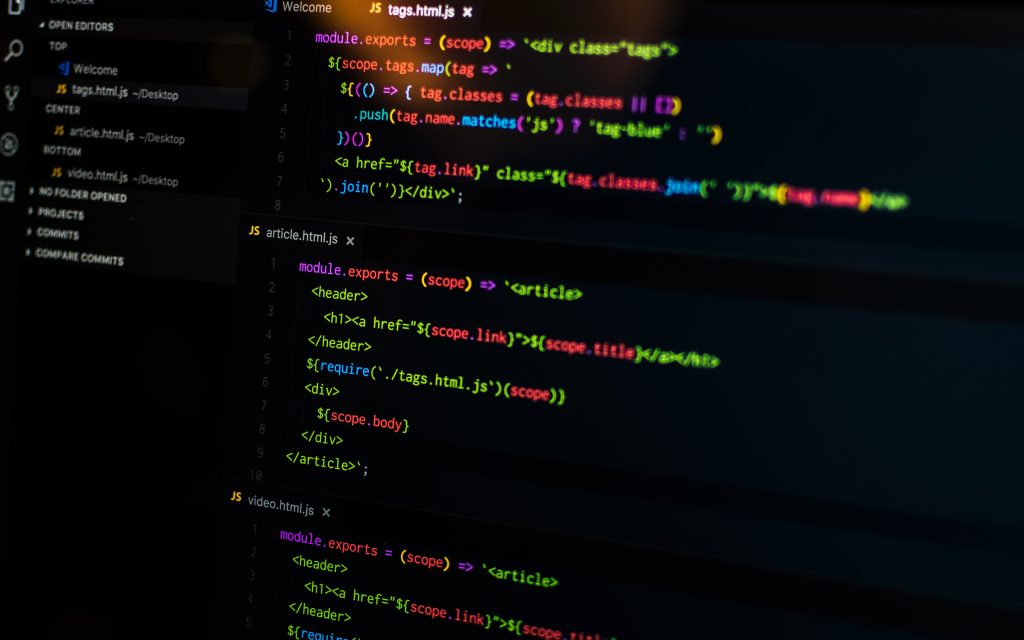
Finally, take a look at the documentation for each of the programming languages. Most of them will be very similar, but they may differ slightly depending on their respective communities.
Blockchain Projects to Work on as a Beginner
It would be best if you considered starting small. You don’t want to dive into too much code or too many projects all at once. Start with a beginner project and slowly expand upon it.
For instance, if you’re interested in creating a dApp, you could start by building a simple website with a built-in chat feature. Then, you could add a few more features such as invoicing, payments, etc.
Once you’ve got your first project under your belt, you’ll understand how things work and know exactly where to go when you need to learn something new.
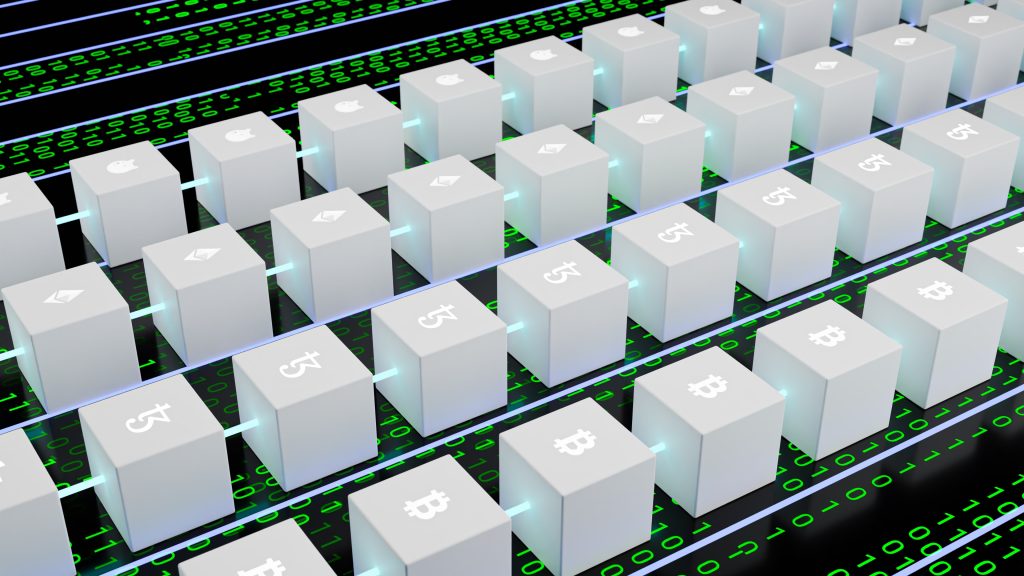
The best way to learn about blockchain technology is to jump right in and start coding. Don’t worry about whether or not you know everything behind the scenes; focus on getting things done. The ideal place to start would be Ethereum because it is the most popular platform for developing dApps.
You can also try implementing some of these ideas in Python since it is a great beginner-friendly language.
What are Smart Contracts?
Smart contracts allow people to interact directly via the internet rather than through intermediaries like banks or other financial institutions. They run autonomously.
What makes smart contracts unique is that they are self-executing programs. They automatically execute certain conditions based on information stored within them. For example, a smart contract might determine whether or not someone has paid their bills. If they haven’t, then the contract will deduct money from their bank account.
The main way to develop smart contracts is by using Solidity. It allows developers to build complex applications quickly. However, it does require a bit of tech knowledge.
How to Build a Blockchain with C# & .NET
To begin, you’ll need to download Visual Studio Code. It is a free code editor for Windows, macOS, Linux, and Chrome OS. (But you can also use another IDE)
Next, install the.NET Core SDK. This is a toolkit that contains all of the necessary tools needed to build.NET Core apps. Then, in the next section, I’ll walk you through setting up a.NET Core Blockchain.
Setting Up Your First Blockchain Project
The first thing we need to take care of is the creation of two classes: The Block and The Blockchain, which are the core of this project.
The Block:
public class Block
{
public int Index { get; set; }
public DateTime TimeStamp { get; set; }
public string PreviousHash { get; set; }
public string Hash { get; set; }
public string Data { get; set; }
public Block(DateTime timeStamp, string previousHash, string data)
{
Index = 0;
TimeStamp = timeStamp;
PreviousHash = previousHash;
Data = data;
Hash = CalculateHash();
}
public string CalculateHash()
{
SHA256 sha256 = SHA256.Create();
byte[] inputBytes = Encoding.ASCII.GetBytes($"{TimeStamp}-{PreviousHash ?? ""}-{Data}");
byte[] outputBytes = sha256.ComputeHash(inputBytes);
return Convert.ToBase64String(outputBytes);
}
}
The Blockchain
public class Blockchain
{
public IList<Block> Chain { set; get; }
public Blockchain()
{
InitializeChain();
AddGenesisBlock();
}
public void InitializeChain()
{
Chain = new List<Block>();
}
public Block CreateGenesisBlock()
{
return new Block(DateTime.Now, null, "{}");
}
public void AddGenesisBlock()
{
Chain.Add(CreateGenesisBlock());
}
public Block GetLatestBlock()
{
return Chain[Chain.Count - 1];
}
public void AddBlock(Block block)
{
Block latestBlock = GetLatestBlock();
block.Index = latestBlock.Index + 1;
block.PreviousHash = latestBlock.Hash;
block.Hash = block.CalculateHash();
Chain.Add(block);
}
}
After that, use the application to create an instance of the blockchain and add blocks to it. You can add the code below; the output serializes into a JSON file.
Blockchain phillyCoin = new Blockchain();
phillyCoin.AddBlock(new Block(DateTime.Now, null, "{sender:Henry,receiver:MaHesh,amount:10}"));
phillyCoin.AddBlock(new Block(DateTime.Now, null, "{sender:MaHesh,receiver:Henry,amount:5}"));
phillyCoin.AddBlock(new Block(DateTime.Now, null, "{sender:Mahesh,receiver:Henry,amount:5}"));
Console.WriteLine(JsonConvert.SerializeObject(phillyCoin, Formatting.Indented));
Blockchain allows you to store sensitive information and prevent unauthorized access. Users cannot alter the data when storing sensitive information on the blockchain without changing the entire ledger.
However, it is just a data structure, and it can easily be changed by this code below:
phillyCoin.Chain[1].Data = "{sender:Henry,receiver:MaHesh,amount:1000}";
That’s why the need to validate data arises, and we can do that with the code below:
public bool IsValid()
{
for (int i = 1; i < Chain.Count; i++)
{
Block currentBlock = Chain[i];
Block previousBlock = Chain[i - 1];
if (currentBlock.Hash != currentBlock.CalculateHash())
{
return false;
}
if (currentBlock.PreviousHash != previousBlock.Hash)
{
return false;
}
}
return true;
}
After adding this code, the function IsValid will check each block’s hash to see if the block has been changed and the previous block’s hash to see if it has been altered or recalculated. Then the function is called once before the data tampering and once to see if any issues arise.
Console.WriteLine($"Is Chain Valid: {phillyCoin.IsValid()}");
Console.WriteLine($"Update amount to 1000");
phillyCoin.Chain[1].Data = "{sender:Henry,receiver:MaHesh,amount:1000}";
Console.WriteLine($"Is Chain Valid: {phillyCoin.IsValid()}");
But what if the hacker recalculates the hash of the tampered block?
It’s simple you just need to add this line of code to prevent it:
phillyCoin.Chain[1].Hash = phillyCoin.Chain[1].CalculateHash();
Okay, but what if the hacker recalculates hashes of the current block and all the following blocks? What to do?
Once again, blockchain developers have thought about this issue and implemented this function to stop it:
Console.WriteLine($"Update the entire chain");
phillyCoin.Chain[2].PreviousHash = phillyCoin.Chain[1].Hash;
phillyCoin.Chain[2].Hash = phillyCoin.Chain[2].CalculateHash();
phillyCoin.Chain[3].PreviousHash = phillyCoin.Chain[2].Hash;
phillyCoin.Chain[3].Hash = phillyCoin.Chain[3].CalculateHash();
After the blocks are recalculated, they’re verified by a consensus algorithm. Each block gets verified on only one node instead of all because blockchain is a decentralized system. If any node changes its vote, others would notice and eventually catch on.
Finding Jobs as a Blockchain Developer
If you’re just starting out, you might not have much work experience yet In this case, you could try freelancing websites like Upwork.
Or, if you already have some experience, you could consider applying to jobs that require blockchain knowledge. It would help if you also kept an eye out for job postings related to blockchain development.
The best way to get started is to start building something! If you don’t know where to begin, you can always build something simple like a basic trading bot or a crypto exchange. Once you’ve built your first project, you’ll likely want to share it with others. That’s when you can start getting paid for your skills.
Having an online portfolio of your work it’s one of the best ways to display your skills, and it’s something that we recommend you do as soon as possible. Even small projects go a long way since they indicate to the potential client that you’re hard-working and knowledgeable.
You can even start posting about your work on social media sites such as Twitter and LinkedIn. These platforms allow you to connect with potential clients and showcase your work.
Conclusion
Blockchain development is one of the hottest career choices in 2022. This should remain true soon with increasing demand, in my opinion. If you decide to pursue a career in blockchain development, keep yourself updated with the latest trends and developments in the industry.
There are many paths to choose from on your journey. Hence, it is essential to do your research. While it may overwhelm you, the best selection can help you. While smart contracts are usually written in Solidity, C#, Python and Javascript are great languages for smart contract interaction and dApps. Similarly, other tools like Brownie help you on your path.
Also, make sure that you learn from others’ mistakes and successes. Don’t hesitate to ask questions whenever you feel confused. This is a great way to improve. While often ignored by new devs, this will be fruitful down the road.
Furthermore, if you liked this post, please let us know in the comments. I put a lot of effort into this one since I love this space. I’d love to hear your feedback and if you learned something helpful from this post. This would encourage me to write more on similar things.
If you’re already a blockchain developer or already learning it, you can check out two of our posts. Write First Smart Contract in Solidity and Smart Contracts in Python: Complete Guide will help you on your learning path.
Lastly, if you have any queries or anecdotes from your experience, please share them below. Good luck on your journey! Have a great week!
Edited by: Syed Umar Bukhari.