Introduction to Content Generation with Python and ChatGPT API
Are you tired of spending hours sifting through long articles or struggling to come up with fresh ideas? Imagine if there was a way to tap into the power of AI to revolutionize how you consume and create content. Say hello to ChatGPT! In this article, we’ll dive into the fascinating world of ChatGPT applications and API access in Python, revealing how you can save time, supercharge your creativity, and enhance your productivity like never before.
Automatic Content Creation with ChatGPT AI
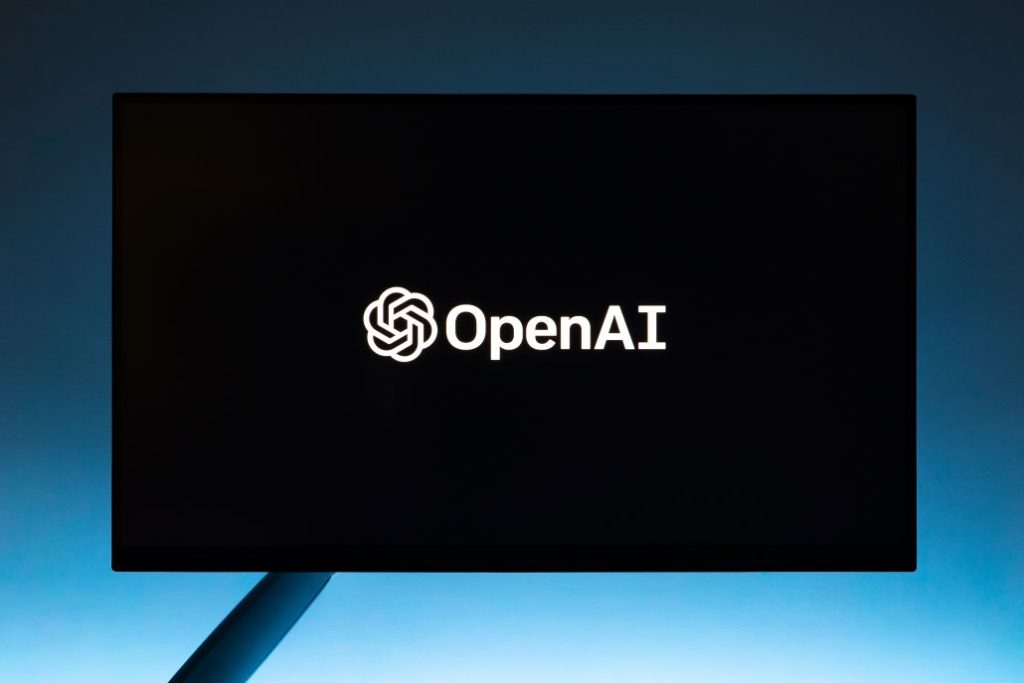
ChatGPT is an excellent tool for automatically producing content. This includes blog posts, articles, product descriptions, job proposals, cover letters, etc. A key benefit of ChatGPT is the time and resources it saves. Developers can input a prompt and receive an instantaneous output. You can produce large chunks of content if you know what you’re doing– in a fraction of the time it took.
Moreover, ChatGPT generates relevant human-like text. As such, ChatGPT is a valuable tool for streamlining content creation due to its versatility. Also, it can boost the work flow in any business.
Prerequisites: Setting up Python and ChatGPT API Access
Now, I will list the prerequisites you need to access the ChatGPT API with Python:
- Python version 3.7 or above: Download and install the latest version of Python.
- Visual Studio Code or PyCharm (or any IDE for Python).
- ChatGPT API: You need to create an account to access it.
- OpenAI’s Python library: Install the required Python library that provides an easy way to interact with the ChatGPT API.
Installing the Necessary Python Libraries for the API
You must install a few vital libraries using Python to work with the ChatGPT API. These libraries will help you interact with the API more efficiently. Besides, it helps to handle various tasks related to content generation. So, here’s how to install these Python libraries:
- Open a terminal or command prompt. (You can do this inside your IDE too)
Then, install the OpenAI library, which offers an easy way to interact with the ChatGPT API.
After that, run this command to install:
pip install openai
Remember to store your API key securely. It’s essential to keep your API key private and avoid hard coding it in your scripts.
Consider installing the “requests” library if you plan to work with additional data formats like JSON. Why? It helps to simplify making HTTP requests. So, use this line to install the library:
pip install requests
Now, let’s move to the next section. This discusses how to connect to the ChatGPT API to gain access.
Connecting to the ChatGPT API using Python: What do you need?
To connect to the ChatGPT API using Python, here is a quick recap to ascertain that you didn’t miss a step.:
- First, ensure you have completed the earlier prerequisites, including installing the above Python libraries.
- Also, a reminder: store your API key securely.
Now, with that out of the way, let’s get started. In your Python script or a terminal, import the required libraries you installed earlier.
import openai
import os
After that, you can connect your OpenAI library using your ChatGPT API key. I’ll discuss more in the next part of this article.
Access your ChatGPT API Key: How to obtain your keys?
At this point, you may be confused. How do I find or gain access to this API key? Don’t worry. To gain access to the ChatGPT API key, you must have signed up on OpenAI. If you haven’t, head over to the OpenAI website and do that now. Once you are on the platform dashboard, you’re nearly there. Then, click on your profile in the top right corner and go to “View API Keys“.
Quick tip, you can go to docs and instantly do this too. Saves a ton of time, am I right?
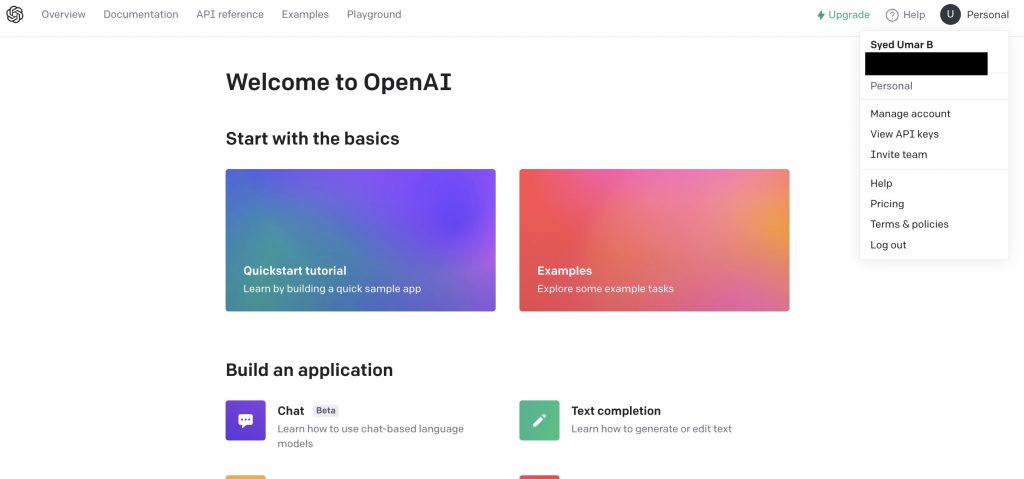
Create a new ChatGPT Secret API Key
After you have done that, click on the “Create new secret key” button. Don’t forget to copy your secret key to somewhere safe and ensure you don’t do it with anyone. As you can see, I have two ChatGPT API keys created already. I do not know if there is a limit on the number of keys you can create. However, from my interaction, I don’t see a limit.
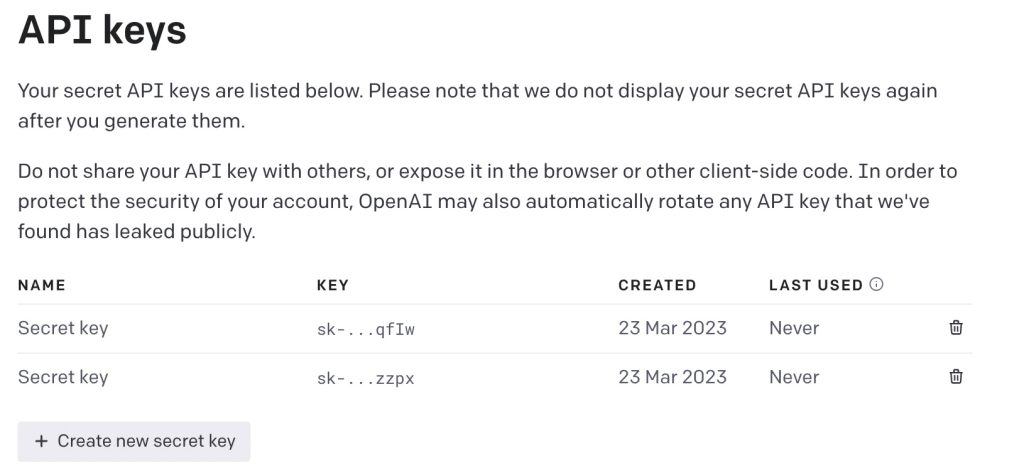
Great. You have your ChatGPT API key now. Can we begin coding to connect the Python app to ChatGPT AI? Well… yes! Just so you know, you are ready to begin now.
Here is you how you can store your API key with OpenAI:
import openai
openai.api_key = "API_Key"
Note: you shouldn’t store your API key like that in production code. Instead, create an environmental variable in the dot env file to store this ChatGPT key. You will also have to install the dotenv library to be able to call the variable with the API key in your code without affecting the security of your project. Also, make sure the .env file is git ignored. Why? You don’t want your API key getting stolen or used illegally.
So, this next section is the juicy part. Let’s dive into it.
Cost of ChatGPT API per Token
Before that, you should know the effect the API has on your bank balance. Using the ChatGPT API can be both affordable and efficient for your projects. The cost for gpt-3.5-turbo is attractively priced at just $0.002 per 1K tokens. On the other hand, for GPT-4 ChatGPT API access, the cost is different. For 8K context, the cost is $0.03 per 1K tokens for prompts and $0.06 per 1K tokens for completions. For a larger 32K context, the price is $0.06 per 1K tokens for prompts and $0.12 per 1K tokens for completions. However, it can scale up quickly if you don’t keep track.
Interacting with ChatGPT using Python: A Beginner’s Guide
Your Python environment is now set up, and you can access the ChatGPT API you created. You’re ready to start building apps using Python and the ChatGPT API.
# Note: you need to be using OpenAI Python v0.27.0 for the code below to work
With the details set up, you can now interact with the ChatGPT API using the OpenAI library. To send a request, use the create() method:
output = openai.ChatCompletion.create(
model="gpt-3.5-turbo",
messages=[{"role": "user", "content":
"Your prompt goes here"}],
max_tokens=150,
n=1,
stop=None,
temperature=0.8,
top_p=1,
)
Replace “Your prompt goes here” with your desired prompt for generating content. Feel free to adjust other parameters, such as max_tokens, temperature, and top_p. Don’t worry if you don’t know what these parameters mean yet. Could you bear with me for a minute?
It might be a good idea to extract the generated text from the response. Let’s see how:
print(output['choices'][0]['message']['content'])
Now you are connected to the ChatGPT API using Python. You can use the OpenAI library to send requests and generate content. Pretty cool, right?
Generating Text with ChatGPT: Key Concepts and Parameters
When working with the ChatGPT API to generate text, it’s critical to understand the main concepts and parameters that affect the output and adjust the generated text.
Let’s discuss some of these key concepts and parameters:
Model: The model parameter specifies the version of the ChatGPT model you want to use. In this case, we use “gpt-3.5-turbo“, a powerful and versatile model. Gpt-3.5-turbo-0301 does not always pay strong attention to system messages.
Messages: The message parameter is a list of message objects. Each contains a role (“user”, “assistant”, or “system”) and content (the actual text input). It provides the prompt to work on. Then, the API uses this input to create the desired content.
Output: The API response is stored in the output variable. Also, you can access the entire response dictionary using print(output). On the contrary, you can extract the generated text using print(output[‘choices’][0][‘message’][‘content’]).
Okay let’s examine some adjustable parameters now. It can help you optimize the content:
Max Tokens: You can control the length of the generated content by passing the max tokens’ parameter to the OpenAI Chat Completion create() method. For example, setting max tokens can limit the response to 100 tokens.
Temperature: You can edit the output text’s creativity and randomness by changing the temperature. A low value means a more deterministic model.
Top-P: The top_p parameter filters out tokens with a cumulative probability lower than the specified value. For example, if = 0.9; the LLM model only considers the 90 most likely words or phrases. A lower top-P value will result in less varied responses.
You can optimize the ChatGPT API’s performance by knowing these key concepts. Hence, this will help you generate content that meets your needs.
Improving ChatGPT AI Prompts for Content Generation
High quality prompts are crucial for getting relevant outputs when using the OpenAI’s API. So, here are some tips for creating great prompts:
Be specific: First, provide clear and concise instructions in your prompt to guide the AI. Then, specify the desired format, tone, and details.
Set context: Start your prompt with a brief context to help the AI better understand the subject.
Use examples: Include examples in your prompt to illustrate the desired output or style.
Iterate and refine: Try different prompts and evaluate the generated text. You can also do this by adjusting the tone, providing more context, or clarifying the instructions.
There will be a whole post on ChatGPT prompt generation soon. So, stay tuned.
Some Interesting ChatGPT Applications with the API access in Python
Text Summarization with ChatGPT
Have you ever wished there was a way to get the gist of a lengthy piece of text in a flash? Here’s what you need! With ChatGPT, you can consume information in a new way powered by AI.
ChatGPT condenses long and complex articles into concise summaries with the incredible power of text summarization. The system will generate an easy to read précis in seconds. Your fingertips will be full of quick, concise, and relevant information. So, no more headaches, no more wasted time.
Besides, ChatGPT is changing the way content is consumed! That said, let’s look at how to code this in Python.
Here is the code snippet in Python text summarization:
def summarize_text(text, model="text-davinci-002"):
prompt = f"Please provide a short summary:\n{text}\nSummary:"
response = openai.Completion.create(engine=model,
prompt=prompt,
max_tokens=50,
n=1,
temperature=0.5,
)
summary = response.choices[0].text.strip()
return summary
# Replace 'your_text_here' with the text you want to summarize
text_to_summarize = "your_text_here"
summary = summarize_text(text_to_summarize)
print("Summary:", summary)
The provided Python script defines a function that takes an input and an optional model name (defaulting to “text-DaVinci-002”) to create a summary using OpenAI’s API.
The function constructs a prompt by appending “Summary:” to the input text, then calls the API with the specified parameters, such as model, prompt, max tokens, n (number of responses), and temperature. Thus, the summary is extracted from the API response, and any whitespace is removed before returning it.
Hence, this script is a convenient way to summarize any text using OpenAI’s language model.
Here’s the long input:
Mindfulness meditation is a practice that involves focusing one's attention on the present moment, without judgment. By cultivating mindfulness, individuals can better manage stress, enhance emotional well-being, and improve overall mental health. Research has shown that regular mindfulness meditation can have lasting positive effects on brain function, including increased focus, memory, and cognitive flexibility
And this is the output seen. Remember, it can be made even more concise according to need.
Summary: Mindfulness meditation is a practice that helps individuals to focus on the present moment without judgment. Research has shown that this practice can have lasting positive effects on brain function, including increased focus, memory, and cognitive flexibility.
Prompt Generating Idea with ChatGPT
Feeling stuck as you face a blank screen — struggling to develop ideas or code snippets? Bid farewell to coding struggles and writer’s block. Why? Because ChatGPT can ease your burdens. With API access, you can energize your projects with creativity!
So, let’s look at a code snippet for integrating OpenAI’s API to create this unique prompt:
def generate_prompt_ideas(category, model="text-davinci-002"):
prompt = f"Generate 5 creative prompt ideas for {category}:\n\n"
response = openai.Completion.create(
engine=model,
prompt=prompt,
max_tokens=150,
n=1,
temperature=0.7,
)
ideas = response.choices[0].text.strip()
return ideas
# Replace 'your_category_here' with the category you want to generate ideas for
category = "your_category_here"
generated_ideas = generate_prompt_ideas(category)
print("Generated Ideas:\n", generated_ideas)
The given Python script defines a function takes input to generate creative ideas for the specified category using the OpenAI ChatGPT API.
This script is valuable for generating creative prompt ideas for any given category using OpenAI’s language model.
Here’s the output, given the input was about travel.
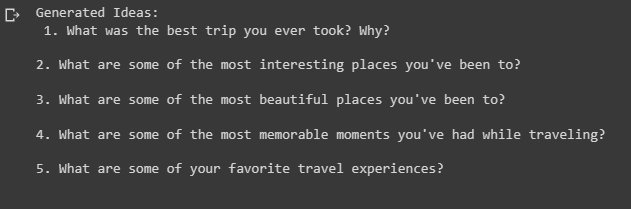
Brainstorming Ideas with ChatGPT
Imagine being in a room full of imaginative individuals, all eager to brainstorm innovative and thrilling concepts. How do you start generating unique and captivating ideas? This is where ChatGPT comes in, functioning as an intelligent assistant that can help you produce ideas on any topic you have in mind.
Let’s look for some codes for integrating Openai’s API in a Python environment to create this unique idea.
def brainstorm_ideas(topic, model="text-davinci-002"):
prompt = f"Brainstorm innovative ideas related to {topic}:\n\nIdeas:"
response = openai.Completion.create(
engine=model,
prompt=prompt,
max_tokens=150,
n=1,
temperature=0.7,
)
ideas = response.choices[0].text.strip()
return ideas
brainstorm_topic = "your_topic_here"
generated_ideas = brainstorm_ideas(brainstorm_topic)
print("Brainstormed Ideas:\n", generated_ideas)
This Python script defines a function to take a topic as input to generate innovative ideas related to the topic using OpenAI’s ChatGPT API.
Hence, this script is a helpful tool for generating innovative ideas on any given topic using OpenAI’s language model.
Here’s the output for a food startup:
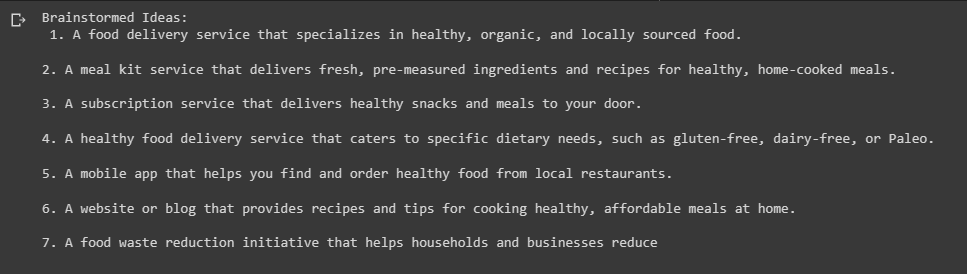
Conclusion: Using the API with Python
In conclusion, you need to sign up for OpenAI to gain access to the ChatGPT API. Using that, there is a unique mix of use cases, such as summarization, prompt generation and brainstorming. Remember to keep your API key secret and not share it with anyone. Furthermore, this post discusses the parameters of the create method as well as some brief ideas to improve prompt quality. But more on that in a future post.
I hope you liked this post. Please let me know what you think and what your experience has been with the API. If you face any issues, let me know too, so I can help.
Similar articles: ChatGPT: The Next Big Thing in AI and ChatGPT AI: Features to 6X Your Productivity in 2023.
Edited by: Syed Umar Bukhari.