Python Data Structures: Binary Search Trees vs. Hash Tables
Introduction
Hello, tech enthusiasts! Today, we’re diving into the fascinating world of Python data structures. More precisely, we’re delving into two powerhouse data structures that you’ll encounter frequently in your coding journey: Binary Search Trees (BSTs) and Hash Tables. By the end of this post, not only will you have a good grasp of both, but you’ll also understand their unique characteristics, strengths, and potential use cases.
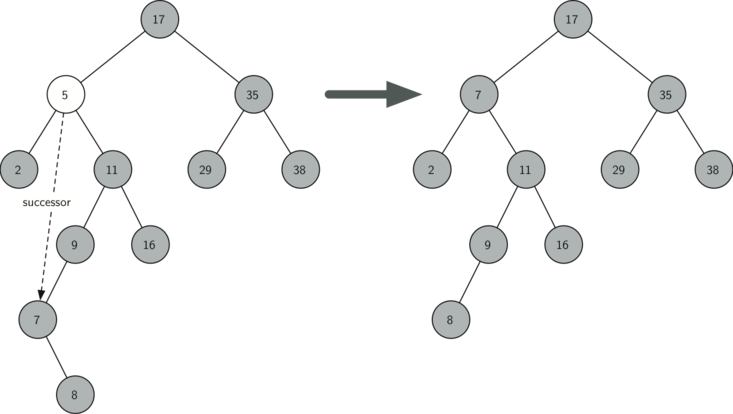
What are Binary Search Trees?
A Binary Search Tree (BST) is a special kind of binary tree that maintains order at all times. The key feature of BSTs is that for any given node, all nodes in its left subtree have values less than the node’s value, and all nodes in its right subtree have values greater than the node’s value. This inherent order makes BSTs an excellent choice for operations like insertion, deletion, and lookup, which can be performed on average in O(log n) time.
Inserting Elements into a BST
Let’s get our hands dirty with some Python code to demonstrate inserting elements into a BST:
class Node:
def __init__(self, key):
self.left = None
self.right = None
self.value = key
class BST:
def __init__(self):
self.root = None
def insert(self, key):
if self.root is None:
self.root = Node(key)
else:
self._insert(self.root, key)
def _insert(self, node, key):
if key < node.value:
if node.left is None:
node.left = Node(key)
else:
self._insert(node.left, key)
else:
if node.right is None:
node.right = Node(key)
else:
self._insert(node.right, key)
# Example Usage
bst = BST()
bst.insert(10)
bst.insert(20)
bst.insert(5)
Advantages and Disadvantages of BSTs
One of the remarkable features of BSTs is their ability to maintain a sorted list of elements, which you can easily iterate over. Additionally, due to their binary nature, they use less memory compared to more complex structures. However, a notable downside is that the performance can degrade to O(n) when the tree becomes unbalanced.
What are Hash Tables?
Hash Tables, on the other hand, offer a different approach. They use a hash function to compute an index into an array of buckets or slots, from which the desired value can be found. This unique mechanism allows operations like insertion, deletion, and lookup, on average, to be performed in constant time, O(1).
Implementing Hash Tables in Python
Here’s how you can create a simple Hash Table in Python:
class HashTable:
def __init__(self):
self.table = [None] * 10
def hash_func(self, key):
return key % len(self.table)
def insert(self, key, value):
hash_key = self.hash_func(key)
if self.table[hash_key] is None:
self.table[hash_key] = []
self.table[hash_key].append(value)
def remove(self, key, value):
hash_key = self.hash_func(key)
if self.table[hash_key] is not None:
try:
self.table[hash_key].remove(value)
except ValueError:
pass
# Example Usage
ht = HashTable()
ht.insert(10, 'val1')
ht.insert(20, 'val2')
ht.insert(15, 'val3')
print(ht.table)
Advantages and Disadvantages of Hash Tables
Hash Tables excel in scenarios where you need constant-time performance for insertions and lookups. They’re indispensable in the creation of associative arrays, databases, and caching systems. However, their performance can be significantly impeded by hash collisions, where multiple keys map to the same index.
Comparison: When to Use Which?
Choosing between a BST and a Hash Table depends on your specific needs. Here are some considerations to help you decide.
Search Efficiency
– BST: Offers O(log n) search time for balanced trees. Useful if you need to maintain a sorted collection.
– Hash Table: Provides O(1) search time but is dependent on the quality of the hash function to prevent collisions.
Memory Usage
– BST: Typically uses less memory but can degrade in performance when unbalanced.
– Hash Table: Requires additional memory for storing keys but offers consistent performance despite the collection size.
Order and Traversal
– BST: If you need an ordered collection you can easily traverse, BSTs are ideal.
– Hash Table: No intrinsic order is maintained, making in order traversing difficult and inefficient.
Conclusion
Both Binary Search Trees and Hash Tables are powerful in their own right, each excelling in different scenarios. By understanding the strengths and trade-offs of each, you can make informed decisions about which data structure to use in your projects. Whether you’re dealing with ordered data or need constant-time complexity, there’s a structure that fits your needs perfectly.
And if you’re looking to delve deeper into data structures, this guide to data structures will be a valuable resource. Happy coding!
So, there you have it! BSTs vs. Hash Tables, both amazing tools in the arsenal of a Python developer. Keep learning, keep coding, and as always, keep that positive energy flowing. Until next time!