String Matching with Python: KMP vs. Boyer-Moore Algorithm
Hey there, fellow tech enthusiasts! If you’ve ever geeked out over algorithms, you’ve probably come across string matching algorithms. They are the backbone of many core functionalities in search engines, text editors, and even DNA sequencing. Today, we’re diving into two powerhouse methods: the Knuth-Morris-Pratt (KMP) and Boyer-Moore algorithms. Ready to sharpen your coding skills? Let’s get started!
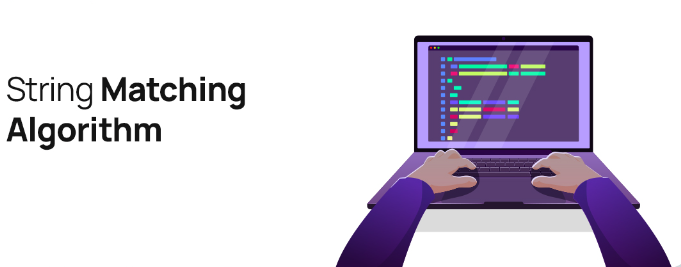
Understanding String Matching
String matching, at its core, is about finding occurrences of a “pattern” string within a “text” string. While it sounds straightforward, efficiency is key. Poor implementations can lead to disastrously slow search operations, turning your program into a snail. That’s why we turn to advanced algorithms like KMP and Boyer-Moore.
The Knuth-Morris-Pratt (KMP) Algorithm
The KMP algorithm, named after its creators, is all about avoiding unnecessary comparisons. It does this using a prefix table to track the longest prefix which is also a suffix. This helps the algorithm “remember” where it left off and skip unnecessary checks.
How KMP Works
def kmp_search(pattern, text):
n = len(text)
m = len(pattern)
lps = [0] * m
j = 0 # index for pattern[]
def compute_lps_array(pattern, m, lps):
length = 0 # length of the previous longest prefix suffix
lps[0] = 0 # lps[0] is always 0
i = 1
while i < m:
if pattern[i] == pattern[length]:
length += 1
lps[i] = length
i += 1
else:
if length != 0:
length = lps[length - 1]
else:
lps[i] = 0
i += 1
compute_lps_array(pattern, m, lps)
i = 0 # index for text[]
while i < n:
if pattern[j] == text[i]:
i += 1
j += 1
if j == m:
print("Found pattern at index " + str(i - j))
j = lps[j - 1]
elif i < n and pattern[j] != text[i]:
if j != 0:
j = lps[j - 1]
else:
i += 1
# Example usage
kmp_search("abc", "abcabcabc")
Notice how the compute_lps_array
function is used to calculate the “longest prefix suffix” array, which helps in minimizing the number of comparisons.
The Boyer-Moore Algorithm
If KMP is about avoiding unnecessary comparisons, Boyer-Moore is all about using the information to skip sections of the text. It’s renowned for its efficiency in practical scenarios.
How Boyer-Moore Works
Boyer-Moore uses two heuristics:
- Bad Character Heuristic: If a mismatch occurs, the position of the last occurrence of the mismatched character in the pattern is used to align the pattern with the text.
- Good Suffix Heuristic: If a mismatch occurs, the pattern is shifted in such a way that the next possible match is attempted.
def boyer_moore(pattern, text):
def bad_char_heuristic(pattern):
bad_char = [-1] * 256
for i in range(len(pattern)):
bad_char[ord(pattern[i])] = i
return bad_char
def good_suffix_heuristic(pattern):
m = len(pattern)
good_suffix = [-1] * (m + 1)
border_pos = [-1] * (m + 1)
i, j = m, m + 1
border_pos[i] = j
while i > 0:
while j <= m and pattern[i - 1] != pattern[j - 1]:
if good_suffix[j] == -1:
good_suffix[j] = j - i
j = border_pos[j]
i -= 1
j -= 1
border_pos[i] = j
j = border_pos[0]
for i in range(m + 1):
if good_suffix[i] == -1:
good_suffix[i] = j
if i == j:
j = border_pos[j]
return good_suffix
bad_char = bad_char_heuristic(pattern)
good_suffix = good_suffix_heuristic(pattern)
s = 0
while s <= len(text) - len(pattern):
j = len(pattern) - 1
while j >= 0 and pattern[j] == text[s + j]:
j -= 1
if j < 0:
print(f"Pattern found at index {s}")
s += good_suffix[0] if good_suffix else 1
else:
s += max(good_suffix[j + 1], j - bad_char[ord(text[s + j])])
# Example usage
boyer_moore("abc", "abcabcabc")
In this implementation, both heuristics are used to determine the next position of the pattern after a mismatch.
Performance Comparison
When it comes to performance, both algorithms have their strengths:
- KMP has a linear complexity of O(n + m), making it efficient in terms of time but requiring additional space for the prefix table.
- Boyer-Moore can perform better in practice, especially with large alphabets, due to its ability to skip larger sections of text—but it might have worse-case time complexity in certain scenarios.
Choosing between these two really depends on the specific use case and the nature of the input data. If you’re dealing with long texts and patterns, and primarily concerned with practical performance, Boyer-Moore might be your best bet. For consistent, predictable performance, KMP can be a solid choice.
Conclusion
And there you have it! Two incredibly efficient and fascinating string matching algorithms—the KMP and Boyer-Moore—each with its elegance and strategic brilliance. Whether you choose the memory-efficient KMP or the skip-savvy Boyer-Moore, understanding these algorithms will significantly enhance your ability to handle text processing tasks more efficiently.
For further reading, check out the comprehensive explanation of KMP and Boyer-Moore on Wikipedia.
Stay curious, keep experimenting, and happy coding! ?