Control Structures in Python: Directing the Program Flow
Hello, wonderful readers! Today, we’re diving deep into the world of Python, exploring the powerful control structures that help direct the program flow. Think of control structures as the GPS of your code, guiding it to execute commands efficiently and effectively. So buckle up, and let’s embark on this delightful journey!
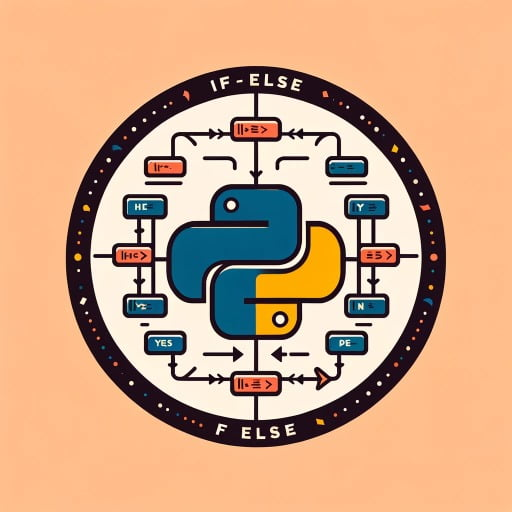
Understanding Control Structures
Control structures in Python are essential for executing code snippets based on certain conditions, repeating tasks, or even branching the flow of execution. They are broadly categorized into three types:
- Conditional Statements
- Loops
- Branching Statements
Conditional Statements
Conditional statements evaluate expressions and handle the program flow based on whether the condition is True or False. The primary conditional statements in Python include:
If Statements
The “if” statement is the simplest form of a conditional statement. It executes a block of code only if the specified condition is True. Here’s a simple example:
age = 18
if age >= 18:
print("You are eligible to vote!")
In this case, the message “You are eligible to vote!” will be printed because the condition age >= 18
is True.
Elif Statements
The “elif” (short for “else if”) statement allows you to check multiple expressions for True and execute a block of code as soon as one of the conditions is true. Here’s how it works:
age = 17
if age >= 18:
print("You are eligible to vote!")
elif age > 15:
print("You are a teenager!")
else:
print("You are just a kid!")
In this example, since age is 17, the output will be “You are a teenager!”
Else Statements
The “else” statement captures any scenario not covered by the previous “if” and “elif” statements. Simply put, it acts as a catch-all condition:
age = 12
if age >= 18:
print("You are eligible to vote!")
elif age > 15:
print("You are a teenager!")
else:
print("You are just a kid!")
Since none of the previous conditions are met, the output will be “You are just a kid!”
Loops
Loops are the hardworking elves of Python, tirelessly repeating tasks until specific conditions are met. There are primarily two types of loops in Python:
For Loops
The “for” loop is used for iterating over a sequence (such as a list, tuple, dictionary, set, or string) and executing a block of code for each item in the sequence:
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
This loop will print each fruit in the list:
apple
banana
cherry
While Loops
The “while” loop repeats a block of code as long as a specified condition is True:
count = 1
while count <= 3:
print("Counting", count)
count += 1
Output:
Counting 1
Counting 2
Counting 3
Branching Statements
Branching statements are used to change the flow of execution, skipping parts of the code or stopping the loop altogether. The most common branching statements are:
Break Statement
The “break” statement is used to terminate the loop prematurely:
for num in range(10):
if num == 5:
break
print(num)
This loop will print numbers from 0 to 4 and stop when it reaches 5.
Continue Statement
The “continue” statement skips the rest of the current iteration and moves on to the next iteration:
for num in range(10):
if num % 2 == 0:
continue
print(num)
This loop will print all odd numbers between 0 and 9 because it skips even numbers with the “continue” statement.
Real-World Applications
Control structures are not just theoretical; they have practical applications in real-world scenarios. For example, e-commerce websites use them to personalize user experiences, scientific computations rely on them for iterative calculations, and game development often leverages them for game logic and AI behaviors. Understanding and mastering these control structures will give you the tools to build more efficient and effective programs.
Additional Resources
Hungry for more? Dive deeper into Python’s control structures by exploring this comprehensive tutorial from Python.org. It’s an excellent resource to solidify your understanding and discover more advanced techniques.
Conclusion
And there you have it, folks! We’ve just navigated the fascinating world of Python’s control structures, equipping you with the knowledge to steer your code with precision and flair. Remember, the beauty of programming lies in its endless possibilities, and mastering these control structures is a significant step toward harnessing that power.
So, gear up, start experimenting, and let your creativity flow through your code. Until next time, happy coding!