Data Structures in Go: Binary Search Trees vs. Hash Tables
Welcome to the enchanting world of data structures in Go! Today, we’re diving deep into two critical and often compared data structures: Binary Search Trees (BSTs) and Hash Tables. These structures are the backbone of efficient data organization and retrieval, and understanding them is essential for any Go developer. Ready to journey through the nuances and applications of BSTs and Hash Tables? Let’s get started!
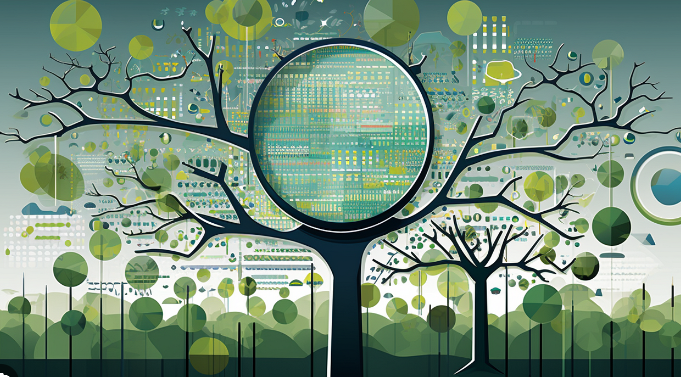
Binary Search Trees (BSTs)
Binary Search Trees are hierarchical data structures where each node has at most two children, referred to as the left child and the right child. The principle that governs a BST is simple yet powerful: for any given node, all values in the left subtree are less than the node’s value, and all values in the right subtree are greater.
Key Operations in BSTs
- Insertion: Starting from the root, compare the value to be inserted with the current node. If it’s smaller, move to the left child; if larger, move to the right child. This process continues until an appropriate null child is found, where the new node is inserted.
- Search: Similar to insertion, start from the root and compare the search value with the current node. Move left or right as necessary until the value is found or a null pointer is reached (indicating the value is not in the tree).
- Deletion: This is the most complex operation and includes three main cases: (1) deleting a leaf node, (2) deleting a node with one child, and (3) deleting a node with two children.
type Node struct {
Key int
Left *Node
Right *Node
}
func insert(node *Node, key int) *Node {
if node == nil {
return &Node{Key: key}
}
if key < node.Key {
node.Left = insert(node.Left, key)
} else {
node.Right = insert(node.Right, key)
}
return node
}
Advantages of BSTs
- Ordered Structure: BSTs inherently maintain order, which makes them suitable for use cases requiring sorted data.
- Efficient Search: BSTs can offer efficient search operations on average, with a time complexity of O(log n).
Drawbacks of BSTs
- Balancing Issues: In the worst case, a BST can degenerate into a linked list if elements are inserted in a sorted manner, leading to O(n) time complexity for search and insertion.
Hash Tables
Hash Tables use an underlying array and a hash function to map keys to their associated values. Unlike BSTs, Hash Tables do not maintain a sorted order, focusing instead on achieving an average constant-time complexity for insertion, deletion, and lookup operations.
Key Operations in Hash Tables
- Insertion: Apply the hash function to the key to determine its index in the array. If the spot is vacant, insert the key-value pair. If occupied, use techniques like chaining or open addressing to resolve collisions.
- Search: Hash the key to get the index and check the position in the array. Collisions are handled using the same technique used in insertion.
- Deletion: Hash the key, find the corresponding entry, and remove it. Again, the handling technique for collisions should be considered.
type HashTable struct {
array [100][]KeyValuePair
}
type KeyValuePair struct {
Key string
Value int
}
func (ht *HashTable) insert(key string, value int) {
index := hashFunction(key) % len(ht.array)
ht.array[index] = append(ht.array[index], KeyValuePair{Key: key, Value: value})
}
func hashFunction(key string) int {
hash := 0
for _, char := range key {
hash = 31*hash + int(char)
}
return hash
}
Advantages of Hash Tables
- Constant Time Complexity: On average, hash tables offer O(1) time complexity for insertion, lookup, and deletion.
- Efficient Memory Utilization: Hash tables can be highly space-efficient depending on the load factor.
Drawbacks of Hash Tables
- Collisions: Hash tables rely heavily on a good hash function. Poor hashing can lead to numerous collisions, degrading performance.
- No Order: Hash tables do not maintain any order, which can be a limitation for applications that need sorted data.
Comparing BSTs and Hash Tables
Both BSTs and Hash Tables have their place in a developer’s toolkit, and choosing between them depends on the specific requirements of your application. Here’s a quick comparison to help you decide:
Feature | Binary Search Trees | Hash Tables |
---|---|---|
Time Complexity (Average) | O(log n) | O(1) |
Ordered Data | Yes | No |
Handling Collisions | N/A | Yes |
Space Utilization | High | Efficient |
Conclusion
In the battle of Binary Search Trees versus Hash Tables, there is no one-size-fits-all answer. Binary Search Trees excel when you need ordered data with reasonable search times, whereas Hash Tables shine in scenarios demanding rapid lookups and insertions. Whichever structure you choose, mastering both will undoubtedly elevate your Go programming skills!
Curious for more? Keep exploring and experimenting with these fascinating data structures. The world of Go is brimming with opportunities, and each new concept is a stepping stone to becoming a better developer. Happy coding!
If you wish to dive deeper into Go and its algorithms, you can check out the detailed documentation on the official Go website.