Taking Dynamic Programming in Go to the Next Level: Fibonacci Sequence (Part III)
Hello, tech enthusiasts! ? Welcome to the third installment in our series on unravelling the mystery of the Fibonacci sequence using dynamic programming in Go. If you’re just joining us, I highly recommend checking out Part I and Part II to get up to speed. Trust me, you won’t want to miss out on those foundational gems! ?
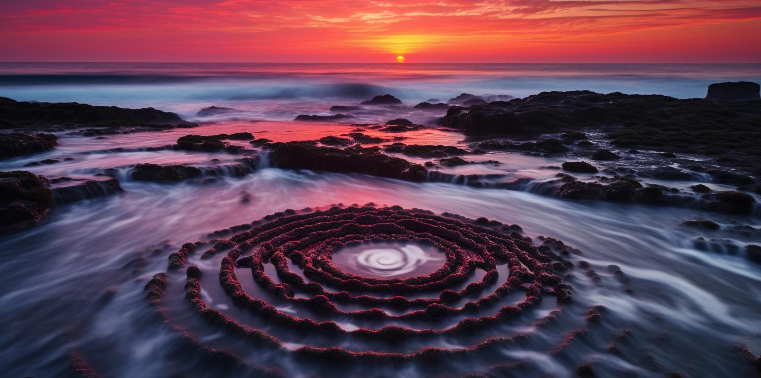
From Good to Great: Optimizing Space Complexity
In our previous discussions, we tackled both the naive recursive approach and the improved dynamic programming technique for calculating Fibonacci numbers. However, there’s always room for improvement! Today, we’ll explore optimizing the space complexity of our dynamic programming solution.
Recap: The Dynamic Programming Approach
As you might recall, the dynamic programming approach we used in Part II involves storing intermediate Fibonacci values in an array to avoid redundant calculations. Here’s the original implementation:
package main
import "fmt"
func fibonacci(n int) int {
if n <= 1 {
return n
}
f := make([]int, n+1)
f[0], f[1] = 0, 1
for i := 2; i <= n; i++ {
f[i] = f[i-1] + f[i-2]
}
return f[n]
}
func main() {
fmt.Println(fibonacci(10)) // Output: 55
}
This code efficiently calculates Fibonacci numbers with a time complexity of O(n) but uses an array of size O(n), which isn’t the most space-efficient. Let’s optimize this!
Optimized Dynamic Programming: Reducing Space Complexity
Although the above code is efficient, we can further optimize space complexity by realizing that we don’t need to store all the Fibonacci numbers up to n
. Instead, we only need the last two numbers at any given time. Let’s update our implementation:
package main
import "fmt"
func fibonacci(n int) int {
if n <= 1 {
return n
}
a, b := 0, 1
for i := 2; i <= n; i++ {
a, b = b, a+b
}
return b
}
func main() {
fmt.Println(fibonacci(10)) // Output: 55
}
By using two variables instead of an array, we drastically reduce the space complexity to O(1) while maintaining the same time complexity of O(n). This makes our solution both time and space efficient. Isn’t that awesome? ?
Beyond Fibonacci: Expanding Dynamic Programming Horizons
While the Fibonacci sequence is an excellent gateway into dynamic programming, the principles we’ve discussed go far beyond it. Here are a few other exciting and complex problems where dynamic programming shines:
- Longest Common Subsequence
- Knapsack Problem
- Edit Distance (Levenshtein Distance)
- Matrix Chain Multiplication
- Minimum Path Sum in a Grid
These problems vary in their complexity and applications, but the dynamic programming approach used for Fibonacci can be adapted and extended to solve them. If you’re curious, the Wikipedia article on dynamic programming is a fantastic resource!
Wrapping Up: The Joy of Learning and Innovating
We’ve journeyed through three fantastic posts together, exploring the Fibonacci sequence from naive recursion to efficient dynamic programming, and now optimizing space complexity. I hope these discussions have lit a spark of curiosity and enthusiasm within you to dive deeper into the world of dynamic programming with Go! ?
I encourage you to experiment with these concepts and apply them to new problems. The joy of coding lies in constant learning and relentless innovation. Keep pushing the boundaries, and who knows, you might just unearth the next big algorithmic breakthrough. ?
Let’s Keep the Conversation Going!
Your feedback and questions are invaluable. Drop a comment below or reach out through social media. Let’s keep this learning journey interactive and fun! Also, stay tuned for more exciting tech tutorials as we explore the endless possibilities of programming together.
Keep coding and stay optimistic! ?