Understanding Interpretation in Python: Execute Code Directly with Ease!
Hey there, tech enthusiasts! ? Do you know what makes Python such a beloved language among developers all over the world? Well, aside from its simplicity and readability, it’s the fact that Python is an interpreted language! But what exactly does that mean? Buckle up, because we’re about to dive into the world of interpretation in Python, where you can execute code directly with a sprinkle of magic and a whole lot of efficiency!
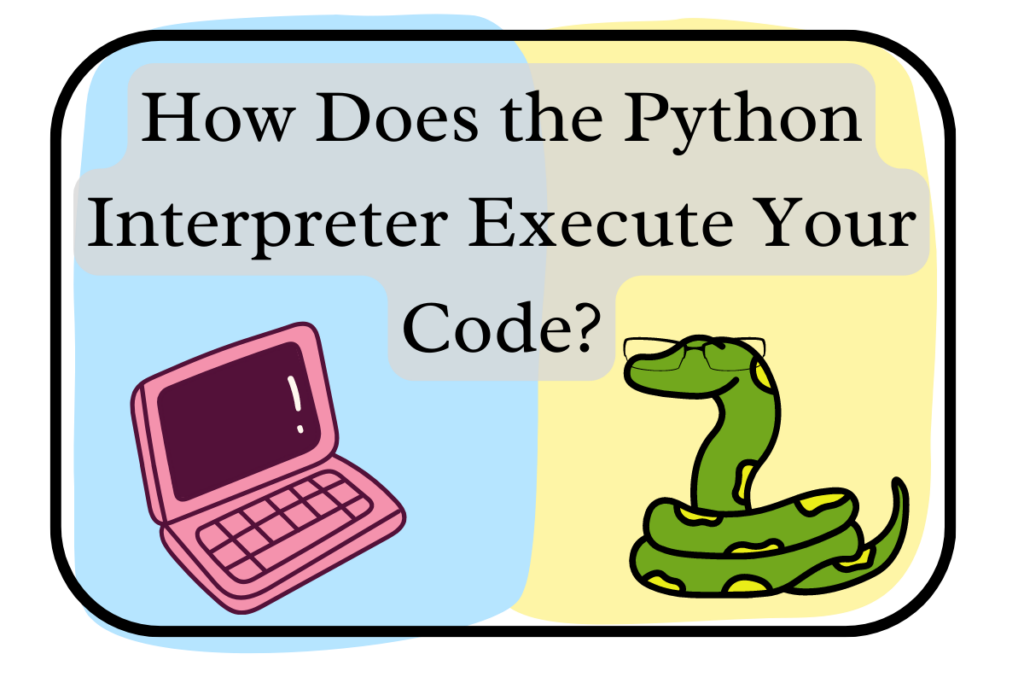
What is an Interpreted Language?
Before we dive into Python specifically, let’s get a grasp of what it means for a language to be interpreted. In the world of programming languages, there are primarily two types of languages: compiled and interpreted.
A compiled language is a language where your code is translated into machine code, or bytecode, before it is run. This means the entire program is converted into an executable file, which can then be run on a computer. Examples include C++ and Rust.
On the other hand, an interpreted language like Python executes statements line by line. Instead of converting the entire program into a machine code file, an interpreter reads the code and performs the actions specified, all in real-time. This direct execution process can make writing and testing code incredibly flexible and fun!
Why Python Shines as an Interpreted Language
Alright, so why is it so special that Python is interpreted? Here are several reasons why this makes Python a standout choice for many developers:
- Interactive Nature: Python’s interpretive nature allows for the interactive console, often referred to as the Python shell or REPL (Read-Eval-Print Loop). This is a lifesaver for testing bits of code on the fly. Spin up your terminal, type
python
(orpython3
), and you’re there! - Portability: Since Python code is interpreted and not compiled into a binary file, Python programs can be run on any platform with a compatible interpreter. Write once, run anywhere!
- Ease of Troubleshooting: Because Python executes code line-by-line, it can provide immediate feedback on errors. This helps in debugging and maintaining code more effectively.
- Flexibility for Development: Python’s interpretive approach makes iterative development a breeze. Developers can write and test chunks of code quickly, making the development process more agile and dynamic.
How Python Interprets Code
Now let’s get into the nitty-gritty of how Python interprets the code. When you run a Python script, the following steps are typically involved:
Step 1: Parsing
When you write Python code, the first thing the interpreter does is parse it. Parsing involves analyzing the syntax and structure of the code. If your code doesn’t follow Python’s syntax rules, you’ll get syntax errors at this stage. The parser converts your code into an intermediate form known as the Abstract Syntax Tree (AST).
Step 2: Compiling to Bytecode
After parsing, the AST is then compiled into Python bytecode. This is an intermediate, lower-level form of your code, which is platform-independent. The compiled bytecode is stored in a file with a .pyc extension. This step is often hidden from the user but is crucial for efficiency.
Step 3: Instruction Execution by the Virtual Machine
The bytecode is then executed by Python’s Virtual Machine (VM). The VM parses these bytecode instructions and performs the necessary operations. It’s important to note that this bytecode is specific to Python and is handled by the interpreter, not the underlying operating system.
Let’s See It in Action!
Enough with the theory; let’s see some real-world examples of interpreting Python code!
Example 1: Python Interactive Shell
python
>>> print("Hello, World!")
Hello, World!
>>> x = 5
>>> y = 2
>>> x + y
7
In the interactive shell, Python reads your input, evaluates it, and prints the result. It’s a great tool for quick calculations or testing small chunks of code.
Example 2: Running a Script
Create a new file called hello.py
and add the following code:
python
def greet(name):
return f"Hello, {name}!"
if __name__ == "__main__":
print(greet("Alice"))
Now, run the script by typing python hello.py
in your terminal, and you should see:
python
Hello, Alice!
Notice how Python executed the script line by line until the end. No need for compiling the code separately!
Best Practices for Python Code Execution
Here are some tips to ensure your interpreted Python code runs smoothly and efficiently:
- Use Virtual Environments: Always use virtual environments to manage dependencies and avoid conflicts with different versions of libraries.
- Write Readable Code: Since Python code is executed line-by-line, following the PEP 8 style guide makes it easier to read and debug your code.
- Optimize Code: Even though Python is an interpreted language, you can optimize the performance of your code by using efficient algorithms and data structures.
- Utilize Existing Libraries: Python has a rich ecosystem of libraries. Leverage them to avoid reinventing the wheel and speed up your development process.
Conclusion
And there you have it, folks! Python’s status as an interpreted language is one of its biggest strengths, offering interactivity, portability, and flexibility. Whether you are just starting out or are a seasoned developer, Python’s interpretive nature allows you to write and test code quickly and efficiently.
For more in-depth understanding of how Python’s interpretation works, you can check out the official Python Documentation.
So go ahead and dive into the magical world of interpreting Python code. You’ll find it dynamic, engaging, and downright fun! Happy coding! ?