Error Handling in Python: Advanced Techniques for Handling Exceptions
Welcome back, dear readers! In our previous posts on error handling in Python, we delved into the basics and some intermediate techniques. Today, let’s go even further and explore some advanced tactics for managing runtime errors in Python. Buckle up; it’s going to be an exciting ride!
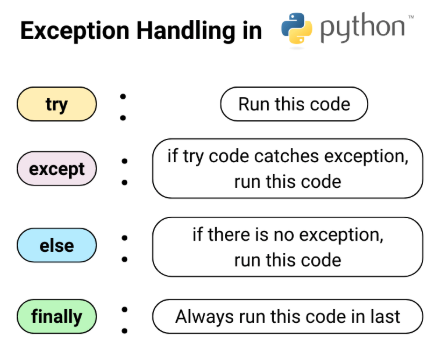
Custom Exception Classes
Sometimes, the built-in exceptions in Python just don’t cut it for specific scenarios in your application. That’s where custom exception classes come into play. By defining your custom exceptions, you can make your error handling more descriptive and fine-tuned to your needs.
class MyCustomError(Exception):
"""Exception raised for custom error conditions."""
pass
try:
raise MyCustomError("Something went wrong!")
except MyCustomError as e:
print(f"Custom error caught: {e}")
In this example, MyCustomError
lets you create more specific and meaningful error messages, helping users and developers debug your code more effectively.
The `raise` Statement
We briefly touched on the raise
statement before, but it deserves a deeper dive. This statement is invaluable when you need to deliberately trigger an exception, either to signal an error condition or to re-raise an exception caught in an except block.
def some_function(value):
if value < 0:
raise ValueError("Value must be non-negative")
return value ** 2
try:
result = some_function(-5)
except ValueError as ve:
print(f"Value Error: {ve}")
Here, the raise
statement helps enforce a constraint by explicitly throwing a ValueError
when the input value is negative.
Exception Chaining
Exception chaining allows a new exception to be raised while preserving the context of the initial exception. This is particularly useful for debugging, as it maintains the stack trace of the original error.
try:
1 / 0
except ZeroDivisionError as zde:
raise RuntimeError("A runtime error occurred") from zde
In this example, the ZeroDivisionError
is caught but then an additional RuntimeError
is raised with the original exception attached. This creates a chain that can be invaluable for understanding the full context of the error.
Context Management for Clean-up Tasks
Effective error handling isn’t just about catching exceptions; it also involves cleaning up resources. Python’s context managers, implemented using the with
statement, make this easier.
with open('file.txt', 'r') as file:
content = file.read()
print(content)
The with
statement ensures that the file is properly closed regardless of whether an error occurs within the block, simplifying your error management and resource handling.
Logging Errors
Logging is a crucial part of any robust error handling strategy. Instead of merely printing error messages, consider using Python’s logging
module to record errors. This gives you flexibility in how and where to record error information.
import logging
logging.basicConfig(filename='app.log', level=logging.ERROR)
try:
1 / 0
except ZeroDivisionError as zde:
logging.error("An error occurred: %s", zde)
With this setup, errors are logged to a file rather than displayed on the console, making it easier to track and analyze them later.
Unit Testing Your Error Handling
Last but not least, don’t forget to test your error handling code! Unit tests can ensure that your exception handling works as expected. Python’s unittest
framework provides tools for checking whether code raises the correct exceptions.
import unittest
def divide(a, b):
if b == 0:
raise ValueError("Cannot divide by zero")
return a / b
class TestDivision(unittest.TestCase):
def test_divide_by_zero(self):
with self.assertRaises(ValueError):
divide(10, 0)
if __name__ == "__main__":
unittest.main()
Here, the test_divide_by_zero
method ensures that our divide
function raises a ValueError
when attempting to divide by zero.
Conclusion
And there you have it! With these advanced techniques, you’re well on your way to mastering error handling in Python. Whether you’re defining custom exceptions, chaining errors, managing resources, logging issues, or testing your code, remember that robust error handling is crucial for building reliable applications.
Stay tuned for more exciting posts and keep pushing the boundaries of what’s possible. And as always, happy coding!