Error Handling in Python vs. Go: How Each Language Helps Beginners Navigate and Solve Programming Errors
Hello there, fellow tech enthusiasts! Today, we embark on an exciting journey to explore how Python and Go handle errors and how each language assists beginners in navigating and solving these pesky interruptions in the flow of our code. Buckle up for a deep dive into these two popular languages and discover how they guide us towards error-free programming! ?
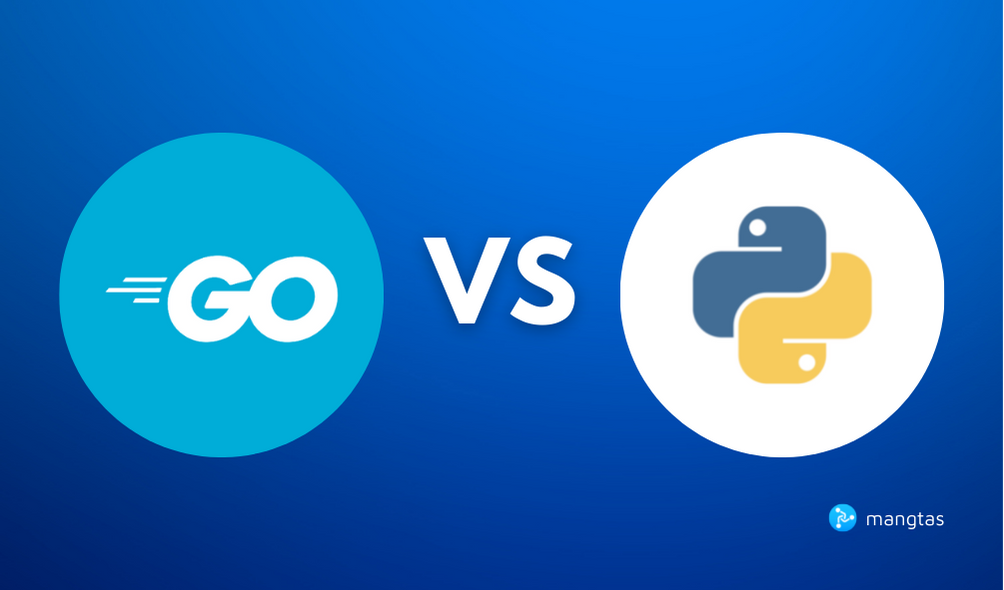
Understanding Error Handling in Programming
Before we get into the specifics, let’s quickly review what error handling in programming actually means. Error handling is the process of responding to and managing errors that occur during the execution of a program. Effective error handling ensures that our programs can deal with unexpected situations without crashing or producing incorrect results.
Python: The Beginner-Friendly Language
Python is well-known for its simplicity and readability, making it a favorite among beginners. Its error handling mechanism is no exception. Let’s examine how Python helps newcomers manage errors.
Try-Except Blocks
One of the core components of Python’s error handling is the try-except statement. It allows you to write code that could potentially raise an error and provides a mechanism to handle that error gracefully.
try:
# Code that might raise an error
result = 10 / 0
except ZeroDivisionError:
# Code to handle the error
result = None
print("Oops! You can't divide by zero.")
In this example, if an attempt is made to divide by zero, Python catches the `ZeroDivisionError` and executes the code in the `except` block. It’s clear, concise, and easy for beginners to grasp.
Specificity in Exceptions
Python encourages handling specific exceptions, enabling detailed error management. For instance, let’s say we want to handle both `ZeroDivisionError` and a `TypeError`.
try:
result = 10 / "a"
except ZeroDivisionError:
result = None
print("Oops! You can't divide by zero.")
except TypeError:
result = None
print("Oops! Incompatible types for division.")
This approach ensures that each error type is handled appropriately, enhancing the robustness of your programs.
Else and Finally Blocks
Python’s `try-except` construct comes with powerful allies, the `else` and `finally` blocks. The `else` block runs if no exception was raised, and the `finally` block always runs, regardless of whether an exception occurred.
try:
result = 10 / 2
except ZeroDivisionError:
result = None
print("Oops! You can't divide by zero.")
else:
print(f"Success! The result is {result}")
finally:
print("Execution complete.")
This comprehensive error handling strategy helps beginners write robust and reliable code.
Go: The Robust Systems Language
Go, or Golang, is designed for systems programming and large-scale applications. It has a different approach to error handling, aimed at encouraging developers to think actively about potential errors.
Returning Errors Explicitly
In Go, errors are treated as values and are explicitly returned from functions. This makes error handling an integral part of the function’s control flow.
package main
import (
"fmt"
"errors"
)
func divide(a, b float64) (float64, error) {
if b == 0 {
return 0, errors.New("cannot divide by zero")
}
return a / b, nil
}
func main() {
result, err := divide(10, 0)
if err != nil {
fmt.Println("Error:", err)
} else {
fmt.Println("Result:", result)
}
}
In this example, the `divide` function returns an error if the divisor is zero, and the calling code checks for and handles the error accordingly. This explicit handling encourages beginners to be vigilant about potential errors and helps prevent bugs.
Custom Error Types
Go allows the creation of custom error types, adding granularity to error handling. This can be particularly useful for large projects.
package main
import (
"fmt"
)
type DivisionError struct {
numerator float64
denominator float64
}
func (e *DivisionError) Error() string {
return fmt.Sprintf("cannot divide %v by %v", e.numerator, e.denominator)
}
func divide(a, b float64) (float64, error) {
if b == 0 {
return 0, &DivisionError{a, b}
}
return a / b, nil
}
func main() {
result, err := divide(10, 0)
if err != nil {
fmt.Println("Error:", err)
} else {
fmt.Println("Result:", result)
}
}
Here, we define a custom `DivisionError` type, which provides detailed information about the error. This approach is highly instructive for beginners, as it encourages more thoughtful and descriptive error handling.
Comparing Python and Go
Let’s wrap up by comparing the error handling techniques in Python and Go, shedding light on how each caters to beginners.
Python
– Ease of Use: Python’s `try-except` blocks are straightforward and easy to understand.
– Readability: The syntax is very readable, making error handling less intimidating for beginners.
– Specificity: The ability to handle specific exceptions improves code quality and clarity.
Go
– Explicit Handling: Go’s explicit error returns force developers to consider errors as part of normal function responses.
– Custom Errors: Creating custom error types helps in creating detailed and meaningful error messages.
– Control Flow: Integrating error handling into control flow makes Go programs more predictable and reliable.
Conclusion: Embrace the Errors!
Both Python and Go have robust error handling mechanisms that cater to beginners, albeit in different ways. Python’s approach is highly intuitive and forgiving, allowing newcomers to smoothly navigate through errors without much fuss. On the other hand, Go’s explicit error returns and custom error types encourage a more disciplined and mindful approach to error management.
No matter which path you choose, remember that encountering errors is a crucial part of the learning journey. Embrace them, learn from them, and soon, you’ll be crafting error-free code like a pro! ?
If you’re eager to dive deeper into error handling or explore other programming concepts, check out more posts on our Sesame Disk Blog. Happy coding, and may your journey be ever-exciting and error-free! ?