Custom Allocators, Object Interning, and Garbage Collection
Welcome Back to the Marvelous World of Python Memory Management! ? First of all, a big thanks for sticking with us throughout our journey into Python memory management. In our previous posts, we covered the basics and advanced techniques of memory management, profiling, debugging, and optimization.
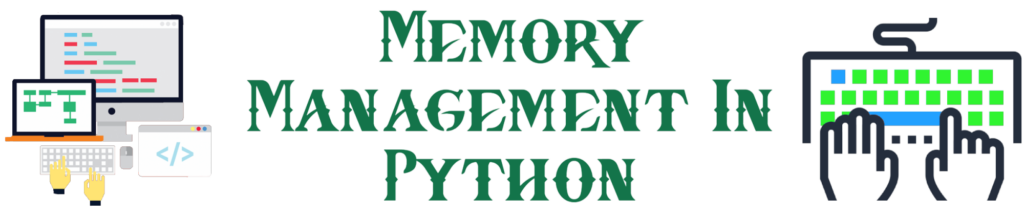
In case you missed them, here are the quick links for a quick refresher:
1. Mastering Memory Management in Python: A Comprehensive Guide to Allocating and Deallocating Memory
2. Advanced Techniques in Python Memory Management: Profiling, Debugging, and Optimization
3. Delving Deeper into Python Memory Management: Tools & Best Practices
Today, we’ll build on that foundation and explore some cutting-edge techniques for optimizing Python memory management even further. This post will keep you hooked with insights and practical tips, all served with a sprinkle of good humor and enthusiasm. Let’s dive right in!
Understanding the Python Memory Model
Before we get into the tips and tricks, let’s have a quick recap of the Python memory model. In Python, memory management includes allocation and deallocation of memory for data structures in your application. Python handles a lot of memory management automatically with its built-in garbage collector, yet understanding how it works can help us make our programs more efficient.
Python uses reference counting and a cyclic garbage collector to track objects that are no longer in use. When an object’s reference count drops to zero, it can be automatically deallocated. The cyclic garbage collector handles more complex scenarios where reference cycles might prevent the reference count from reaching zero.
Fine-Tuning Object Handling
Here’s where we can play our card to optimize memory handling. By maintaining a watchful eye on how objects are created, reused, and destroyed, we can squeeze out performance gains and avoid the dreaded memory leaks.
Using `__slots__` for Memory Efficiency
If you’re dealing with many instances of the same class and these instances have a limited number of attributes, you might consider using `__slots__`. It allows you to explicitly declare data members (like in a C struct) and can significantly reduce the memory footprint.
class MyClass:
__slots__ = ['attribute1', 'attribute2']
def __init__(self, attribute1, attribute2):
self.attribute1 = attribute1
self.attribute2 = attribute2
Careful Management of Dictionaries and Lists
Dictionaries and lists are fundamental to Python, but they can also be memory hogs if not managed carefully. Here are a few tips:
1. Reuse Dictionaries: Instead of frequently creating and destroying dictionaries, try to reuse them. This can prevent frequent memory allocations and deallocations, making your code more efficient.
2. Use Generators: Instead of creating lists for sequences that are only accessed once, use generators. They generate items on-the-fly and consequently use far less memory.
def big_data_generator():
for i in range(1000000):
yield i
Leveraging Built-in Modules
Modules like `array` and `collections` offer specialized data structures that are more memory efficient for specific tasks.
1. array module: Great for handling large arrays of basic data types.
import array
arr = array.array('i', [1, 2, 3, 4, 5])
2. collections.deque: More efficient than lists when it comes to appending and popping operations from both ends.
from collections import deque
my_queue = deque([1, 2, 3, 4, 5])
my_queue.append(6)
Memory Profiling: Your Secret Sauce
Profiling tools can be a game changer. They help you pinpoint exactly where your code is using (or wasting) memory. Here are a few notable ones:
1. memory_profiler: Offers line-by-line analysis of memory usage in your code.
2. pympler: Provides detailed summaries of how much memory your objects are consuming.
from memory_profiler import profile
@profile
def my_function():
a = [i for i in range(10000)]
b = [i**2 for i in range(10000)]
return a, b
You can analyze the output and make adjustments based on the areas consuming the most memory.
Keep an Eye on Reference Cycles
Reference cycles are situations where objects refer to each other, creating a loop that prevents Python’s reference counting mechanism from deallocating them. Although Python’s cyclic garbage collector can handle these scenarios, it’s good to be aware of and avoid creating them. Tools like gc module can help you manually trigger garbage collection and inspect objects that are uncollectable.
import gc
gc.collect()
Final Thoughts: The Path Ahead
So there you have it – taking Python memory management up a notch! By leveraging the techniques and tools outlined here, you’ll be equipped to craft efficient, nimble applications that make the most out of your system’s resources.
As always, Python’s universe is vast and ever-changing. The more you learn, the more you realize there is to know. Stay curious, keep experimenting, and you’ll continue to grow as a developer. Don’t forget to check out our previous posts linked above if you need a refresher!
Looking to optimize how Python manages memory? New tools and insights are always around the corner, so stay tuned, keep coding, and never stop learning. And remember: the key to efficient coding is not just writing code that works but writing code that works intelligently! ?✨
Until next time, happy coding! ?