Data Management in Go: Heap vs. Priority Queue
Welcome, fellow tech enthusiasts! Today we’re diving into the fascinating world of data management in Go. We’ll be exploring two critical structures: Heaps and Priority Queues. These structures are like the dynamic duos of data handling—each with its own strengths and use cases. Whether you’re an experienced Go developer or just starting to dabble in the language, this post will give you a solid understanding of when and how to use Heaps and Priority Queues.
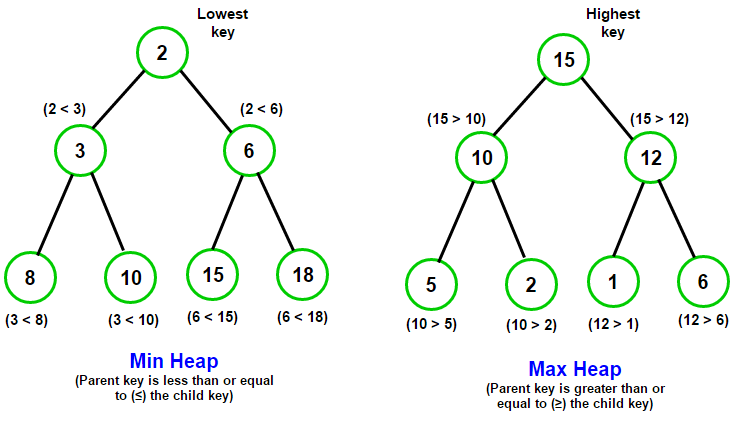
Understanding the Basics
Before we dive deep, let’s break down what Heaps and Priority Queues are.
Heap
A Heap is a specialized tree-based data structure that satisfies the heap property. If it’s a max heap, every parent node is greater than its child nodes. Conversely, in a min heap, every parent node is smaller than its child nodes. Heaps are commonly implemented using arrays.
Priority Queue
A Priority Queue is an abstract data type where each element has a “priority” associated with it. Elements are dequeued based on their priority. While a typical queue follows a First-In-First-Out (FIFO) order, a priority queue dequeues elements based on their priority, not arrival time.
Both data structures are integral for efficient data management, but they serve different purposes. Let’s look at their implementations in Go and understand their practical uses.
Implementing a Heap in Go
Go doesn’t have a built-in heap, but it has a package named `container/heap` that provides heap operations. Here’s a basic implementation of a min heap:
package main
import (
"container/heap"
"fmt"
)
type MinHeap []int
func (h MinHeap) Len() int { return len(h) }
func (h MinHeap) Less(i, j int) bool { return h[i] < h[j] }
func (h MinHeap) Swap(i, j int) { h[i], h[j] = h[j], h[i] }
func (h *MinHeap) Push(x interface{}) {
*h = append(*h, x.(int))
}
func (h *MinHeap) Pop() interface{} {
old := *h
n := len(old)
x := old[n-1]
*h = old[0 : n-1]
return x
}
func main() {
h := &MinHeap{2, 1, 5}
heap.Init(h)
heap.Push(h, 3)
fmt.Printf("minimum: %d\n", (*h)[0])
for h.Len() > 0 {
fmt.Printf("%d ", heap.Pop(h))
}
}
In this code:
– We define `MinHeap` to be a type of `[]int`.
– We implement the `heap.Interface` interface with methods: `Len`, `Less`, `Swap`, `Push`, and `Pop`.
– We initialize the heap, push a new element into it, and then pop all elements while printing them.
Implementing a Priority Queue in Go
Priority Queues can also be implemented using Go’s `container/heap` package. Let’s look at an example:
package main
import (
"container/heap"
"fmt"
)
type Item struct {
value string
priority int
index int
}
type PriorityQueue []*Item
func (pq PriorityQueue) Len() int { return len(pq) }
func (pq PriorityQueue) Less(i, j int) bool {
return pq[i].priority > pq[j].priority // for max-heap
}
func (pq PriorityQueue) Swap(i, j int) {
pq[i], pq[j] = pq[j], pq[i]
pq[i].index = i
pq[j].index = j
}
func (pq *PriorityQueue) Push(x interface{}) {
n := len(*pq)
item := x.(*Item)
item.index = n
*pq = append(*pq, item)
}
func (pq *PriorityQueue) Pop() interface{} {
old := *pq
n := len(old)
item := old[n-1]
item.index = -1
*pq = old[0 : n-1]
return item
}
func main() {
items := map[string]int{
"orange": 3, "banana": 2, "apple": 4, "pear": 1,
}
pq := make(PriorityQueue, len(items))
i := 0
for value, priority := range items {
pq[i] = &Item{
value: value,
priority: priority,
index: i,
}
i++
}
heap.Init(&pq)
item := &Item{
value: "papaya",
priority: 5,
}
heap.Push(&pq, item)
for pq.Len() > 0 {
item := heap.Pop(&pq).(*Item)
fmt.Printf("%s: %d\n", item.value, item.priority)
}
}
In this code:
– We define an `Item` type to hold our value and priority.
– We implement `heap.Interface` with methods: `Len`, `Less`, `Swap`, `Push`, and `Pop`.
– We create a Priority Queue, initialize it with items, and then push an additional item.
– We finally pop all items to ensure they are dequeued based on priority.
When to Use Heaps vs. Priority Queues
Choosing between Heaps and Priority Queues depends on your requirements:
– Use Heaps when you need a simple and efficient way to get the smallest or largest item quickly.
– Use Priority Queues when you need to manage elements with specific priorities and need to dequeue them based on priority.
For instance, a heap is perfect for implementing an efficient sorting algorithm like Heap Sort, while a priority queue might be used in a task scheduling system where tasks with higher priorities need to be executed first.
Performance Considerations
Both heaps and priority queues have similar time complexities:
– Insertion: O(log n)
– Deletion: O(log n)
– Accessing the min/max (or high priority) element: O(1)
However, these structures are not ideal for situations where you need quick access to any arbitrary element or need to frequently update element priorities.
Wrapping Up
Understanding when and how to use Heaps and Priority Queues in Go can significantly improve your program’s efficiency and performance. While Heaps provide a straightforward way to manage ordered data, Priority Queues excel in scenarios requiring custom priority handling.
For further reading on Go’s `container/heap` and its intricacies, you can check the official Golang documentation.
Keep experimenting, keep learning, and happy coding! And remember, in the whimsical words of Bjarne Stroustrup, “Our civilization runs on software.” So, let’s keep our software efficient and our days delightful!
Stay curious and until next time! ?