Diving Deeper: Optimizing Fibonacci with Dynamic Programming
Welcome back, fellow coders! If you’ve been following our previous post, you’ve got a solid understanding of how dynamic programming can dramatically optimize our computation of the Fibonacci sequence. Today, we’re going to build on that foundation, exploring more advanced techniques and optimizations.
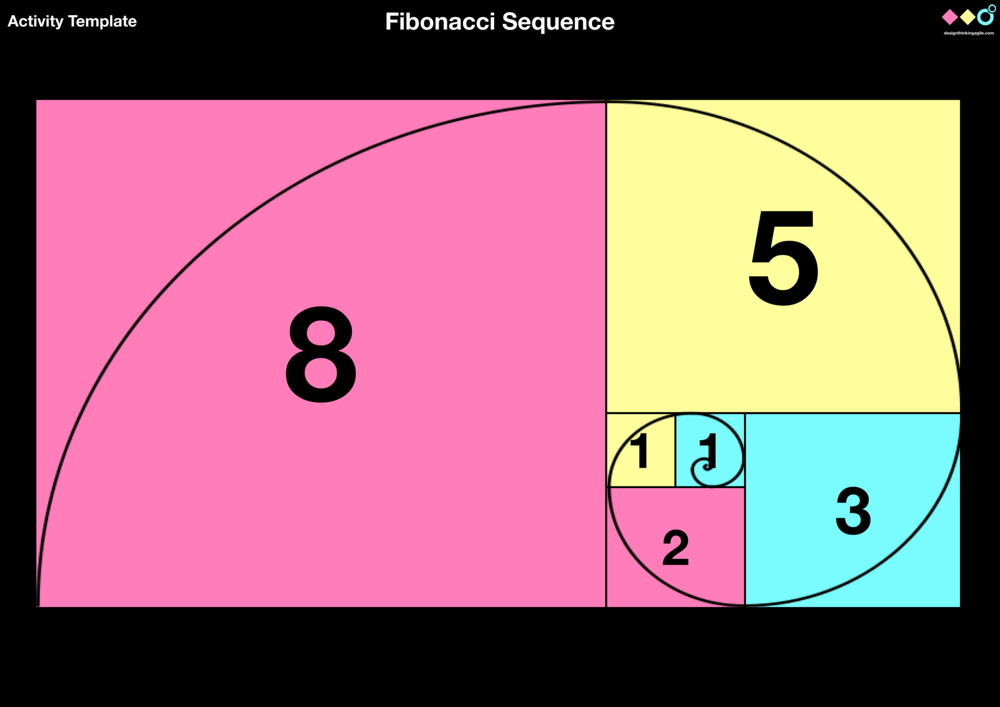
Revisiting Dynamic Programming: Memoization and Tabulation
In our last discussion, we touched on two key dynamic programming (DP) strategies: memoization and tabulation. Let’s have a quick recap before we dive deeper:
- Memoization: This is a top-down approach where we store the results of expensive function calls and reuse them when the same inputs occur again.
- Tabulation: Contrary to memoization, tabulation is a bottom-up technique that builds a table in a step-by-step manner and uses this table to solve the problem.
In this post, we’ll look at how these methods can be further optimized and even combined with other techniques for more efficient solutions.
Optimizing Memoization
Memoization is already a giant leap over naive recursion, but there’s always room for improvement!
Memoization is an optimization technique used primarily to speed up computer programs by storing the results of expensive function calls and reusing those results when the same inputs occur again. Instead of computing the result of a function every time it’s called with the same arguments, memoization saves the result after the first computation and returns the cached result on subsequent calls with the same arguments.
Let’s consider some advanced tweaks:
Using Built-in Tools
Python’s functools.lru_cache
is a powerful tool for memoization. By using this decorator, we can automatically memoize values without having to explicitly store them:
from functools import lru_cache
@lru_cache(maxsize=None)
def fibonacci(n):
if n < 2:
return n
return fibonacci(n-1) + fibonacci(n-2)
This is a clean, efficient way to handle memoization, ensuring that our Fibonacci function is both easy to read and performant.
Optimizing the Base Case
Another crucial aspect is correctly optimizing the base case. In our previous example, we handle n < 2
within the function. While this may seem trivial, ensuring a clear and concise base case helps avoid unnecessary computations, especially in large-scale applications.
Enhancing Tabulation with Space Optimization
Tabulation can also be optimized, especially regarding space complexity. Typically, tabulation uses an array of size n
to store Fibonacci numbers. However, since we only need the last two Fibonacci numbers at any step, we can reduce space complexity from O(n)
to O(1)
:
def fibonacci(n):
if n == 0:
return 0
elif n == 1:
return 1
a, b = 0, 1
for _ in range(2, n+1):
c = a + b
a = b
b = c
return b
This method only uses a constant amount of space, storing just the last two Fibonacci numbers while iterating, resulting in significant memory savings.
Combining Dynamic Programming with Matrix Exponentiation
If you’re looking for blazing fast Fibonacci computation, combining DP with matrix exponentiation is the way to go. This technique utilizes matrix multiplication to achieve a time complexity of O(log n)
, making it far superior for very large n
. Here’s how it’s done:
import numpy as np
def fibonacci_matrix(n):
F = np.matrix([[1, 1], [1, 0]])
if n == 0:
return 0
F = np.linalg.matrix_power(F, n-1)
return F[0, 0]
print(fibonacci_matrix(10)) # Outputs: 55
In this approach, we leverage NumPy’s efficient matrix operations, providing a powerful and performant solution to our Fibonacci problem.
Exploring Further: Fibonacci in Machine Learning and Beyond
Dynamic programming and the Fibonacci sequence don’t just halt at algorithm classes. These concepts permeate various aspects of technology, including machine learning algorithms and financial models. Understanding and optimizing such fundamental algorithms can provide a robust foundation for tackling more complex problems.
Conclusion: A Continuous Learning Journey
We’ve explored various facets of dynamic programming and its application in computing the Fibonacci sequence. From memoization and tabulation to matrix exponentiation, each method offers unique advantages. Remember, mastering these techniques not only enhances your problem-solving toolkit but also prepares you for a vast array of real-world applications.
We hope this journey has been as enlightening for you as it has been for us. Stay curious, keep coding, and always strive for optimization. Until next time, happy coding!
If you enjoyed this deep dive, don’t forget to check back regularly for more insightful posts. And of course, feel free to share your thoughts and questions in the comments below. Let’s keep the conversation going!