What Have We Learned So Far?
In our previous discussion on graph algorithms in Go, we delved into the core concepts of graph theory, and explored the differences between Breadth-First Search (BFS) and Depth-First Search (DFS). If you haven’t read that post yet, you can find it here. Today, we’ll build on that foundation, diving deeper into how BFS and DFS can be implemented in Go, and why they are crucial for understanding connectivity in graphs.
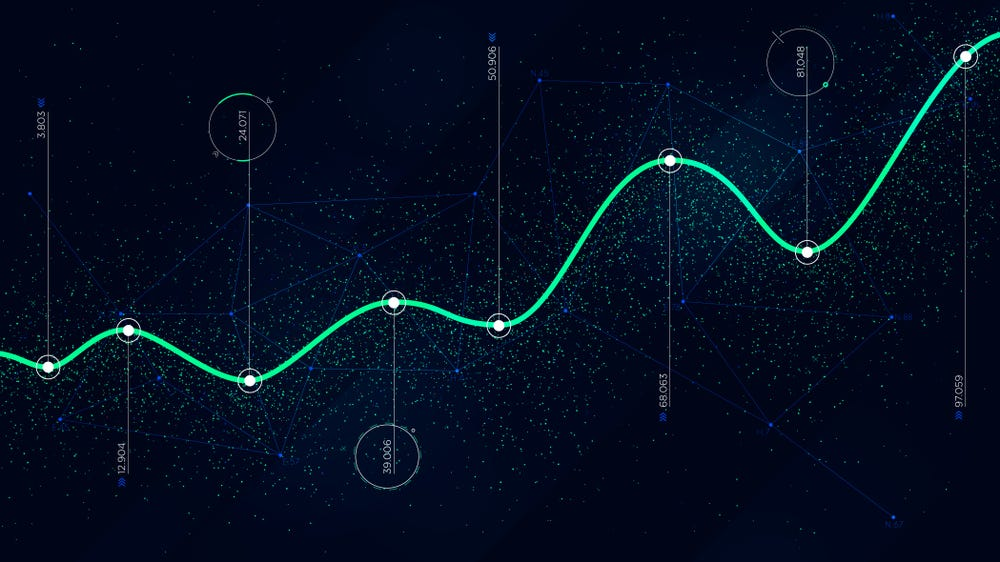
The Breadth and Depth of Connectivity
Why Is Connectivity Important?
Connectivity in a graph determines whether there’s a path between any two nodes. This is vital in real-world applications such as network design, social networks, and even in biological networks! By verifying connectivity, you can ensure robustness and efficiency in these systems.
Implementing BFS in Go: A Refresher
Remember, BFS explores all neighbors at the present depth level before moving on to nodes at the next depth level. Here’s a quick reminder of the BFS implementation in Go:
package main
import (
"container/list"
"fmt"
)
type Graph struct {
nodes int
adjacent map[int][]int
}
func NewGraph(nodes int) *Graph {
return &Graph{
nodes: nodes,
adjacent: make(map[int][]int),
}
}
func (g *Graph) AddEdge(node1, node2 int) {
g.adjacent[node1] = append(g.adjacent[node1], node2)
g.adjacent[node2] = append(g.adjacent[node2], node1)
}
func (g *Graph) BFS(start int) {
visited := make([]bool, g.nodes)
queue := list.New()
visited[start] = true
queue.PushBack(start)
for queue.Len() > 0 {
element := queue.Front()
currentNode := element.Value.(int)
fmt.Printf("%d ", currentNode)
queue.Remove(element)
for _, neighbor := range g.adjacent[currentNode] {
if !visited[neighbor] {
visited[neighbor] = true
queue.PushBack(neighbor)
}
}
}
}
func main() {
g := NewGraph(5)
g.AddEdge(0, 1)
g.AddEdge(0, 2)
g.AddEdge(1, 2)
g.AddEdge(2, 3)
g.AddEdge(3, 4)
fmt.Println("BFS starting from node 0:")
g.BFS(0)
}
Implementing DFS in Go: Dive Deeper!
In contrast, DFS explores as far as possible along each branch before backtracking. Here’s how you can implement DFS in Go:
package main
import "fmt"
type Graph struct {
nodes int
adjacent map[int][]int
}
func NewGraph(nodes int) *Graph {
return &Graph{
nodes: nodes,
adjacent: make(map[int][]int),
}
}
func (g *Graph) AddEdge(node1, node2 int) {
g.adjacent[node1] = append(g.adjacent[node1], node2)
g.adjacent[node2] = append(g.adjacent[node2], node1)
}
func (g *Graph) DFSUtil(node int, visited []bool) {
visited[node] = true
fmt.Printf("%d ", node)
for _, neighbor := range g.adjacent[node] {
if !visited[neighbor] {
g.DFSUtil(neighbor, visited)
}
}
}
func (g *Graph) DFS(start int) {
visited := make([]bool, g.nodes)
g.DFSUtil(start, visited)
}
func main() {
g := NewGraph(5)
g.AddEdge(0, 1)
g.AddEdge(0, 2)
g.AddEdge(1, 2)
g.AddEdge(2, 3)
g.AddEdge(3, 4)
fmt.Println("DFS starting from node 0:")
g.DFS(0)
}
BFS vs DFS: Which To Use?
Both BFS and DFS have their unique advantages and use cases. The choice between them depends on what you’re trying to achieve:
- BFS is excellent for finding the shortest path in unweighted graphs and for exploring nodes in layers. It’s optimal for scenarios such as peer-to-peer networks or web crawlers.
- DFS is more memory efficient than BFS and can handle large graph searches. It’s particularly useful for problems requiring path existence from a source node to a target node and in applications like puzzle-solving or topology-based problems.
Advanced Connectivity: Strongly Connected Components
For directed graphs, understanding connectivity goes beyond simple path existence. Strongly Connected Components (SCCs) are subgraphs where every vertex is reachable from every other vertex in the same subgraph. Identifying these SCCs is essential in areas like compiler optimizations and understanding web structures.
A well-known algorithm for finding SCCs is Tarjan’s algorithm. It’s a DFS-based approach that efficiently decomposes a graph into its strongly connected components. Discover more about Tarjan’s algorithm [here](https://en.wikipedia.org/wiki/Tarjan%27s_strongly_connected_components_algorithm).
Conclusion: Where To Go From Here?
There you go, folks! We’ve traversed through the essential graph algorithms—BFS and DFS—and gained insight into their applications and importance for understanding connectivity. Whether you’re crafting a social network or optimizing network routes, grasping these concepts is a game-changer.
Feel inspired? Ready to dive deeper? There’s so much more to explore in the universe of graph theory. From advanced algorithms to real-world applications, the world of graphs is as vast as it is fascinating.
Stay tuned for our next post, where we’ll tackle more advanced topics in Go and further our journey into the depths of programming and technology. Remember, the key to mastery is continuous learning, optimistic curiosity, and a dash of humor to keep things fun! Happy coding!
References
For more information on graph algorithms and their practical applications, check out this amazing resource: [Wikipedia – Graph Theory](https://en.wikipedia.org/wiki/Graph_theory).
Do keep in touch and feel free to leave comments or questions below. I look forward to exploring the limitless potential of technology with you, one exciting post at a time!