Ever wished your computer could read your mind and be your interpreter, translator, and guide? Wish no more… because of LangChain.
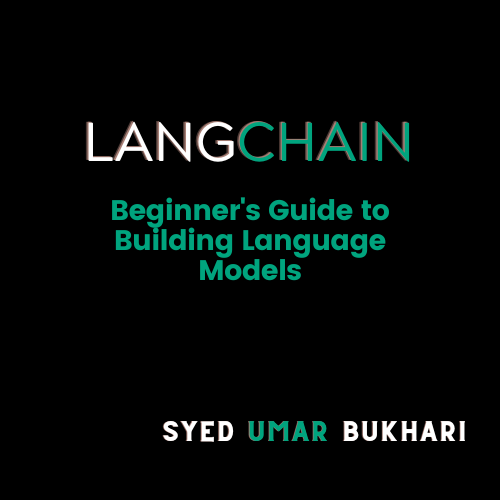
LangChain is a versatile and comprehensive framework designed for constructing applications around large language models (LLMs). It offers a structured approach to development by chaining together various components essential to language model applications. Moreover, these components include prompt templates, LLMs themselves, and agents that act as the interface between users and the language model.
Curious? Keep reading; you won’t believe what’s possible with LangChain! Don’t worry if you have never heard of it before– this article will walk you through the very basics.
A Framework for Building Language Models
At its core, LangChain provides a framework that simplifies the complex process of building, managing, and scaling applications that utilize language models. Unlike traditional development workflows where one has to handle the various moving parts of a language model application individually, LangChain offers an efficient and standardized way of managing these components.
Chains Together Different Components
Prompt Templates
These are pre-formulated prompts that can be used to instruct language models more effectively. Instead of coming up with a new prompt every time, developers can use reusable templates that help in eliciting more accurate and useful responses from the language models.
Large Language Models (LLMs)
LangChain is compatible with various large language models, such as GPT 4, LLaMA 2, PaLM, etc., and makes it easier to integrate them into applications. This eliminates the hassle of dealing with proprietary APIs or complex configurations.
Agents
These are the intermediaries between the users and the language model. Addtionally, they handle tasks like user input validation, data pre-processing, and routing the information to and from the language model.
Benefits of Using LangChain
LangChain offers a robust and streamlined approach to integrating language models into various applications. Its user-friendly and modular design addresses many challenges faced by developers, offering key advantages such as:
- Flexibility: Modular components make customization straightforward.
- Scalability: Built to grow with your project’s needs.
- Streamlined Development: Quick and efficient from ideation to deployment.
And that’s not all! It also solves the following problems:
- Complexity: Managing and deploying LLMs can be complex and time-consuming. And LangChain abstracts away this complexity, making it easy to use LLMs in your projects.
- Cost: LLMs can be expensive to train and deploy. LangChain provides a way to share LLMs, so you don’t have to train or deploy your own.
- Accuracy: LLMs can be very accurate, but they can also be biased. LangChain provides a way to mitigate bias in LLMs, so you can be confident in the results they produce.
Great, but how do I use this LangChain?
How to Get Started with LangChain
Getting started with LangChain is an easy process designed to be user-friendly. Hence, follow the steps below to quickly set up your environment and dive into building powerful applications with language models.
Requirements
Before getting started with LangChain, ensure you have the following prerequisites in place:
Software
- Python 3.6 or Higher: LangChain requires Python 3.6 or above. You can download the latest Python version from the official website.
Libraries
- OpenAI Python Package (Optional): If you plan on using OpenAI’s GPT models, you will need their Python package. This will be installed in the installation steps.
Accounts & API Keys
- OpenAI Account (Optional): If you plan to use OpenAI’s GPT models, you’ll need an OpenAI account to obtain an API key. Sign up here.
- ColossalAI Account (Optional): If you’re using ColossalAI, you’ll need to register and obtain an API key.
Hardware
- Memory & CPU: While LangChain is designed to be lightweight, the language models it interacts with can be resource-intensive.
Installation
- Install LangChain: Open your terminal and run the following command to install LangChain:
pip install langchain
- Install Dependencies: If you plan on using OpenAI’s model APIs, install the necessary Python package:
pip install openai
Environment Setup
- API Key Configuration: You’ll need to acquire an API key from the language model provider. For OpenAI, create an account and get the API key. After that, set it as an environment variable like so:
export OPENAI_API_KEY="your_openai_api_key_here"
Replace the string with your OpenAI key above. Now we can get started with the real development process!
Basic Usage
- Initialize Language Model: You can use various language models with LangChain. For this example, we will use ChatGPT by OpenAI and ColossalAI as our LLM (Large Language Model).
- Initialize ChatGPT:
from langchain.llms import OpenAI
chatgpt_llm = OpenAI(api_key=os.environ["OPENAI_API_KEY"], model="gpt-4.0-turbo")
- Initialize ColossalAI:
from langchain.llms import ColossalAI colossal_llm = ColossalAI(api_key="your_colossal_api_key_here")
- Create a Chain: LangChain allows you to create a chain consisting of an LLM, prompt templates, and agents to perform specific tasks. Here’s a simple example that uses a chain to answer questions.
from langchain import LLMChain, PromptTemplate, Agent
# Create a PromptTemplate for question answering
question_template = PromptTemplate("answer the question: {question}")
# Create an Agent to handle the logic
qa_agent = Agent(prompt_template=question_template, llm=llm)
# Create a chain
chain = LLMChain(agents=[qa_agent])
# Use the chain
response = chain.execute({"question": "What is the capital of France?"})
print(response)
This should print output like {'answer': 'The capital of France is Paris.'}
Not so hard, right? Next we focus on more specific prompts.
Create Prompt Templates and Agents
Now let’s create two specific prompt templates and agents for the chatbot functionality for ChatGPT and ColossalAI.
- Question Answering: Creating prompt template for Q/A.
question_template = PromptTemplate("Answer this question: {question}")
qa_agent = Agent(prompt_template=question_template, llm=chatgpt_llm)
2. Small Talk: Creating prompt template for small talk.
small_talk_template = PromptTemplate("Engage in small talk: {text}")
small_talk_agent = Agent(prompt_template=small_talk_template, llm=colossal_llm)
Then, we must get everything connected.
Chaining It All Together
Here we create a chain that consists of multiple agents to handle different tasks.
from langchain import LLMChain
chain = LLMChain(agents=[qa_agent, small_talk_agent])
# For question answering
qa_response = chain.execute({"question": "What is the capital of France?"})
print(qa_response) # Output: {'answer': 'The capital of France is Paris.'}
# For small talk
small_talk_response = chain.execute({"text": "How's the weather?"})
print(small_talk_response) # Output: {'response': 'The weather is lovely! How can I assist you further?'}
What if you want to change the language model you use for an agent? It’s simple and the next section discusses how to do that.
Switching Language Models
You can easily switch between different language models like ChatGPT and ColossalAI by changing the llm
parameter when initializing the agent.
# Switching to ColossalAI instead of ChatGPT for question answering
qa_agent = Agent(prompt_template=question_template, llm=colossal_llm)
# Use the chain again
qa_response = chain.execute({"question": "What is the capital of Japan?"})
print(qa_response) # Output should differ depending on the model.
What we’ve seen so far is merely the tip of the iceberg! Don’t scratch your head and keep reading to know how we can enhance the functionalities further!
Expanding LangChain Functionality with Additional Agents
LangChain allows for extra complexity by letting you include more than just question-answering and small talk in your chatbot.
Initialize Additional Agents
Below, we illustrate how to expand your existing chatbot setup to also handle tasks like sentiment analysis and language translation.
- Sentiment Analysis
entiment_template = PromptTemplate("Analyze sentiment: {text}")
sentiment_agent = Agent(prompt_template=sentiment_template, llm=chatgpt_llm)
2. Language Translation (English to Spanish)
translation_template = PromptTemplate("Translate from English to Spanish: {text}")
translation_agent = Agent(prompt_template=translation_template, llm=colossal_llm)
Extend Your Existing Chain
Then, add these new agents to your existing chain.
chain = LLMChain(agents=[qa_agent, small_talk_agent, sentiment_agent, translation_agent])
Execute The New Chain
- Sentiment Analysis
sentiment_response = chain.execute({"text": "I am so happy today!"})
print(sentiment_response)
# Output: {'sentiment': 'positive'}
2. Language Translation (English to Spanish)
translation_response = chain.execute({"text": "Hello, how are you?"})
print(translation_response)
# Output: {'translation': 'Hola, ¿cómo estás?'}
Combining Multiple Agents for a More Robust Chatbot
Here’s how you can combine different functionalities to create a more versatile chatbot that reacts to the sentiment of a user:
user_input = "Tell me a joke!"
small_talk_response = chain.execute({"text": user_input})
joke = small_talk_response['response']
sentiment_response = chain.execute({"text": joke})
user_sentiment = sentiment_response['sentiment']
if user_sentiment == 'positive':
print(f"Chatbot: {joke}")
else:
print("Chatbot: I apologize for the earlier joke. How can I assist you further?")
More Programming Use Cases
Langchain can also assist you in coding more efficiently and easily.
SQL Database Operations
For instance, you can even write an agent to perform SQL queries and return the result:
sql_query_template = PromptTemplate("Execute SQL Query: SELECT * FROM {table}")
sql_query_agent = Agent(prompt_template=sql_query_template, llm=chatgpt_llm)
Then, to execute this agent, add it to your chain and execute it:
chain = LLMChain(agents=[qa_agent, small_talk_agent, sql_query_agent])
sql_response = chain.execute({"table": "users"})
print(sql_response)
# Output: {'result': [...]}
Code Writing
LangChain can dynamically write code snippets for you:
code_template = PromptTemplate("Write Python code to: {task}")
code_agent = Agent(prompt_template=code_template, llm=colossal_llm)
For example, to generate code for a simple “Hello, World!” application:
chain = LLMChain(agents=[qa_agent, small_talk_agent, code_agent])
code_response = chain.execute({"task": "print Hello, World!"})
print(code_response) # Output: {'code': 'print("Hello, World!")'}
Pretty cool, right? Wait till you find out you can even combine its SQL and code writing capabilities!
Combining SQL and Code Writing
Imagine you want to generate a Python code snippet that performs a SQL query. You can achieve this by chaining the agents:
code_sql_response = chain.execute({"task": "perform SQL query", "table": "users"})
print(code_sql_response) # Output: {'code': '...', 'result': [...]}
The above code is just a template since you would have to provide the database details to get an output. By combining these agents, you create a chatbot that’s not only versatile in handling textual tasks but also capable of interacting with databases and generating code on the fly.
I still have an itch for creating my agent, what do I do? Well…
Code Customization
LangChain’s architecture is designed for customization. Beyond the basic agents and LLMs, you can also create your own agents to perform highly specialized tasks. For instance, let’s create a custom agent that filters out profanity from text messages.
from langchain import Agent
class ProfanityFilterAgent(Agent):
def process(self, data):
text = data.get('text', '')
clean_text = text.replace('badword', '****') # Replace 'badword' with stars and remember to write the profanity you want to filter here
return {'clean_text': clean_text}
# Add your custom agent to a chain
chain = LLMChain(agents=[ProfanityFilterAgent(), qa_agent])
response = chain.execute({'text': 'This is a badword example.'})
print(response)
Leveraging LangChain for Diverse Use Cases
Before we dive in, let’s set the stage: LangChain isn’t just another tool in your tech stack—it’s a game-changer. From chatbots to data analytics, we’ll explore and add onto what we have discussed in regards to how this versatile platform can be the answer to a wide array of use cases.
Chatbots
LangChain enhances chatbot functionalities by enabling advanced natural language understanding. With LangChain’s ability to structure and understand chat messages using schema definitions, you can more effectively map user input to actions, thus reducing the chances of miscommunication.
from langchain import OpenAI, ChatPromptTemplate, HumanMessagePromptTemplate
llm = OpenAI(temperature=0.2, openai_api_key=openai_api_key)
prompt = ChatPromptTemplate(
messages=[
HumanMessagePromptTemplate.from_template("User is asking for the availability of {product_name}.")
],
input_variables=["product_name"]
)
availability_query = prompt.format_prompt(product_name="Laptop Model X")
response = llm.run(availability_query)
print("Chatbot:", response)
Question Answering
LangChain’s power extends to complex question-answering scenarios, as we touched on above, like customer support, academic tutoring, and virtual assistant technology. The platform allows for the easy inclusion of retrieval-based question answering, where it can fetch the most appropriate answer from a database or a set of documents.
LangChain simplifies the integration process, making it possible to have robust Q&A systems without complex configurations.
from langchain import OpenAI, RetrievalQA
llm = OpenAI(temperature=0, openai_api_key=openai_api_key)
qa = RetrievalQA.from_chain_type(llm=llm, chain_type="qa", retriever=some_retriever_instance)
query = "What is the capital of Germany?"
answer = qa.run(query)
print("Answer:", answer)
Summarization
In an information-heavy world, summarization becomes a useful tool to distill long articles, reports, or conversations into short, manageable readouts. LangChain allows for dynamic summarization tasks to be performed easily, offering concise summaries generated through advanced NLP algorithms. You can even fine-tune the level of summarization to suit your specific needs.
from langchain import OpenAI
llm = OpenAI(temperature=0, openai_api_key=openai_api_key)
summary_query = "Summarize the following text: ..."
summary = llm.run(summary_query)
print("Summary:", summary)
Text Generation
LangChain allows for controlled text generation through its integrated models. Whether you’re generating product descriptions, headlines, or even automated news reports, LangChain’s ability to handle structured prompts can guide the text generation in the direction you want.
from langchain import OpenAI
llm = OpenAI(temperature=0.7, openai_api_key=openai_api_key)
text_gen_query = "Generate a product description for a futuristic smartwatch."
generated_text = llm.run(text_gen_query)
print("Generated Text:", generated_text)
Creative Writing
Creative writing often requires inspiration, brainstorming, and iteration. LangChain can serve as a virtual writing assistant that suggests dialogues, scenes, or entire narrative arcs. So, its advantage over other text generation tools is its ability to understand complex, user-defined prompts and schemas, offering more targeted and contextually appropriate suggestions.
from langchain import OpenAI
llm = OpenAI(temperature=0.8, openai_api_key=openai_api_key)
creative_query = "Write a dialogue between a detective and a suspect."
creative_text = llm.run(creative_query)
print("Creative Text:", creative_text)
Data Analysis
Data analysis often involves SQL queries, data transformations, and statistical calculations. LangChain can automate these steps, transforming natural language queries into executable SQL or Pandas code. Hence, this is particularly useful for business analysts and other non-technical users, allowing them to perform complex data manipulations without the need for coding skills.
from langchain import SQLDatabase, SQLDatabaseChain
sqlite_db_path = 'data/my_data.db'
db = SQLDatabase.from_uri(f"sqlite:///{sqlite_db_path}")
db_chain = SQLDatabaseChain(llm=llm, database=db)
data_analysis_query = "Calculate the average age of users in the Users table."
data_analysis_result = db_chain.run(data_analysis_query)
print("Data Analysis Result:", data_analysis_result)
PDF Interaction
Manual extraction of specific data from PDFs can be extremely time-consuming, especially for large sets of documents. LangChain can be paired with a PDF processing library to read, extract, and even modify PDF content using natural language queries. As a result, this could be incredibly useful for professionals in law, healthcare, or academia who often need to sift through large volumes of textual data.
from langchain import OpenAI
from PyPDF2 import PdfFileReader
llm = OpenAI(temperature=0, openai_api_key=openai_api_key)
def read_pdf(file_path):
pdf_reader = PdfFileReader(file_path)
text = ""
for page_num in range(pdf_reader.getNumPages()):
page = pdf_reader.getPage(page_num)
text += page.extract_text()
return text
pdf_text = read_pdf('some_file.pdf')
pdf_query = f"Extract the section about financial summary from the text: {pdf_text}"
pdf_section = llm.run(pdf_query)
print("PDF Section:", pdf_section)
Deploying Your LangChain Model
After discussing its diverse use cases, let’s leverage Gradio and Streamlit’s user-friendly interfaces to deploy the LangChain models. So, whether you’re a seasoned developer or a newbie, these platforms offer code templates to expedite the process. Hence, let’s dive into how you can make your LangChain model accessible to the world in just a few simple steps.
Deployment Using Streamlit Template
Streamlit offers a straightforward way to create web apps with Python. Therefore, it can be used to deploy LangChain models.
# streamlit_app.py
import streamlit as st
from streamlit_chat import message # Assuming you've got a widget or function to manage chat messages
from langchain.chains import ConversationChain
from langchain.llms import OpenAI
def load_chain():
"""Logic for loading the chain you want to use should go here."""
llm = OpenAI(temperature=0)
chain = ConversationChain(llm=llm)
return chain
chain = load_chain()
# StreamLit UI Configurations
st.set_page_config(page_title="LangChain Demo", page_icon=":robot:")
st.header("LangChain Demo")
if "generated" not in st.session_state:
st.session_state["generated"] = []
if "past" not in st.session_state:
st.session_state["past"] = []
def get_text():
input_text = st.text_input("You: ", "Hello, how are you?", key="input")
return input_text
user_input = get_text()
if user_input:
output = chain.run(input=user_input)
st.session_state.past.append(user_input)
st.session_state.generated.append(output)
if st.session_state["generated"]:
for i in range(len(st.session_state["generated"]) - 1, -1, -1):
message(st.session_state["generated"][i], key=str(i))
message(st.session_state["past"][i], is_user=True, key=str(i) + "_user")
Then, to deploy, simply run:
streamlit run streamlit_app.py
Deployment Using Gradio Template
Gradio is another powerful library to turn machine learning models into web apps. It is equally effective for deploying LangChain models.
# gradio_app.py
import os
from typing import Optional, Tuple
import gradio as gr
from langchain.chains import ConversationChain
from langchain.llms import OpenAI
from threading import Lock
# Define chain and logic to load it
def load_chain():
llm = OpenAI(temperature=0)
chain = ConversationChain(llm=llm)
return chain
# Set OpenAI API key
def set_openai_api_key(api_key: str):
if api_key:
os.environ["OPENAI_API_KEY"] = api_key
chain = load_chain()
os.environ["OPENAI_API_KEY"] = ""
return chain
class ChatWrapper:
def __init__(self):
self.lock = Lock()
def __call__(self, api_key: str, inp: str, history: Optional[Tuple[str, str]], chain: Optional[ConversationChain]):
self.lock.acquire()
try:
history = history or []
if chain is None:
history.append((inp, "Please paste your OpenAI key to use"))
return history, history
import openai
openai.api_key = api_key
output = chain.run(input=inp)
history.append((inp, output))
except Exception as e:
raise e
finally:
self.lock.release()
return history, history
# Gradio UI configurations
# ... [Your Gradio UI code here]
# Launch Gradio app
block.launch(debug=True)
Challenges and Limitations of LangChain
While LangChain offers a wide array of functionalities and features, it’s important to acknowledge its challenges and limitations.
Data Bias
The Challenge
LangChain relies on machine learning models like ChatGPT and ColossalAI, which are trained on vast datasets that could contain biased information. Hence, this poses the risk of the platform perpetuating harmful stereotypes or generating skewed responses.
Proposed Solution
A two-pronged approach could help mitigate this challenge:
- Post-training Audits: Incorporate tools that audit the behavior of the language models, flagging and correcting outputs that reflect bias.
- User Feedback Loop: Implement a feature where users can report biased or inappropriate behavior, allowing for continuous improvement.
Safety and Security
The Challenge
As LangChain could be used in customer-facing applications, there is a concern about the safety and security of the data it handles, especially if it interacts with databases containing sensitive information.
Proposed Solution
- Data Encryption: All data that LangChain processes should be encrypted both in transit and at rest.
- Role-based Access Control (RBAC): Implement RBAC features to limit who can deploy or interact with LangChain instances, particularly in contexts where sensitive data is involved.
Scalability
The Challenge
As the adoption of LangChain grows, scalability could become a concern. Handling a high volume of requests in real-time may present a bottleneck, affecting the speed and performance of the service.
Proposed Solution
- Load Balancing: Distribute incoming queries across multiple instances of LangChain to ensure that no single instance becomes a bottleneck.
- Caching: Implement caching mechanisms to store frequently asked questions and their corresponding answers, thereby reducing the load on the LLM.
Performance
LangChain is not just about ease of use; it’s also built for performance. Because of that, here are some key points that highlight LangChain’s performance efficiency:
- Low Latency: Its low latency is critical for applications requiring real-time responses, like chatbots.
- High Accuracy: This level of accuracy is particularly beneficial for tasks like sentiment analysis, language translation, and question-answering.
- High Scalability: Built with scalability in mind, LangChain is designed to grow with your needs.
Future of LangChain
What does the future of LangChain hold? Let’s find out!
Potential Applications
- Healthcare: LangChain could be used to develop advanced chatbots capable of providing medical information, scheduling appointments, or even analyzing medical records.
- Education: It could serve as a real-time tutor, answering questions and providing code examples for students learning programming or other technical skills.
- E-commerce: Beyond customer service, it could assist in product recommendations based on natural language queries, enhancing the shopping experience.
Research Directions
- Multi-modal Interaction: Research could focus on enabling LangChain to handle more than just text, such as voice or images, to create more interactive and dynamic experiences.
- Real-time Adaptation: Exploring how LangChain can adapt in real-time to different user behaviors or needs could make it even more useful.
- Explainability: Ensuring that the language model’s decision-making process can be understood by users, particularly in sensitive or critical applications.
By addressing its limitations and continuing to innovate, LangChain has the potential to significantly impact various sectors and become a go-to solution for natural language understanding and generation tasks.
Conclusion: Color Me LLM
In this article, we’ve explored LangChain as a powerful framework for building language models into coherent chains for specialized tasks. Whether you’re interested in developing conversational agents, data analytics tools, or complex applications requiring multiple language models, LangChain provides an effective and efficient way to achieve your objectives.
Finally, we’ve walked you through the entire process, from the initial setup and basic usage to more advanced features like SQL query execution and dynamic code writing. Moreover, as natural language processing continues to evolve, LangChain offers a scalable, forward-thinking solution that can adapt to your project’s growing needs.
Thank you for reading, and we encourage you to start chaining your language models to solve real-world problems effectively. Also, if you learned something new in this article, let me know below.
Similar articles: LLaMA and ChatGPT and ChatGPT AI.
Written by: Syed Umar Bukhari.