Algorithms in GoLang: Defining Steps to Solve Problems
Welcome, fellow tech enthusiasts! Today, we’re diving into the fascinating world of algorithms, focusing specifically on how to define and implement them in GoLang, popularly known as Go. Whether you’re a seasoned programmer or just getting started, understanding algorithms is crucial for solving problems efficiently and effectively. Let’s get started!
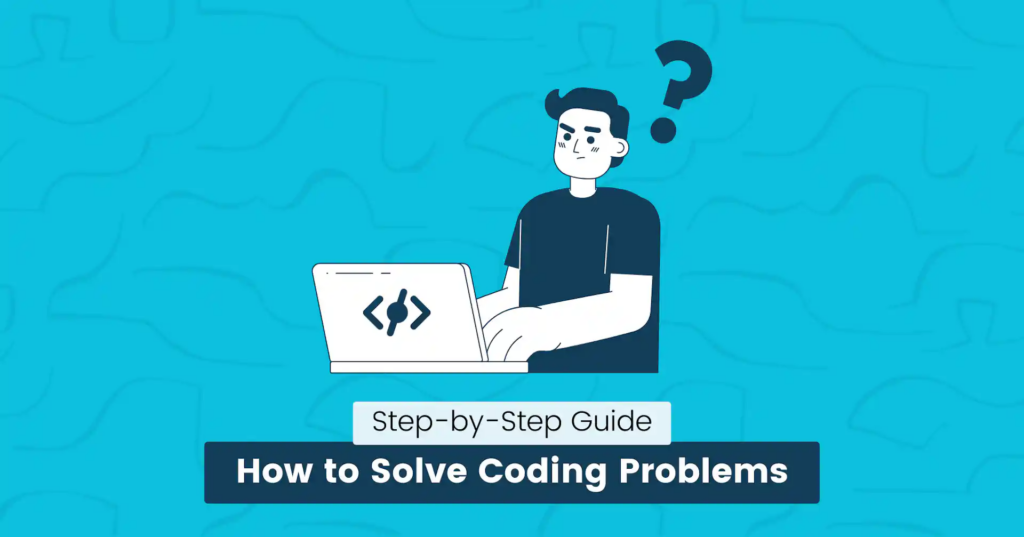
What is an Algorithm?
An algorithm is a step-by-step procedure or formula for solving a problem. It consists of a finite set of instructions, designed to perform a specific task. In essence, algorithms are the building blocks of computer programs.
Why GoLang?
GoLang, created by Google, is renowned for its simplicity, efficiency, and performance. Its concurrency model, garbage collection, and robust standard library make it an excellent choice for implementing algorithms. If you’re new to Go, you might want to check out the Go documentation for a solid introduction.
Understanding the Problem
Before jumping into code, let’s outline the problem we want to solve with our algorithm. For this example, we’ll solve the classic problem of finding the greatest common divisor (GCD) of two numbers. The GCD of two integers is the largest integer that divides both numbers without leaving a remainder.
Euclidean Algorithm
The Euclidean algorithm is a great method for finding the GCD of two numbers. Here’s how it works:
- If both numbers are zero, the GCD is undefined.
- If one number is zero, the GCD is the other non-zero number.
- Otherwise, continuously replace the larger number by the remainder when the larger number is divided by the smaller number until one of the numbers becomes zero.
Let’s see how to implement this in GoLang.
Implementing the Euclidean Algorithm in GoLang
First, we’ll define a function to calculate the GCD using the Euclidean algorithm:
package main
import (
"fmt"
)
func gcd(a, b int) int {
if a == 0 {
return b
} else if b == 0 {
return a
} else {
for b != 0 {
a, b = b, a%b
}
return a
}
}
func main() {
num1 := 48
num2 := 18
fmt.Printf("The GCD of %d and %d is %d\n", num1, num2, gcd(num1, num2))
}
Here’s a breakdown of the code:
- We define a function
gcd(a, b int) int
that takes two integers and returns an integer. - We start by handling the base cases where either
a
orb
is zero. - If neither number is zero, we use a loop to replace the larger number by the remainder of the division until one of the numbers becomes zero.
- Finally, we call this function in the
main()
function with example values and print the result.
Key Points
- In each iteration, the pair
(a, b)
is updated to(b, a % b)
. - Initially,
a
is the greater number. - The role of
a
andb
keeps switching in the loop, but the key observation is that the value ofa
always gets the value ofb
from the previous iteration, andb
gets the remainder (a % b
). - When
b
becomes0
,a
holds the GCD.
Thus, the greater number (or a number that quickly reduces the value of b
to zero) becomes the starting point of each iteration and dictates the process of finding the GCD.
Improving Algorithm Performance
While the above implementation is correct, we can make it more efficient by using recursion. Recursion often makes the implementation cleaner and easier to understand:
package main
import (
"fmt"
)
func gcd(a, b int) int {
if b == 0 {
return a
}
return gcd(b, a % b)
}
func main() {
num1 := 48
num2 := 18
fmt.Printf("The GCD of %d and %d is %d\n", num1, num2, gcd(num1, num2))
}
In the recursive approach, the logic is more intuitive. We keep calling the gcd
function with the remainder until we reach the base case where b
is zero.
Applications of Algorithms
Algorithms are not limited to mathematical computations. They are extensively used in various fields such as:
- Data Science: For analyzing and building predictive models.
- Software Development: To optimize code and improve performance.
- Machine Learning: For creating models that can learn and make decisions.
- Cybersecurity: In cryptographic security and threat detection.
Learning Resources
If you’re eager to delve deeper into algorithms and GoLang, here are some fantastic resources:
- GoLang Tour: An interactive journey through Go’s capabilities.
- GeeksforGeeks on Euclidean Algorithm: A detailed explanation of the Euclidean algorithm.
- Coursera Algorithms Specialization: A comprehensive course on algorithms.
Conclusion
Understanding and implementing algorithms is a pivotal skill for any programmer. With GoLang’s efficiency and ease of use, you can craft optimized solutions for a variety of problems. So, keep experimenting, keep learning, and happy coding!
Got questions, or want to share your own implementations? Drop a comment below, and let’s discuss the incredible world of algorithms together. Stay curious and innovative!