Greedy vs. Dynamic Programming in Python for Coin Change Problem
Hey there, Pythonistas! Today, we’re diving deep into the world of algorithms with the classic Coin Change Problem. You know, that pesky little problem where you’re given a set of coin denominations and need to determine the minimum number of coins needed to make a given amount. Sounds simple, right? Well, it’s one of those deceptively tricky problems, like trying to keep a cat in a bath. So, let’s roll up our sleeves and get into the meat and potatoes (or circuits and code, in our case) of Greedy and Dynamic Programming approaches to solve this problem!
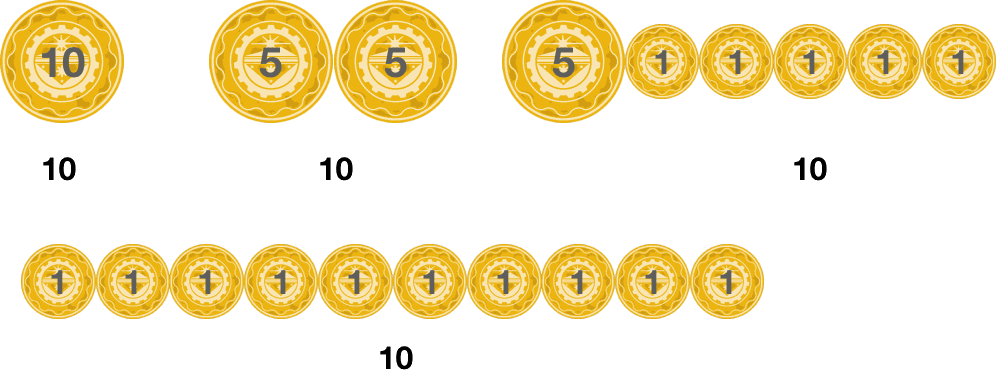
Understanding the Coin Change Problem
Before we get too deep into the algorithmic jungle, let’s briefly go over what the Coin Change Problem is. Given a total amount V
and an array of denominations coins[]
, the problem is to find the minimum number of coins needed to make the amount V
. Sounds easy peasy lemon squeezy, right? Until you realize that there are multiple ways to approach it.
Greedy Algorithm
The Greedy algorithm is like that friend who always goes for the biggest slice of pizza first. It’s simple, quick, and sometimes effective. In the case of the Coin Change Problem, the greedy approach aims to pick the largest denomination coin first and then proceed with the remaining amount.
Python Implementation of Greedy Algorithm
Let’s jump right into the code:
def greedy_coin_change(coins, amount):
# Sort coins to start with the largest denomination
coins.sort(reverse=True)
num_coins = 0
for coin in coins:
while amount >= coin:
amount -= coin
num_coins += 1
if amount != 0:
return -1 # If amount is not zero, it means change wasn't possible with given coins
return num_coins
# Example Usage
coins = [1, 2, 5, 10, 20, 50]
amount = 93
print(f"Greedy solution: {greedy_coin_change(coins, amount)} coins")
Now, before you throw your hats in the air celebrating, not so fast! The greedy solution works only when specific conditions are met, such as the denominations are multiple of each other. If not, it could fail spectacularly, like trying to make a purchase with only large bills at a coin-only vending machine. For more on why greedy algorithms might not always work, check out this deep dive on greedy algorithms.
Dynamic Programming
Enter the hero of our story: Dynamic Programming (DP). This approach is like the Sherlock Holmes of algorithms—methodical, thorough, and always gets the job done. The idea is to solve the problem by solving subproblems and building up to the final solution. We’ll use a table to store the minimum number of coins needed for each amount up to V
.
Python Implementation of Dynamic Programming Approach
Here’s how to implement the DP solution:
def dynamic_coin_change(coins, amount):
# Initialize the DP table with a value greater than any possible solution (amount + 1)
dp = [amount + 1] * (amount + 1)
# Base case: No coin needed to make the amount 0
dp[0] = 0
for current_amount in range(1, amount + 1):
for coin in coins:
if coin <= current_amount:
dp[current_amount] = min(dp[current_amount], dp[current_amount - coin] + 1)
# If dp[amount] has not been updated, return -1 indicating no solution
return dp[amount] if dp[amount] != amount + 1 else -1
# Example Usage
coins = [1, 2, 5, 10, 20, 50]
amount = 93
print(f"DP solution: {dynamic_coin_change(coins, amount)} coins")
Ah, the beauty of dynamic programming! It ensures that all subproblems are tackled and the optimal solution is reached. Of course, it takes more memory than our greedy friend, but it’s rock-solid in terms of correctness. For a deeper dive into dynamic programming, you might want to read this fantastic guide on our previous posts (Part I, Part II).
Greedy vs. Dynamic Programming: The Showdown!
Alright, so we have our contenders in the ring: Greedy vs. DP. Who comes out on top? Let’s sum it up:
- Greedy Approach:
- Pros: Quick, simple, and easy to implement.
- Cons: Not always correct, can fail for certain coin denominations.
- Dynamic Programming Approach:
- Pros: Always finds the optimal solution, reliable.
- Cons: More complex, uses more memory.
In most real-world scenarios, you’ll likely want to go with dynamic programming to ensure you get the correct result every time. However, if you’re dealing with denominations that are multiples of each other, the greedy approach is a quick and dirty solution that can save you some time.
Wrapping Up
And there you have it, folks! Greedy vs. Dynamic Programming in the world of the Coin Change Problem. Whether you need a quick solution in a pinch or a rock-solid method that handles any situation, you now have the tools to make it rain (with coins, of course). Until next time, keep coding and keep smiling!