Control Structures in GoLang: Directing Program Flow
Programing languages allow us to direct a software program to perform specific tasks based on given conditions. This task is done using control structures, which are vital components in any coding language. Right now, we are about to take a thrilling ride on the roller-coaster of GoLang control structures. So buckle up, it’s going to be a ride full of loops and conditions!
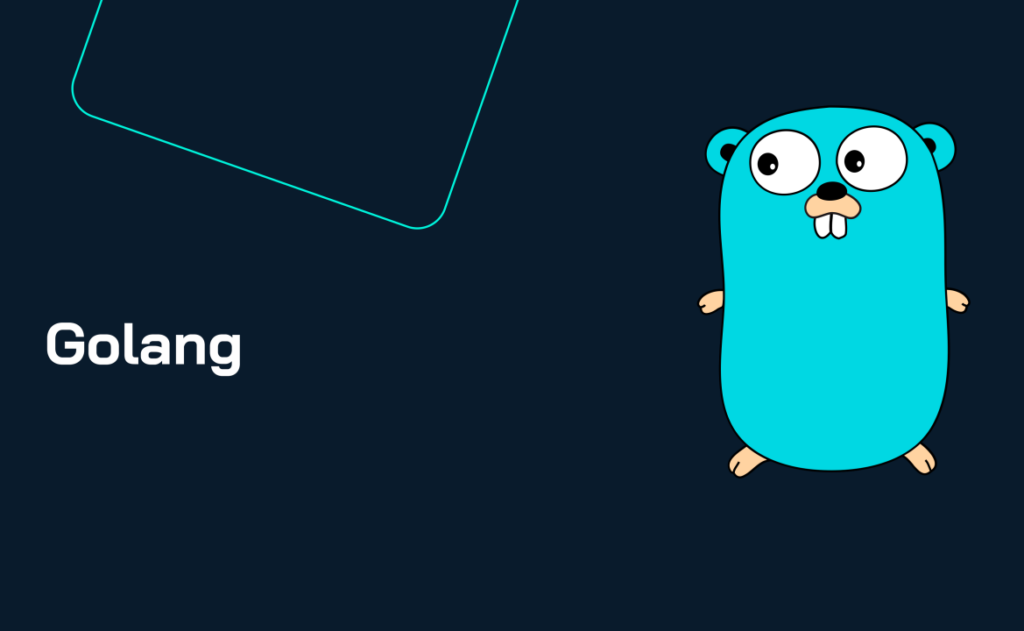
What are Control Structures?
To fully appreciate control structures in GoLang, let us clarify what control structures really are. Control structures direct the flow of a program. They help your program to make decisions based on different conditions. Imagine your program as a tourist in an unusual city. Control structures are like road signs pointing them where to go and what to do next. If they encounter a “U-turn” sign, they’ll promptly make a U-turn back to where they started; that’s a loop right there!
Types of Control Structures in GoLang
There are basically three types of control structures in Go Programming Language: Real structures, Iterative structures, and decision-making structures. Let’s delve into them one by one. They might seem complicated at first, but don’t worry, we’ll tackle each with a hands-on approach.
if-else Control Structure
Let’s say you want to know if a number is even or odd. You put the number in your program and it tells you if it’s odd or even. This is where the if-else comes into play in GoLang, allowing you to code based on different conditions. Below is a simplistic implementation:
package main
import "fmt"
func main() {
num := 10
if num % 2 == 0 {
fmt.Print("Even")
} else {
fmt.Print("Odd")
}
}
In the snippet above, we used the if-else control structure to determine if a number is even or odd. If the modulus of the number divided by 2 equals 0, that means it’s an even number and the program prints “Even,” otherwise, it prints “Odd.” Easy right?
For Loop Control Structure
Now consider you have a series of tasks that need to be performed over and over again. Say for instance, you want to generate the first ten numbers in the Fibonacci series. You can leverage the for loop control structure in GoLang to implement this task. Here is a simple code example showcasing this.
package main
import "fmt"
func main() {
a, b := 0, 1
for i := 0; i < 10; i++ {
fmt.Print(a, " ")
a, b = b, a+b
}
}
Switch Control Structure
Deciding what to eat every day can be a confusing task. The switch control structure in GoLang, however, can make this decision for you, and I promise, it won’t pick broccoli every time. Have a look at the code snippet below to see how this can be done cleverly.
package main
import (
"fmt"
"math/rand"
"time"
)
func main() {
rand.Seed(time.Now().UnixNano())
choice := rand.Intn(3) + 1
switch choice {
case 1:
fmt.Print("Pizza")
case 2:
fmt.Print("Pasta")
case 3:
fmt.Print("Broccoli...")
default:
fmt.Print("Water")
}
}
Is it just me, or do you also hear Johann Strauss’s Radetzky March playing in the background?
Installation Instructions for Different Operating Systems in GoLang
To run the above pieces of code, you first need to install GoLang in your system. Instructions vary with different operating systems, but don’t fret; I have provided installation guidelines below for three major operating systems:
1. Windows: Download the MSI installer package from here and follow the prompts.
2. MacOS: You can install GoLang via Homebrew using the command `brew install go`.
3. Linux: Use the following command `sudo apt install golang-go` for Ubuntu, or `sudo yum install golang` for Fedora.
Remember, Google is your best friend when you encounter any setup problems.
Conclusion
Control structures in GoLang can seem daunting, but with the detailed instruction given in this post, you are well on your way to directing your program flow more efficiently. Remember, practice makes perfect. You will encounter difficulties at first, but with continuous coding in GoLang, mastering control structures in GoLang will soon be a walk in the park…or code!
Practice using the samples in this post, because after all, you know what they say: coding is the art of telling another human what should be done by a computer – that’s why it is so hard to debug! But keep practicing, and you will master it all.