Unlocking the Power of Data Structures in Python: Organizing and Storing Data Efficiently
Welcome back, tech enthusiasts! Today, we’re diving into the fascinating world of data structures in Python. If you’re looking to elevate your programming game and master the art of organizing and storing data efficiently, you’re in the right place.
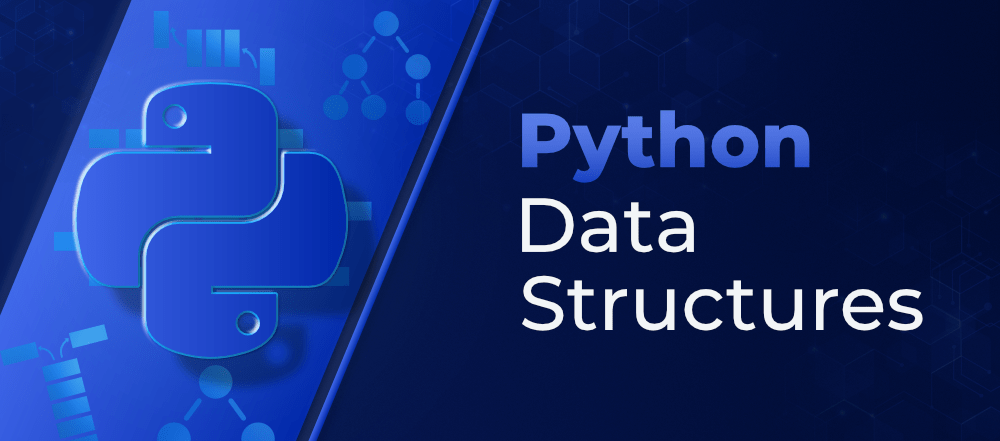
Why Data Structures Matter
Data structures are essential for efficient data management and algorithm performance. Whether you’re building a simple application or a complex system, understanding data structures can significantly impact your code’s efficiency and readability.
The Basics: Lists
Lists in Python are perhaps the most commonly used data structure. They are dynamic arrays that can hold a collection of items, allowing you to handle and manipulate related data seamlessly.
# Creating a list in Python
fruits = ['apple', 'banana', 'cherry']
print(fruits)
Lists are versatile, supporting various data types and providing methods for appending, removing, and sorting elements.
Advanced Collections: Tuples
Tuples are similar to lists but with a key difference: they are immutable. Once defined, the elements in a tuple cannot be changed. This characteristic makes tuples ideal for data that should remain constant.
# Creating a tuple in Python
coordinates = (10, 20)
print(coordinates)
Use tuples when you need to ensure data integrity and avoid accidental modifications.
Efficient Data Retrieval: Dictionaries
Dictionaries are unordered collections of key-value pairs. They provide a fast and efficient way to retrieve data based on a unique key.
# Creating a dictionary in Python
student = {
'name': 'John',
'age': 21,
'courses': ['Math', 'Science']
}
print(student)
Dictionaries offer quick lookups, making them ideal for scenarios where you need to store and retrieve data based on unique identifiers.
Managing Ordered Data: Sets
Sets are collections of unique elements, meaning no duplicates are allowed. They are particularly useful for membership testing and eliminating duplicate entries.
# Creating a set in Python
numbers = {1, 2, 3, 4, 4, 5}
print(numbers)
Notice in the example above that the duplicate ‘4’ is automatically removed from the set.
Combining Data Structures
One of Python’s strengths is its ability to combine different data structures. For example, you can have a list of dictionaries or a dictionary of lists.
# List of dictionaries
students = [
{'name': 'John', 'age': 21},
{'name': 'Jane', 'age': 22}
]
print(students)
# Dictionary of lists
courses = {
'Math': [70, 80, 90],
'Science': [85, 90, 95]
}
print(courses)
This flexibility allows you to create complex data models and structures suited to your specific needs.
When to Use Each Structure
- Lists: Use lists for ordered collections of elements where you may need to add or remove items frequently.
- Tuples: Opt for tuples when you need an immutable sequence of elements.
- Dictionaries: Choose dictionaries for key-value pair mappings with fast lookups.
- Sets: Use sets to store unique elements and perform membership tests.
Performance Considerations
Each data structure has its own performance characteristics. Understanding these can help you make better choices for your specific use cases. For example, list lookups are O(n), whereas dictionary lookups are O(1) due to hash table implementation.
For more detailed performance analysis, check out this comprehensive guide on Python’s time complexity.
Conclusion: Embrace the Power of Data Structures
By mastering Python’s data structures, you can write more efficient, readable, and maintainable code. Whether you’re building simple scripts or complex systems, choosing the right data structure can make all the difference.
Don’t stop hereākeep exploring, experimenting, and pushing the boundaries of what’s possible with Python. The world of technology waits for no one, but with the right knowledge, you’re always ahead of the curve!
Hungry for more? Stay tuned for our next post where we’ll dive deeper into advanced data structures and their real-world applications. Until then, happy coding!