Unraveling Dynamic Programming in Python: Fibonacci Sequence Solutions
Hello, tech enthusiasts! If you’re a fan of squeezing efficiency out of your code like juice from a lemon, you’ve landed at the right place! Today, we’re diving into the wonderful world of Dynamic Programming (DP) in Python, with the Fibonacci sequence as our star guest. Buckle up, because by the end of this post, you’ll be a DP dynamo!
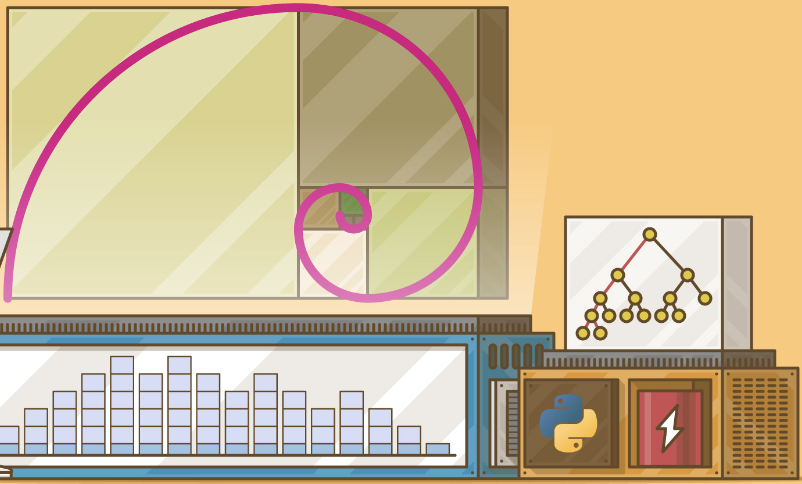
What is Dynamic Programming?
Dynamic Programming is a method for solving complex problems by breaking them down into smaller subproblems. It is particularly powerful when these subproblems overlap, meaning the same subproblems are solved multiple times. Instead of solving the same problem again and again, DP stores the results of subproblems in a table and reuses them whenever needed. This technique drastically reduces computation time and increases efficiency.
The Fibonacci Sequence – A Perfect Candidate for DP
The Fibonacci sequence, where each number is the sum of the two preceding ones, is a classic example of an overlapping subproblem situation. The sequence starts with 0 and 1, followed by 1, 2, 3, 5, 8, and so on. Let’s see why DP fits like a glove here.
The Naive Recursive Approach
Let’s start with the naive recursive solution to understand why we need DP. Here’s the code:
def fib_recursive(n):
if n <= 1:
return n
return fib_recursive(n-1) + fib_recursive(n-2)
print(fib_recursive(10)) # Output: 55
While this code is elegant and simple, it’s highly inefficient for large values of `n` due to its exponential time complexity of O(2^n). Each call to `fib_recursive` makes two more calls, resulting in a massive number of redundant calculations. Yikes!
Memoization to the Rescue!
Memoization is a top-down approach where we store the results of expensive function calls and reuse them when the same inputs occur again. Let’s see how memoization can be implemented to optimize our Fibonacci function:
def fib_memoization(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fib_memoization(n-1, memo) + fib_memoization(n-2, memo)
return memo[n]
print(fib_memoization(10)) # Output: 55
By using a dictionary to store previously computed values, we’ve transformed our function from exponential to linear time complexity, O(n). Great, but can we do even better? Yes, we can!
Iterative Bottom-Up DP
The iterative approach, also known as bottom-up DP, builds the solution from the ground up. No recursion, just iteration. Here’s how it looks:
def fib_iterative(n):
if n <= 1:
return n
fib = [0] * (n + 1)
fib[1] = 1
for i in range(2, n + 1):
fib[i] = fib[i-1] + fib[i-2]
return fib[n]
print(fib_iterative(10)) # Output: 55
In this implementation, we use a list to store Fibonacci numbers until the `n`th number. Consequently, the space complexity is O(n). While efficient, it’s still possible to save more space.
Space Optimized Iterative DP
We can optimize the space complexity further since we only need the last two Fibonacci numbers at any point in our calculation. Here’s the space-optimized version:
def fib_optimized(n):
if n <= 1:
return n
a, b = 0, 1
for _ in range(2, n + 1):
a, b = b, a + b
return b
print(fib_optimized(10)) # Output: 55
Voila! With our optimized approach, we’ve reduced both time and space complexities to O(n) and O(1), respectively. Efficiency never looked so good!
Conclusion
Dynamic Programming isn’t just a fancy buzzword—it’s a powerful tool that can drastically improve the performance of your code. By leveraging memoization or iterative techniques, you can make quick work of seemingly complex problems like the Fibonacci sequence.
Feeling inspired to dive deeper into the world of Dynamic Programming? Check out this [comprehensive guide](https://www.geeksforgeeks.org/dynamic-programming/) for more tips and tricks.
Remember, the key to mastering DP (or any programming concept) lies in practice. So roll up your sleeves, fire up that IDE, and start optimizing! Happy coding!
Be sure to subscribe for more tech-tastic insights, and until next time, keep coding and keep smiling!