Error Handling in Python: Managing Runtime Errors
Python is a fantastic programming language that combines simplicity and power. However, even the most robust Python code can encounter runtime errors. Good error handling practices are crucial to creating applications that are both user-friendly and maintainable. In this post, we’ll explore various techniques to manage runtime errors in Python, making your programs more resilient and your code more clean.
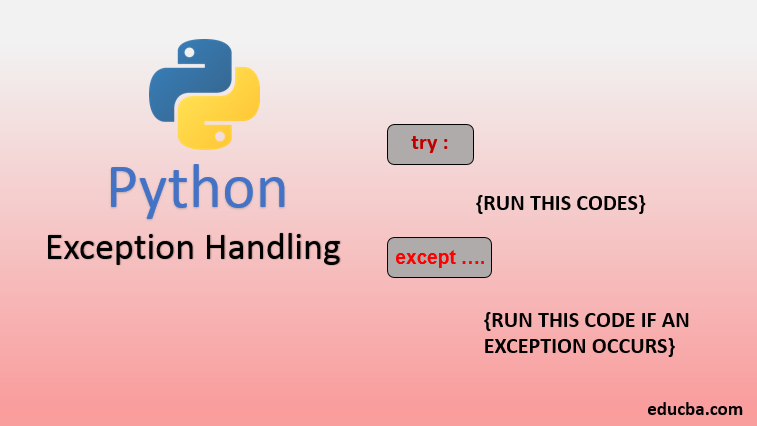
Understanding Runtime Errors
Runtime errors, also termed exceptions, occur during the execution of a program. Unlike syntax errors, which are detected during the code compilation stage, runtime errors are typically the result of unforeseen conditions during the program’s runtime. Common examples include division by zero, file not found errors, and type mismatches.
The Basics of Exception Handling
Python provides a robust way to handle exceptions through the try-except block. This structure allows you to attempt to execute code (in the try
block) and catch any exceptions that occur (in the except
block). Here’s a simple example:
try:
result = 10 / 0
except ZeroDivisionError:
print("You can't divide by zero!")
In the code above, attempting to divide by zero raises a ZeroDivisionError
, which is then caught by the except
block, displaying an appropriate message. This prevents the program from crashing and allows it to handle the error gracefully.
Handling Multiple Exceptions
Sometimes, you might need to handle more than one type of exception. Python allows you to manage multiple exceptions using different except blocks:
try:
value = int(input("Enter a number: "))
result = 10 / value
except ZeroDivisionError:
print("You can't divide by zero!")
except ValueError:
print("Invalid input! Please enter a valid number.")
This approach ensures that specific errors are caught and handled appropriately, providing more informative feedback to the user.
Using the Else Clause
The else
clause in a try-except block is executed only if no exceptions are raised in the try block. It allows you to cleanly separate your exception handling code from the main logic:
try:
value = int(input("Enter a number: "))
result = 10 / value
except ZeroDivisionError:
print("You can't divide by zero!")
except ValueError:
print("Invalid input! Please enter a valid number.")
else:
print(f"The result is {result}")
Here, the else
block executes only if no exception occurred, making the logic of your code clearer and easier to manage.
The Finally Block
The finally
block is executed no matter what, whether an exception occurred or not. This is useful for cleaning up resources, such as closing files or network connections:
try:
file = open('example.txt', 'r')
content = file.read()
except FileNotFoundError:
print("The file was not found!")
finally:
file.close()
print("File has been closed.")
The finally
block ensures that the file is always closed, regardless of whether the file was successfully read or not.
Custom Exception Handling
In addition to built-in exceptions, you can also define your own custom exceptions. This is useful for specific errors that need special handling. You do this by subclassing the Exception
class:
class CustomError(Exception):
pass
try:
raise CustomError("This is a custom error message")
except CustomError as e:
print(e)
Defining custom exceptions allows you to tailor error handling specifically to the needs of your application, providing greater control over your program’s behavior.
Best Practices for Error Handling
To effectively manage runtime errors in Python, consider the following best practices:
- Be Specific: Catch specific exceptions rather than using a generic except clause. This helps in understanding and debugging errors.
- Keep it Simple: Avoid overly complex try-except blocks. Simple, clear error handling makes your code easier to understand and maintain.
- Inform the User: Provide informative error messages to the user. This enhances user experience and helps in diagnosing issues.
- Log Errors: Use logging to record exceptions. This is particularly useful in production environments for tracking down issues.
For a more in-depth exploration of these practices, you can check out this excellent resource from the Real Python website.
Conclusion
Effective error handling is essential for writing robust and reliable Python applications. By employing try-except blocks, handling multiple exceptions, and using else and finally clauses, you can create programs that are more resilient and user-friendly. Moreover, incorporating custom exceptions and following best practices will make your code cleaner and more maintainable.
So throw away the fear of exceptions and embrace these techniques in your programming toolbox. Happy coding!