Functions in Python: Encapsulating Code for Reuse
Hey there, tech enthusiasts! Today, we’re diving into one of the fundamental concepts of programming: functions in Python. If you’re ready to level up your coding skills, stick around to learn how functions can help you encapsulate code for reuse, making your projects cleaner, more efficient, and infinitely cooler!
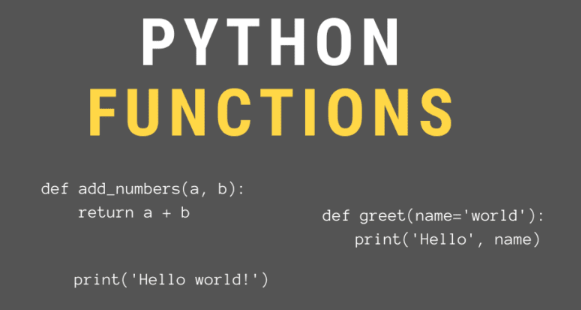
What is a Function?
A function is a block of reusable code designed to perform a single, related action. Functions provide better modularity for your application and a high degree of code reusability. A function can take input, do some processing, and then produce output. It’s like a well-oiled machine that takes ingredients, does some magic, and then provides an output.
Why Use Functions?
Using functions in Python (or any programming language) has countless benefits. Here are some of the key advantages:
- Code Reusability: Write a function once, and use it many times.
- Modularity: Divide your code into useful blocks that can be managed independently.
- Ease of Testing: Test individual components to ensure each part works correctly.
- Readability: Functions break down complex problems into simple, easy-to-understand pieces.
Defining a Function in Python
Wondering how you can define a function in Python? Let’s dig into some basic syntax and examples. To define a function, use the def
keyword followed by the function name and a pair of parentheses. Inside the parentheses, you can specify your parameters. The function body follows, and it is indented as per Python’s indentation rules.
def function_name(parameters):
"""docstring"""
# function body
return result
Here’s a quick example to make it clearer.
def greet(name):
"""This function greets the person passed in as a parameter"""
print(f"Hello, {name}. Good to see you!")
# Call the function with an argument
greet('Alice')
When you run the above code, it’ll greet Alice with a cheerful message. Simple and effective, right?
Function Parameters and Arguments
Functions can take parameters, which act as placeholders for the values we want to pass in when we call the function. These values (or data) are called arguments when the function is called. Here’s how you can work with them:
def add_numbers(a, b):
"""This function adds two numbers"""
return a + b
# Call the function with arguments
result = add_numbers(5, 3)
print(result) # Output: 8
In the example above, a
and b
are parameters of the add_numbers
function. When we call the function with arguments 5 and 3, the function performs the addition and returns the result.
Default Parameters
Sometimes, you might want to define a function with default parameter values. This allows you to call the function without specifying all arguments. Check this out:
def greet(name="Guest"):
"""This function greets the person passed in or Guest by default"""
print(f"Hello, {name}. Welcome!")
greet() # Output: Hello, Guest. Welcome!
greet('Alice') # Output: Hello, Alice. Welcome!
Here, if no argument is passed to the greet
function, “Guest” will be used as the default value.
Return Statement
The return
statement is used to exit a function and go back to the place from where it was called. It can return a value or a result to the caller. Let’s see an example:
def multiply_numbers(x, y):
"""This function multiplies two numbers and returns the result"""
return x * y
result = multiply_numbers(4, 5)
print(result) # Output: 20
In this case, multiply_numbers
returns the product of x and y, and we print the result, which is 20.
Lambda Functions
A lambda function is a small anonymous function defined with the lambda
keyword. It can have any number of arguments but only one expression. It’s often used for short, throwaway functions. Here’s an example:
add = lambda a, b: a + b
print(add(2, 3)) # Output: 5
In this case, lambda a, b: a + b
is a lambda function that takes two arguments and returns their sum.
Built-in Functions
Python comes with a plethora of built-in functions ready to use. Functions like print()
, len()
, and range()
are all built-in and can be used in any Python script. Check out the full list of built-in functions in Python documentation.
Conclusion
There you have it, folks! Python functions are an essential part of writing clean and efficient code. Whether you are a beginner or a seasoned programmer, mastering functions will undeniably elevate your coding game. Keep coding, keep improving, and remember, a well-organized codebase is a happy codebase!
Are you excited to explore more about Python? Stay tuned for our next post and keep that coding spirit high! ?