Input/Output in GoLang: Interacting with External Data Sources
Welcome to another exciting journey into the world of GoLang, where creativity and efficiency merge to create something magical! Today, we’re diving into the heart of input/output operations (I/O) in GoLang, especially focusing on how to interact with external data sources. Whether you’re reading from files, writing to them, or fetching data from the web, GoLang has got your back! Let’s jump right in and make some GoLang magic happen.
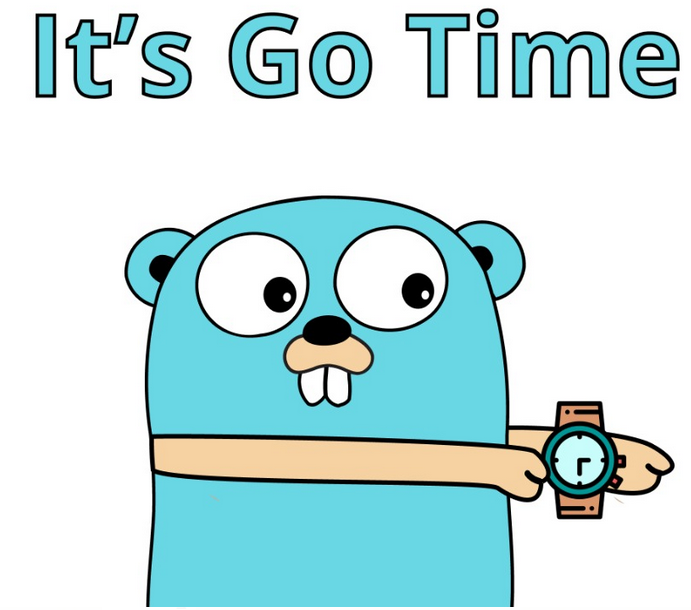
Understanding the Basics of I/O in GoLang
Before we get our hands dirty with code, let’s take a moment to understand what input/output operations mean in GoLang. In simple terms, I/O operations are actions that facilitate communication between your Go program and the external environment, which could be a file, a database, a network service, or even a user.
GoLang provides a rich standard library that simplifies I/O tasks. Here are some of the most commonly used packages:
fmt
: For formatted I/O.os
: For low-level file handling.bufio
: Provides buffered I/O.net/http
: For HTTP client and server implementations.
Armed with this knowledge, let’s dive into some practical examples.
Reading from Files
The beauty of GoLang lies in its simplicity and elegance. Reading a file in Go is straightforward. Here’s a quick example:
package main
import (
"fmt"
"io/ioutil"
"log"
"os"
)
func main() {
file, err := os.Open("example.txt")
if err != nil {
log.Fatal(err)
}
defer file.Close()
contents, err := ioutil.ReadAll(file)
if err != nil {
log.Fatal(err)
}
fmt.Println(string(contents))
}
In this example, we use the os
package to open a file called example.txt
. We then read its contents using the ioutil
package and print them to the console. Simple yet effective!
Writing to Files
Writing to a file in Go is just as simple. Here’s a quick snippet to illustrate that:
package main
import (
"log"
"os"
)
func main() {
file, err := os.Create("output.txt")
if err != nil {
log.Fatal(err)
}
defer file.Close()
_, err = file.WriteString("Hello, GoLang!")
if err != nil {
log.Fatal(err)
}
log.Println("Data written to file successfully")
}
This code creates a new file called output.txt
and writes the string “Hello, GoLang!” into it. Easy peasy!
Interacting with Web APIs
GoLang excels in handling web requests. Using the net/http
package, you can fetch data from APIs seamlessly. Let’s fetch JSON data from a public API.
package main
import (
"encoding/json"
"fmt"
"log"
"net/http"
)
type ApiResponse struct {
UserID int `json:"userId"`
ID int `json:"id"`
Title string `json:"title"`
Completed bool `json:"completed"`
}
func main() {
resp, err := http.Get("https://jsonplaceholder.typicode.com/todos/1")
if err != nil {
log.Fatal(err)
}
defer resp.Body.Close()
var result ApiResponse
err = json.NewDecoder(resp.Body).Decode(&result)
if err != nil {
log.Fatal(err)
}
fmt.Printf("Fetched data: %+v\n", result)
}
In this example, we make a GET request to a public API that returns JSON data, decode the response, and print the formatted result. It’s as easy as 1-2-3!
Buffered I/O with bufio
The bufio
package provides more control over I/O operations by introducing buffering. This can make your I/O operations more efficient.
package main
import (
"bufio"
"fmt"
"log"
"os"
)
func main() {
file, err := os.Open("largefile.txt")
if err != nil {
log.Fatal(err)
}
defer file.Close()
scanner := bufio.NewScanner(file)
for scanner.Scan() {
fmt.Println(scanner.Text())
}
if err := scanner.Err(); err != nil {
log.Fatal(err)
}
}
In this example, we read a large file line by line using a buffered scanner. This approach is more memory-efficient, especially for larger files.
Conclusion
Interacting with external data sources in GoLang is both fun and straightforward. Whether you’re working with files, web APIs, or other data sources, GoLang’s powerful standard library has you covered. Give these examples a try, and you’ll realize just how delightful and efficient GoLang can be.
Stay curious, stay inspired, and keep exploring the limitless possibilities with GoLang. The sky’s the limit, and we’re just getting started!
Thanks for reading, and don’t forget to visit our blog at Sesame Disk Blog for more exciting content. Happy coding!