Sorting Algorithms in Go: QuickSort vs. MergeSort
Hey there, tech enthusiasts! Today, we’re diving into the fascinating world of sorting algorithms, specifically comparing the tried-and-true QuickSort and MergeSort algorithms as implemented in Go. Sorting algorithms are fundamental to computer science and play a crucial role in optimizing the performance of applications. So, let’s get our hands dirty with some code and see which one reigns supreme!
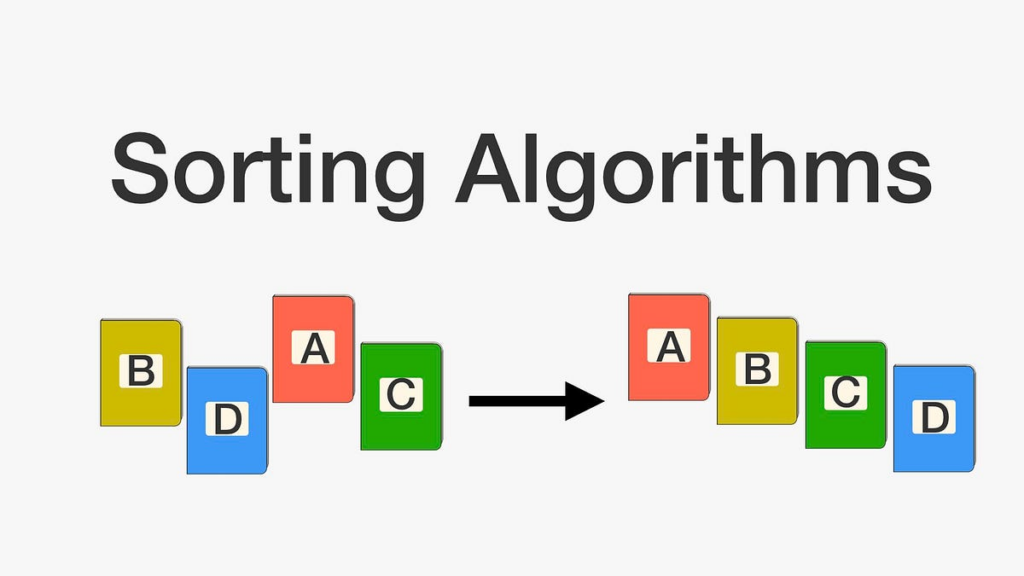
Understanding Sorting Algorithms
Before we jump into code, it’s essential to grasp what sorting algorithms are. Simply put, a sorting algorithm takes an array and rearranges the elements in a specific order, either ascending or descending. Sorting is a vital process because it eases the searching, merging, and extraction of data.
Meet QuickSort
QuickSort, as its name implies, is known for its speed and efficiency in most cases. It follows a divide-and-conquer approach to sort elements. QuickSort selects a ‘pivot’ element from the array and partitions the other elements into two sub-arrays—those less than the pivot and those greater than the pivot. This process is recursively applied to the sub-arrays.
Let’s take a look at how QuickSort is implemented in Go:
package main
import "fmt"
func quickSort(arr []int) []int {
if len(arr) < 2 {
return arr
}
pivot := arr[len(arr)/2]
var left, right []int
for _, v := range arr {
if v < pivot {
left = append(left, v)
} else if v > pivot {
right = append(right, v)
}
}
return append(append(quickSort(left), pivot), quickSort(right)...)
}
func main() {
arr := []int{3, 6, 8, 10, 1, 2, 1}
fmt.Println("Unsorted:", arr)
sorted := quickSort(arr)
fmt.Println("Sorted:", sorted)
}
Pretty straightforward, right? QuickSort excels due to its average-case time complexity of O(n log n). However, beware, as it can degrade to O(n²) if the pivot choices are poor.
Introducing MergeSort
Next, let’s look at MergeSort, another divide-and-conquer-based sorting algorithm. Unlike QuickSort, MergeSort has a consistent O(n log n) time complexity, regardless of how the input data is distributed. It splits the array into two halves, recursively sorts each half, and then merges the sorted halves.
Here’s the implementation of MergeSort in Go:
package main
import "fmt"
func mergeSort(arr []int) []int {
if len(arr) < 2 {
return arr
}
mid := len(arr) / 2
left := mergeSort(arr[:mid])
right := mergeSort(arr[mid:])
return merge(left, right)
}
func merge(left, right []int) []int {
result := make([]int, 0, len(left)+len(right))
i, j := 0, 0
for i < len(left) && j < len(right) {
if left[i] < right[j] {
result = append(result, left[i])
i++
} else {
result = append(result, right[j])
j++
}
}
result = append(result, left[i:]...)
result = append(result, right[j:]...)
return result
}
func main() {
arr := []int{3, 6, 8, 10, 1, 2, 1}
fmt.Println("Unsorted:", arr)
sorted := mergeSort(arr)
fmt.Println("Sorted:", sorted)
}
MergeSort might seem a bit more verbose, but its stability and reliable performance make it a favorite for many developers.
QuickSort vs. MergeSort: The Showdown
Now that we’ve covered both algorithms, let’s compare them!
- Time Complexity: On average, both QuickSort and MergeSort have a time complexity of O(n log n). However, QuickSort can degrade to O(n²) in the worst case.
- Space Complexity: QuickSort has an advantage here with O(log n) space complexity, as it sorts in place. MergeSort, on the other hand, requires O(n) additional space for merging.
- Stability: MergeSort is a stable sort, meaning it maintains the relative order of equal elements. QuickSort is not stable by default.
- Use Cases: Choose QuickSort for its speed and space efficiency in practical scenarios. Opt for MergeSort when dealing with large datasets that require stability and consistent performance.
Practical Insights and Tips
When implementing these algorithms in Go, keep in mind these factors:
- Array Size: For smaller arrays, simpler algorithms like Insertion Sort may be more efficient due to lower overhead.
- Data Distribution: Consider the nature of your data. Nearly sorted or uniformly distributed data can affect the performance of QuickSort.
- Parallelism: MergeSort, with its straightforward divide-and-conquer technique, is more straightforward to parallelize effectively.
- Libraries: Go’s standard library provides an efficient
sort
package. It’s always good to check built-in solutions before rolling your own.
Further Resources
For those eager to dive deeper, check out this comprehensive article on sorting algorithms and their applications: Sorting Algorithms Part 1.
Conclusion
Sorting algorithms like QuickSort and MergeSort are the unsung heroes in our software. Implementing them in Go is not only educational but also a fantastic way to enhance performance. Remember, the best algorithm depends on your data and specific use case. Stay curious, keep experimenting, and most importantly, have fun coding! ?
Until next time, happy coding! ?