Understanding Variables in GoLang: Storing Data for Manipulation Like a Pro
Every programming language uses variables and GoLang is no exception. This post is here to enlighten you on how to use variables in GoLang with a little bit of humor on the side. So, buckle up and let’s dive straight into the world of GoLang variables!
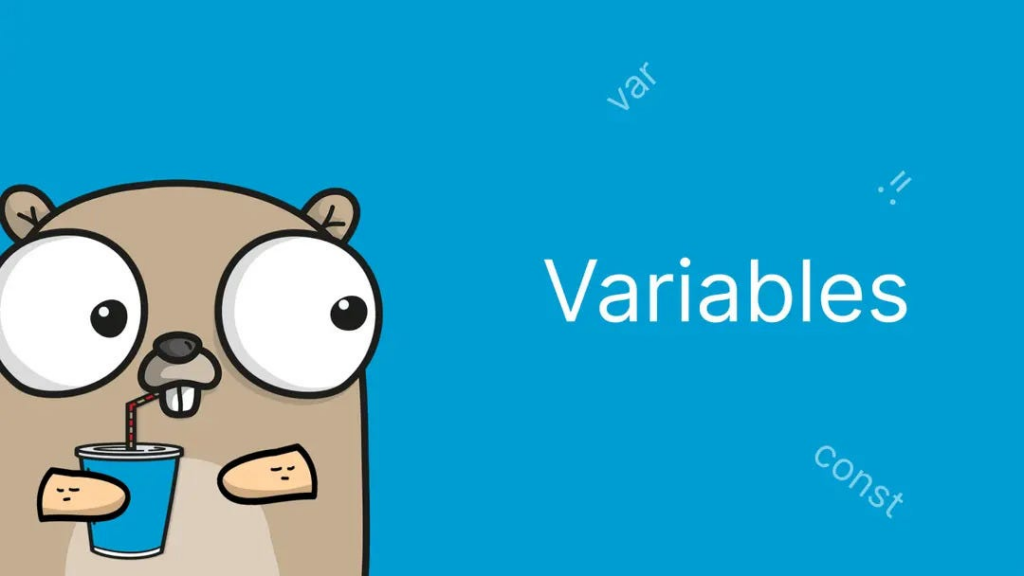
What Exactly are Variables?
At its simplest, a variable is like a waiter at your favorite restaurant. Just as the waiter keeps taking your orders (data), storing, and delivering them to the kitchen staff for preparation (manipulation), variables in a programming language do exactly the same thing.
Declare it Right! – Syntax for GoLang Variables
Declaring variables in GoLang isn’t rocket science, but it does follow a certain syntax. Here’s the most common way of declaring a variable:
var variableName variableType
For example, if you wanted to declare a variable “num” of type “int”, you would write:
var num int
Remember, the variable names in GoLang are case sensitive. So, “num” and “Num” would be treated as two different variables. Talk about the language having a case of selective attention!
Variable Initialization in GoLang
Declaring a variable is great but it’s like having a container without anything in it. We also need to initialize it by assigning a value. Here’s how it’s done:
var num int = 10
However, GoLang is pretty smart, and if you don’t explicitly mention the variable type, it will infer it from the value you assign (Ah! A little touch of magic there).
var num = 10 // GoLang understands that 'num' is an integer.
Short Variable Declaration in GoLang
GoLang has an even shorter method for declaring and initializing variables, aptly called the short variable declaration. It’s swift, it’s easy, and it turns your code into a crisp one-liner. It uses the := operator.
num := 10 // It's that simple!
This method is commonly used inside functions. It’s kind of the espresso shot of variable declarations!
Values, Types, and Address of Variables
In GoLang, each variable holds three things: a value, a type, and an address. Using the Printf function from the fmt package, you can check these as follows:
num := 10
fmt.Printf("Type: %T Value: %v Address: %v", num, num, &num)
The above code returns the type (“int”), value (10), and the memory address where ‘num’ is stored.
Playing Around With Variables
Once you’ve declared and initialized variables, you can use them in many ways, such as in basic mathematical operations, conditional statements, or loops. Here’s a quick example:
var num1 = 10
var num2 = 20
var sum = num1 + num2 //sum is now 30
In GoLang, just like in real life, you’ve got to be careful how you handle your variables. The possibilities — and the responsibilities — are endless.
To conclude, understanding variables in GoLang is fundamental to grasp the language. You can think of mastering variables like learning the ABC’s of the language. After all, you wouldn’t try to write a novel without knowing the alphabets, right?
So, that’s it! With a little bit of practice, you’ll be storing and manipulating data with GoLang variables in no time. Get out there and start coding. And remember, just like a good joke, the best code is all about… execution. Happy coding!