Object-Oriented Programming in GoLang: Modeling Real-World Entities
Hello, fellow tech enthusiasts! Today, we’re going to dive into the fascinating world of Object-Oriented Programming (OOP) in GoLang. If you’re coming from an OOP background in languages like Java, C++, or Python, you may notice that GoLang approaches OOP concepts a bit differently; you could say they do not exist or at least not the same way. But, fear not! We’ll make sense of it all and have loads of fun along the way.
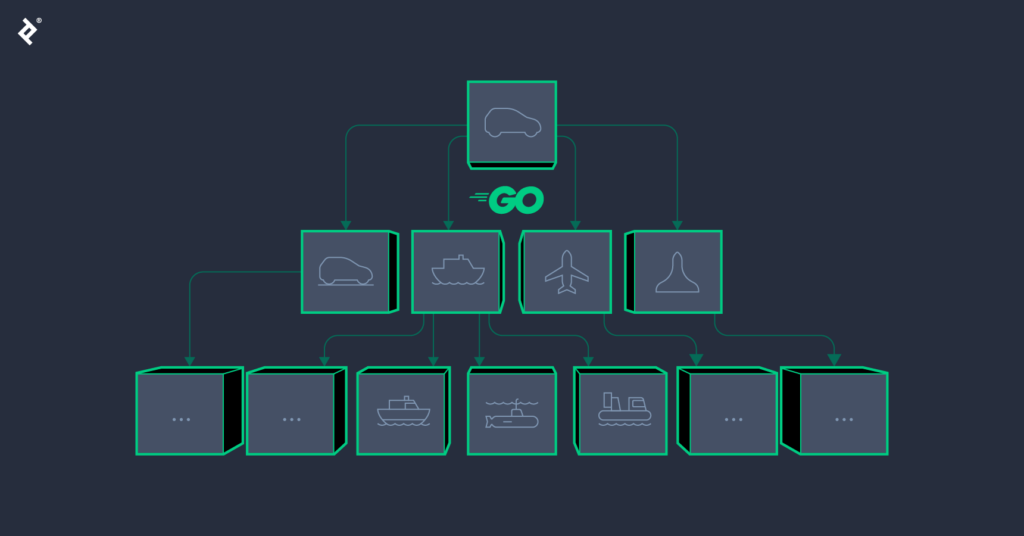
Understanding OOP in GoLang
Unlike some traditional OOP languages, GoLang does not have classes and inheritance. However, it still provides robust mechanisms to model real-world entities through structures (structs) and interfaces. GoLang’s OOP methodologies are lightweight yet powerful, ensuring that your code remains clean, performant, and easy to maintain.
Structs: The Building Blocks
In GoLang, structs are the primary way to model entities. A struct is a collection of fields, encapsulating data that belong together. It looks something like this:
type Car struct {
Make string
Model string
Year int
}
In this example, we’ve defined a Car
struct with fields for the make, model, and year. Here’s how you can create a Car instance:
myCar := Car{
Make: "Tesla",
Model: "Model 3",
Year: 2020,
}
Structs lay the foundation, allowing us to encapsulate and organize complex data succinctly.
Methods: Adding Behavior
To add behavior to our structs, GoLang uses methods. Methods are functions with a special receiver argument. Let’s add a method to our Car
struct:
func (c Car) DisplayDetails() {
fmt.Printf("Make: %s, Model: %s, Year: %d\n", c.Make, c.Model, c.Year)
}
Here, DisplayDetails
is a method with receiver c
of type Car
. This method allows us to print out the details of a car instance.
To call the method, simply write:
myCar.DisplayDetails()
This approach beautifully marries data and behavior, making our code both intuitive and simple to test.
Interfaces: Defining Contracts
In GoLang, interfaces are used to define behavior that types must implement. Let’s see a practical example:
type Vehicle interface {
DisplayDetails()
}
func printDetails(v Vehicle) {
v.DisplayDetails()
}
In this snippet, the Vehicle
interface requires a DisplayDetails
method. Any type that has this method automatically implements the Vehicle
interface. Now, our Car
struct already implements this interface because it has the DisplayDetails
method.
To demonstrate this, we can now pass a Car
instance into the printDetails
function:
printDetails(myCar)
Interfaces make your code highly flexible and decoupled, enabling you to write functions that work with any type implementing specific methods without being concerned about the underlying types.
Extending Structs with Embedded Structs
GoLang doesn’t support traditional inheritance, but it provides composition through embedded structs. Let’s see how this works:
type ElectricCar struct {
Car
BatteryCapacity int
}
In this example, ElectricCar
embeds Car
and adds a new field, BatteryCapacity
. This means ElectricCar
inherits all fields and methods from Car
, and you can instantiate it like so:
myEV := ElectricCar{
Car: Car{
Make: "Tesla",
Model: "Model S",
Year: 2021,
},
BatteryCapacity: 100,
}
You can now call the inherited DisplayDetails
method as:
myEV.DisplayDetails()
fmt.Printf("Battery Capacity: %d kWh\n", myEV.BatteryCapacity)
Fully Embracing OOP in GoLang
While GoLang’s approach to OOP might seem unconventional, it actually provides a simpler, more concise way to implement object-oriented designs. By focusing on composition over inheritance, and using interfaces to define behavior, GoLang encourages the creation of clean, maintainable, and reusable code.
For further exploration of GoLang and its unique OOP capabilities, I highly recommend checking out the official GoLang documentation. It’s packed with fantastic resources and examples that will help you deepen your understanding and proficiency with the language.
Conclusion
OOP in GoLang might not involve classes and traditional inheritance hierarchies, but its use of structs, methods, interfaces, and embedded structs offers a powerful and flexible way to model real-world entities. Embracing these principles will not only make your code more manageable and efficient but also open your mind to new ways of organizing and thinking about programming problems.
Thanks for joining me on this journey through GoLang’s object-oriented landscape. Until next time, happy coding! ?