Pathfinding Algorithms in Go: Dijkstra’s Algorithm vs. A*
Hello, tech enthusiasts! Today we’re diving into the fascinating world of pathfinding algorithms, specifically Dijkstra’s Algorithm and A*. These algorithms are crucial for navigation, gaming, and a multitude of AI applications. We’ll explore how they work, their differences, and how to implement them in Go. So, fasten your seatbelts as we embark on this computational journey!
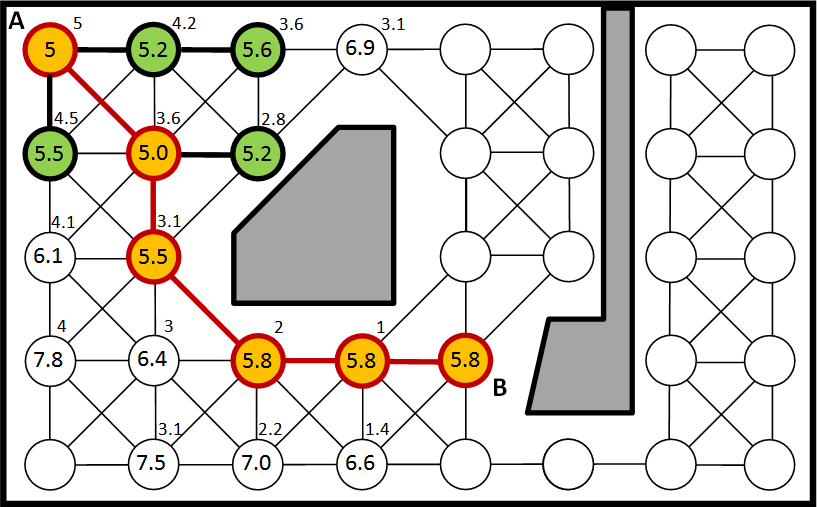
We have written related posts in the past, please check them out.
Understanding Pathfinding Algorithms
Pathfinding algorithms are essential in computer science for finding the shortest path between two points. They are extensively used in various fields such as robotics, game development, and network routing.
Dijkstra’s Algorithm: The Pioneer
Dijkstra’s Algorithm, named after Edsger Dijkstra, is a classic pathfinding algorithm. It guarantees the shortest path in a graph with non-negative weights. Here’s a step-by-step breakdown of Dijkstra’s Algorithm:
1. Initialization: Mark all nodes unvisited. Set the distance to the starting node to 0 and to all other nodes to infinity.
2. Visit the Unvisited Node with the Shortest Distance: From the current node, consider all its unvisited neighbors and calculate their tentative distances through the current node. Update the neighbor’s distance if the calculated distance is less than the known distance.
3. Mark the Current Node as Visited: This node is added to the path and no longer considered for further updates.
4. Repeat: Continue the process until all nodes are visited.
5. Result: The shortest path is found.
Here’s a Go implementation of Dijkstra’s Algorithm:
package main
import (
"container/heap"
"fmt"
)
type Node struct {
ID int
Cost int
Distance int
}
type PriorityQueue []*Node
func (pq PriorityQueue) Len() int { return len(pq) }
func (pq PriorityQueue) Less(i, j int) bool {
return pq[i].Distance < pq[j].Distance
}
func (pq PriorityQueue) Swap(i, j int) {
pq[i], pq[j] = pq[j], pq[i]
}
func (pq *PriorityQueue) Push(x interface{}) {
*pq = append(*pq, x.(*Node))
}
func (pq *PriorityQueue) Pop() interface{} {
old := *pq
n := len(old)
item := old[n-1]
*pq = old[0 : n-1]
return item
}
func Dijkstra(graph map[int]map[int]int, start int) map[int]int {
distances := make(map[int]int)
for node := range graph {
distances[node] = 1<<31 - 1 // infinite
}
distances[start] = 0
pq := &PriorityQueue{}
heap.Init(pq)
heap.Push(pq, &Node{ID: start, Distance: 0})
for pq.Len() > 0 {
current := heap.Pop(pq).(*Node)
for neighbor, weight := range graph[current.ID] {
alt := distances[current.ID] + weight
if alt < distances[neighbor] {
distances[neighbor] = alt
heap.Push(pq, &Node{ID: neighbor, Distance: alt})
}
}
}
return distances
}
func main() {
graph := map[int]map[int]int{
0: {1: 4, 7: 8},
1: {0: 4, 2: 8, 7: 11},
2: {1: 8, 3: 7, 8: 2, 5: 4},
3: {2: 7, 4: 9, 5: 14},
4: {3: 9, 5: 10},
5: {2: 4, 3: 14, 4: 10, 6: 2},
6: {5: 2, 7: 1, 8: 6},
7: {0: 8, 1: 11, 6: 1, 8: 7},
8: {2: 2, 6: 6, 7: 7},
}
distances := Dijkstra(graph, 0)
fmt.Println("Shortest paths from node 0:")
for node, distance := range distances {
fmt.Printf("Node %d: %d\n", node, distance)
}
}
A*: The Heuristic Mate
A* (pronounced A-star) is another powerful pathfinding algorithm. It enhances Dijkstra’s Algorithm by incorporating a heuristic function to guide the search. This heuristic estimates the cost to reach the goal, thereby speeding up the route search.
The A* algorithm can be broken down as follows:
1. Initialization: Similar to Dijkstra’s, but includes a heuristic estimate.
2. Visit Nodes: Nodes are picked based on the sum of the path cost from the start and the heuristic cost to the goal.
3. Evaluate Path: Nodes in A* have a score, f(n) = g(n) + h(n), where g(n) is the path cost from start to the current node n, and h(n) is the estimated cost to reach the goal.
4. Repeat and Find the Optimal Path: Continue until the goal is reached, ensuring the path with the lowest score is selected.
Let’s look at a Go implementation for the A* algorithm:
package main
import (
"container/heap"
"fmt"
"math"
)
type AStarNode struct {
ID int
Cost int
Distance int
Heuristic int
}
type AStarPriorityQueue []*AStarNode
func (pq AStarPriorityQueue) Len() int { return len(pq) }
func (pq AStarPriorityQueue) Less(i, j int) bool {
return pq[i].Heuristic + pq[i].Distance < pq[j].Heuristic + pq[j].Distance
}
func (pq AStarPriorityQueue) Swap(i, j int) {
pq[i], pq[j] = pq[j], pq[i]
}
func (pq *AStarPriorityQueue) Push(x interface{}) {
*pq = append(*pq, x.(*AStarNode))
}
func (pq *AStarPriorityQueue) Pop() interface{} {
old := *pq
n := len(old)
item := old[n-1]
*pq = old[0 : n-1]
return item
}
func heuristic(a, b int) int {
return int(math.Abs(float64(a - b)))
}
func AStar(graph map[int]map[int]int, start, goal int) map[int]int {
distances := make(map[int]int)
for node := range graph {
distances[node] = 1<<31 - 1 // infinite
}
distances[start] = 0
pq := &AStarPriorityQueue{}
heap.Init(pq)
heap.Push(pq, &AStarNode{ID: start, Distance: 0, Heuristic: heuristic(start, goal)})
for pq.Len() > 0 {
current := heap.Pop(pq).(*AStarNode)
if current.ID == goal {
break
}
for neighbor, weight := range graph[current.ID] {
alt := distances[current.ID] + weight
if alt < distances[neighbor] {
distances[neighbor] = alt
est := heuristic(neighbor, goal)
heap.Push(pq, &AStarNode{ID: neighbor, Distance: alt, Heuristic: est})
}
}
}
return distances
}
func main() {
graph := map[int]map[int]int{
0: {1: 4, 7: 8},
1: {0: 4, 2: 8, 7: 11},
2: {1: 8, 3: 7, 8: 2, 5: 4},
3: {2: 7, 4: 9, 5: 14},
4: {3: 9, 5: 10},
5: {2: 4, 3: 14, 4: 10, 6: 2},
6: {5: 2, 7: 1, 8: 6},
7: {0: 8, 1: 11, 6: 1, 8: 7},
8: {2: 2, 6: 6, 7: 7}
}
start, goal := 0, 4
distances := AStar(graph, start, goal)
fmt.Printf("Shortest path from node %d to node %d:\n", start, goal)
for node, distance := range distances {
fmt.Printf("Node %d: %d\n", node, distance)
}
}
Dijkstra’s vs. A*: Head-to-Head
While both algorithms are pathfinding champions, there are key differences:
– Dijkstra’s Algorithm:
– Always finds the shortest path.
– Explores all possible paths, leading to high computational costs.
– Best used when all edge weights are non-negative.
– A* Algorithm:
– Uses heuristics for more efficient searches.
– Can quickly direct the search towards the target with lower computational effort.
– Ideal when a faster solution is acceptable over guaranteed shortest paths.
For more in-depth exploration, check out this comparison on GeeksforGeeks.
Conclusion
Both Dijkstra’s and A* algorithms are fundamental tools in the pathfinding arsenal. Understanding and implementing them in Go paves the way to solving complex routing and navigation problems. Whether you need the precision of Dijkstra’s or the efficiency of A*, having both in your toolkit is invaluable. Happy coding, and may your paths always be well-defined and optimally charted!
Keep exploring, keep coding, and never stop innovating!