Real-Time Web Applications with WebSockets and Socket.io; Unlocking Advanced Features
Now that you’ve familiarized yourself with the foundational concepts of WebSockets and Socket.io, and how they revolutionize real-time interactions on the web, it’s time to get our hands dirty with implementation. For those just tuning in, I highly recommend checking out our comprehensive guide on WebSockets and Socket.io to catch up on the basics.
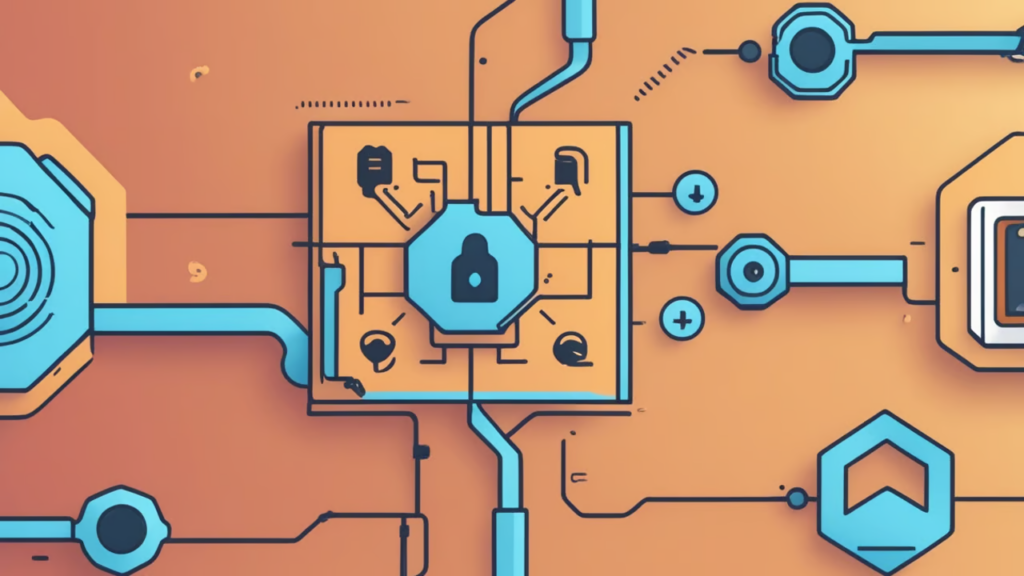
Setting Up Your Environment
To start, ensure you have Node.js and npm installed on your machine. If they’re not installed, head over to the official Node.js site and grab the latest stable version.
First, let’s create a new directory for our project and initialize it:
mkdir realtime-app
cd realtime-app
npm init -y
Next, we need to install `express` and `socket.io`:
npm install express socket.io
Creating the Server
In your project directory, create a file named `server.js`. This will serve as our entry point. In this file, we will set up a basic Express server and integrate Socket.io for real-time communications:
const express = require('express');
const http = require('http');
const socketIo = require('socket.io');
const app = express();
const server = http.createServer(app);
const io = socketIo(server);
app.get('/', (req, res) => {
res.sendFile(__dirname + '/index.html');
});
// Listening for connections
io.on('connection', (socket) => {
console.log('A user connected');
socket.on('disconnect', () => {
console.log('User disconnected');
});
});
server.listen(3000, () => {
console.log('Listening on *:3000');
});
This simple server listens for connections and logs when a user connects or disconnects. We are serving a basic HTML file at the root endpoint which we’ll create next.
Creating the Client-Side Code
Create a file named `index.html` in the root of your project, and add the following content to it:
<!DOCTYPE html>
<html>
<head>
<title>Socket.io Chat</title>
<script src="https://cdn.socket.io/4.0.0/socket.io.min.js"></script>
<script>
document.addEventListener("DOMContentLoaded", () => {
var socket = io();
socket.on('connect', () => {
console.log('Connected to server');
});
socket.on('disconnect', () => {
console.log('Disconnected from server');
});
});
</script>
</head>
<body>
<h1>Welcome to Socket.io Chat!</h1>
</body>
</html>
Here, we load the Socket.io client library and establish a connection to our server. When the server sends events for connecting or disconnecting, the client logs it to the console.
Adding Real-Time Features
To build a real-time chat application, we’ll need to add some interaction to our front end and back end.
First, let’s update our `index.html` to include a form for sending messages and a list to display them:
<!DOCTYPE html>
<html>
<head>
<title>Socket.io Chat</title>
<script src="https://cdn.socket.io/4.0.0/socket.io.min.js"></script>
<script>
document.addEventListener("DOMContentLoaded", () => {
var socket = io();
var form = document.getElementById('form');
var input = document.getElementById('input');
socket.on('connect', () => {
console.log('Connected to server');
});
socket.on('disconnect', () => {
console.log('Disconnected from server');
});
socket.on('chat message', (msg) => {
var item = document.createElement('li');
item.textContent = msg;
document.getElementById('messages').appendChild(item);
});
form.addEventListener('submit', (e) => {
e.preventDefault();
if (input.value) {
socket.emit('chat message', input.value);
input.value = '';
}
});
});
</script>
</head>
<body>
<h1>Socket.io Chat</h1>
<ul id="messages"></ul>
<form id="form" action="">
<input id="input" autocomplete="off" /><button>Send</button>
</form>
</body>
</html>
Next, update `server.js` to handle the `chat message` event:
const express = require('express');
const http = require('http');
const socketIo = require('socket.io');
const app = express();
const server = http.createServer(app);
const io = socketIo(server);
app.get('/', (req, res) => {
res.sendFile(__dirname + '/index.html');
});
// Listening for connections
io.on('connection', (socket) => {
console.log('A user connected');
socket.on('disconnect', () => {
console.log('User disconnected');
});
socket.on('chat message', (msg) => {
io.emit('chat message', msg);
});
});
server.listen(3000, () => {
console.log('Listening on *:3000');
});
Now, whenever a client sends a chat message, it emits the event to all connected clients, causing the message to be displayed in real-time on everyone’s screen. Exciting, right?
Conclusion
By following this guide, you have successfully set up a real-time web application utilizing WebSockets and Socket.io. The potential applications of this technology are vast, ranging from chat applications and live notifications to collaborative tools and real-time analytics. If you’re eager to delve deeper, explore the Socket.io documentation which offers a plethora of advanced features to fine-tune and expand your app’s capabilities.
Thank you for joining me on this exciting journey into real-time web applications. If you found this post helpful, be sure to check out our previous articles and stay tuned for more in-depth guides and insights. Happy coding!