Real-World Applications of Python vs. Go: Practical Projects Beginners Can Tackle with Each Language
Hello, tech enthusiasts! If you’re here, you’re probably grappling with the delicious dilemma of choosing between learning Python or Go. Both are incredible programming languages, each with its unique strengths and use cases. But, as a newcomer, you might be wondering what kinds of real-world applications you can start building with each. Let’s explore some practical projects for beginners that showcase the power of both Python and Go.
This is far from our first post on the subject. In fact we have a library on this subject for different criteria.
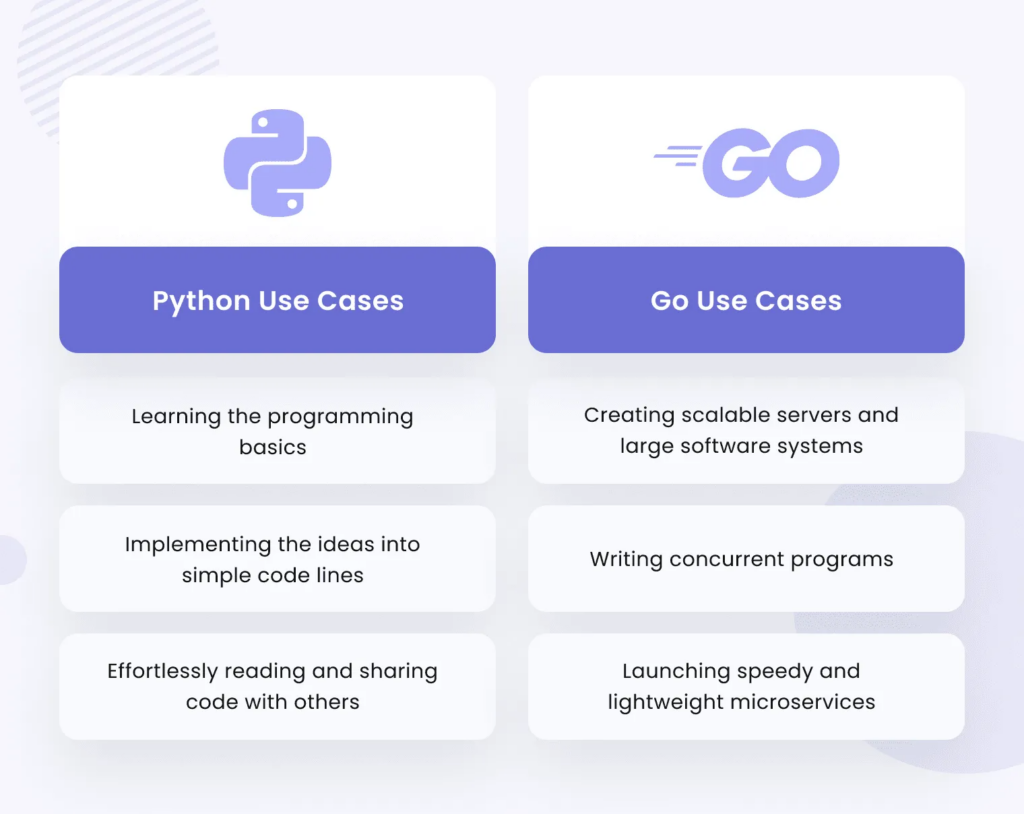
Why Learn Python?
Python is a versatile, high-level programming language that is incredibly beginner-friendly, thanks to its simple and readable syntax. Here are a few reasons why Python is a fantastic starting point:
- Easy to Learn: Python’s syntax closely resembles English, making it easier to read and write.
- Vast Libraries and Frameworks: It has an extensive range of libraries that make tasks simpler.
- Great Community Support: Python has a vibrant community, ensuring plenty of learning resources and support.
Python Project Ideas for Beginners
Dive into these practical Python projects to kickstart your learning journey:
Web Scraping with Beautiful Soup
Web scraping is a technique used to extract data from websites. Python’s Beautiful Soup library makes web scraping a breeze. Here’s a simple example:
import requests
from bs4 import BeautifulSoup
url = 'https://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
for heading in soup.find_all('h2'):
print(heading.text)
This project will help you understand HTML structure and how to extract information, which is incredibly useful for data analysis.
Building a Basic Web Application with Flask
Flask is a lightweight web framework for Python. It’s perfect for beginners to create their first web application. Here’s a snippet to get you started:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def home():
return "Hello, Flask!"
if __name__ == '__main__':
app.run(debug=True)
With Flask, you can expand this basic application to include routes, templates, and even a database. Flask tutorials are abundant and comprehensive online.
Automating Tasks with Python
One of Python’s strengths is its ability to automate boring tasks. By using libraries like Schedule, you can automate anything from sending emails to organizing files on your computer:
import schedule
import time
def job():
print("Task executed!")
schedule.every(10).minutes.do(job)
while True:
schedule.run_pending()
time.sleep(1)
Simple, yet effective for day-to-day automation!
Why Learn Go?
Go, also known as Golang, is a statically-typed, compiled programming language developed by Google. It’s known for its performance and simplicity. Here’s why Go might be exciting for you:
- Performance: Go is fast and efficient, as it compiles directly to machine code.
- Concurrency: With Goroutines, Go handles concurrent tasks effortlessly.
- Simplicity: Go has a clean syntax and is easy to learn.
Go Project Ideas for Beginners
Ready to dive into Go? Here are some beginner-friendly projects:
Creating a Simple REST API
Go is renowned for building robust web services and APIs. Here’s a starter example of a REST API using Go’s net/http package:
package main
import (
"encoding/json"
"net/http"
)
func helloWorld(w http.ResponseWriter, r *http.Request) {
w.Header().Set("Content-Type", "application/json")
json.NewEncoder(w).Encode(map[string]string{"message": "Hello, World!"})
}
func main() {
http.HandleFunc("/", helloWorld)
http.ListenAndServe(":8080", nil)
}
This example sets up a simple endpoint that returns a JSON response. You can expand it to handle more routes and data operations!
Building a CLI Tool
Go is perfect for building command-line tools due to its speed and simplicity. Using the Cobra library, you can create a CLI tool effortlessly:
package main
import (
"fmt"
"github.com/spf13/cobra"
)
func main() {
var rootCmd = &cobra.Command{Use: "app"}
var helloCmd = &cobra.Command{
Use: "hello",
Short: "Prints Hello, World!",
Run: func(cmd *cobra.Command, args []string) {
fmt.Println("Hello, World!")
},
}
rootCmd.AddCommand(helloCmd)
rootCmd.Execute()
}
This basic CLI tool can be customized and expanded for various functionalities, making it incredibly useful.
Concurrent Web Scraper
Go’s concurrency model makes it ideal for tasks like web scraping. Here’s a simple concurrent web scraper using Goroutines:
package main
import (
"fmt"
"io/ioutil"
"net/http"
"sync"
)
func fetchURL(wg *sync.WaitGroup, url string) {
defer wg.Done()
resp, err := http.Get(url)
if err != nil {
fmt.Println(err)
return
}
body, _ := ioutil.ReadAll(resp.Body)
fmt.Println(string(body))
}
func main() {
var wg sync.WaitGroup
urls := []string{"https://example.com", "https://example.org"}
for _, url := range urls {
wg.Add(1)
go fetchURL(&wg, url)
}
wg.Wait()
}
This project introduces you to Go’s powerful concurrency features and is a great way to understand how Goroutines work.
Conclusion
Both Python and Go have their unique strengths and are suited to different types of projects. Python’s simplicity and vast libraries make it perfect for web development, data analysis, and automation. On the other hand, Go’s performance and concurrency model make it ideal for building fast, scalable web services and efficient tools.
Ultimately, the choice between Python and Go depends on your interests and the type of projects you wish to tackle. Whether you choose to explore the vast libraries of Python or the efficient concurrency of Go, both paths will lead you to exciting and rewarding programming adventures.
Happy coding! And remember, the world of programming is vast and full of opportunities. Keep learning and stay curious!