Sorting Algorithms Face-Off in Python: QuickSort vs. MergeSort
When it comes to sorting algorithms, QuickSort and MergeSort often find themselves in the spotlight. Both are favorites due to their efficiency and performance, yet they differ in several aspects. Today, we’ll dive deep into these two powerful sorting techniques and see how they stack up against each other in Python. So, buckle up and get ready for a thrilling sorting showdown!
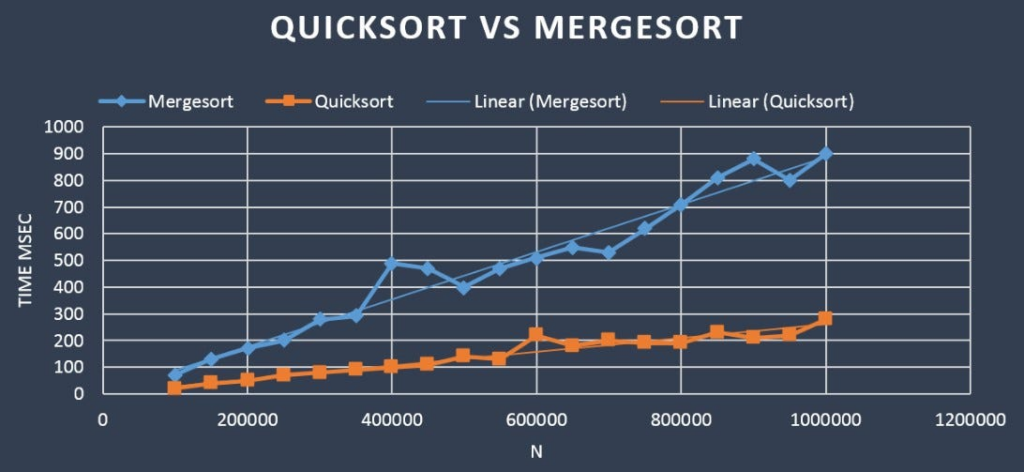
Understanding QuickSort
QuickSort is a divide-and-conquer algorithm that works by selecting a ‘pivot’ element from the array and partitioning the other elements into two sub-arrays—one with elements less than the pivot and one with elements greater than the pivot. It then recursively sorts the sub-arrays. The relative simplicity and efficiency make QuickSort a popular choice.
QuickSort Implementation in Python
Here’s how you can implement QuickSort in Python:
def quicksort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quicksort(left) + middle + quicksort(right)
# Example usage:
arr = [3, 6, 8, 10, 1, 2, 1]
print(quicksort(arr))
QuickSort’s average-case time complexity is \(O(n \log n)\), but it can degrade to \(O(n^2)\) in the worst case when the smallest or largest element is always picked as the pivot. Despite this, QuickSort is often faster in practice due to its cache efficiency and low overhead.
Understanding MergeSort
MergeSort is also a divide-and-conquer algorithm, which divides the array into two halves, recursively sorts them, and then merges the sorted halves to produce the sorted array. Unlike QuickSort, the splits are always in the middle, ensuring a consistent time complexity.
MergeSort Implementation in Python
Let’s see how MergeSort can be implemented in Python:
def mergesort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = mergesort(arr[:mid])
right = mergesort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i = j = 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
result.append(left[i]
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
# Example usage:
arr = [3, 6, 8, 10, 1, 2, 1]
print(mergesort(arr))
MergeSort’s time complexity is \(O(n \log n)\) consistently, making it a reliable option especially when performance in the worst-case scenario is critical. However, it requires additional space for merging, leading to a space complexity of \(O(n)\).
QuickSort vs. MergeSort: Key Differences
Time Complexity
Both algorithms have an average time complexity of \(O(n \log n)\). However, QuickSort’s worst-case time complexity can degrade to \(O(n^2)\), while MergeSort maintains \(O(n \log n)\) in both average and worst cases.
Space Complexity
QuickSort is generally more space-efficient with an in-place version requiring \(O(\log n)\) additional space. MergeSort, on the other hand, requires \(O(n)\) additional space due to the merging process.
Stability
MergeSort is a stable sorting algorithm, meaning that it maintains the relative order of equal elements. QuickSort, however, is not stable by default.
Performance in Practice
In practice, QuickSort tends to outperform MergeSort due to better cache performance and lower constant factors. This is why many standard libraries, including Python’s built-in `sort()`, use a variant of QuickSort called Timsort.
When to Use Which?
Choosing between QuickSort and MergeSort boils down to specific use cases:
- QuickSort – Use QuickSort when average performance is more critical than worst-case performance, especially for large datasets stored in memory. Its in-place sorting and low overhead make it a speed demon in practice.
- MergeSort – Opt for MergeSort when stable sorting is needed or when working with linked lists. Its consistent \(O(n \log n)\) time complexity ensures predictable performance, and it handles large datasets stored on slower media well.
Resources for Further Reading
If you’re interested in diving deeper into sorting algorithms and exploring more about QuickSort and MergeSort, check out these excellent resources:
Wrapping Up
Both QuickSort and MergeSort have their strengths and specific use-case scenarios. By understanding their inner workings and performance characteristics, you can make informed decisions on which to use for your next project. Remember, the right tool for the job makes all the difference, and knowing when and how to use these powerful sorting algorithms is a key step towards efficient coding. Keep exploring, keep coding, and let’s keep pushing the boundaries of what’s possible with Python!