Sorting Algorithms in Go: QuickSort vs. MergeSort
Sorting algorithms are the backbone of many computer science problems, and understanding how they work can significantly enhance your coding efficiency. Today, we’ll dive into two of the most powerful and commonly used sorting algorithms: QuickSort and MergeSort, specifically in the Go programming language (aka Golang). Whether you’re a seasoned developer or just starting out, this guide will walk you through the basics and help you understand the pros and cons of each algorithm.
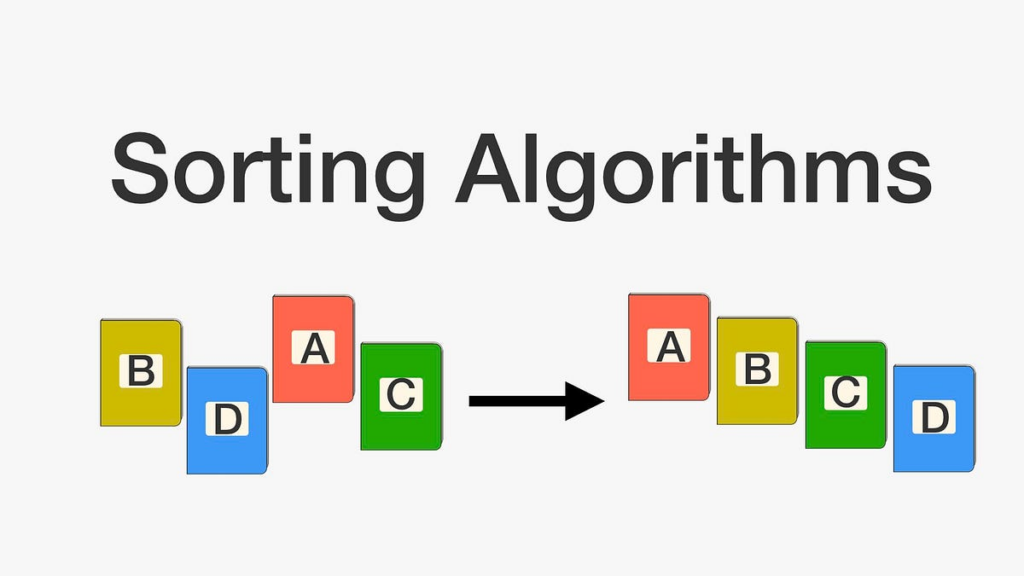
Introduction to Sorting Algorithms
Sorting algorithms are procedures that arrange data in a particular order, commonly in ascending or descending numerical or lexicographical order. Effective sorting is crucial for optimizing the efficiency of other algorithms that require sorted data to work correctly. Furthermore, non-trivial software systems often need to sort large volumes of data, making the choice of sorting algorithm vital for performance.
QuickSort: Speed and Efficiency
QuickSort is a highly efficient sorting algorithm and is based on the divide-and-conquer approach. Tony Hoare developed it in 1959, and its time complexity is approximately O(n log n) on average cases, though it can degrade to O(n^2) in the worst cases. QuickSort is faster in practice than other sorting algorithms, provided the array’s partitioning is balanced.
QuickSort Implementation in Go
package main
import (
"fmt"
)
func quickSort(arr []int) []int {
if len(arr) <= 1 {
return arr
}
pivot := arr[len(arr)/2]
left := []int{}
right := []int{}
for _, v := range arr {
if v < pivot {
left = append(left, v)
} else if v > pivot {
right = append(right, v)
}
}
return append(append(quickSort(left), pivot), quickSort(right)...)
}
func main() {
data := []int{34, 7, 23, 32, 5, 62}
fmt.Println("Unsorted:", data)
sortedData := quickSort(data)
fmt.Println("Sorted:", sortedData)
}
The Go code above implements QuickSort. First, we define the function quickSort
, which recursively sorts the elements. The pivot element (used for partitioning) is the middle element of the array. Then, we create two sub-arrays: left
and right
, containing the elements smaller and larger than the pivot, respectively. Finally, we concatenate the left
array, pivot, and right
array using the append
function.
MergeSort: Stability and Predictability
MergeSort is another excellent example of a divide-and-conquer algorithm. It was invented by John von Neumann in 1945. Unlike QuickSort, MergeSort guarantees O(n log n) time complexity in all scenarios. Furthermore, MergeSort is a stable sort, which means it preserves the order of equal elements.
MergeSort Implementation in Go
package main
import (
"fmt"
)
func mergeSort(arr []int) []int {
if len(arr) <= 1 {
return arr
}
mid := len(arr) / 2
left := mergeSort(arr[:mid])
right := mergeSort(arr[mid:])
return merge(left, right)
}
func merge(left, right []int) []int {
result := []int{}
i, j := 0, 0
for i < len(left) && j < len(right) {
if left[i] < right[j] {
result = append(result, left[i])
i++
} else {
result = append(result, right[j])
j++
}
}
for i < len(left) {
result = append(result, left[i])
i++
}
for j < len(right) {
result = append(result, right[j])
j++
}
return result
}
func main() {
data := []int{34, 7, 23, 32, 5, 62}
fmt.Println("Unsorted:", data)
sortedData := mergeSort(data)
fmt.Println("Sorted:", sortedData)
}
The Go code above shows how to implement MergeSort. The function mergeSort
splits the array into two halves until arrays of one element are obtained. It uses the merge
function to combine these smaller arrays back into a sorted array. This process guarantees a consistent O(n log n) run time due to the balanced nature of the division.
QuickSort vs. MergeSort: When to Use What?
Understanding when to use QuickSort or MergeSort can make a significant impact on application performance:
- QuickSort: It’s often faster for smaller datasets due to lower memory overhead. It’s also highly advantageous for in-place sorting, requiring less additional memory.
- MergeSort: This algorithm shines with larger datasets and provides stable sorting, making it ideal for applications where the order of equal elements matters. It also performs consistently across different types of data.
Conclusion
Both QuickSort and MergeSort are indispensable tools in any developer’s toolkit. QuickSort is your go-to for speed and efficiency in smaller datasets, while MergeSort offers stability and predictability, especially in large or ordered datasets.
Understanding the strengths and weaknesses of these algorithms will allow you to select the best method for your specific needs. Happy coding!
For further reading, check out this detailed comparison on QuickSort vs. MergeSort.
Keep Exploring!
Stay curious, keep experimenting, and happy coding! Sorting might seem trivial, but knowing the right algorithm can make or break your application’s performance. Enjoy your journey into the world of sorting algorithms, and remember, the best way to learn is by doing. So, get your hands on some code and start sorting!