Libraries in Python: Reusing Pre-Written Code
Hello, fellow coders and developers! Today, we're diving deep into one of Python's most powerful features: libraries. If you've ever marveled at how quickly you can get things done in Python, there's a good chance you've taken advantage of this treasure trove. Libraries are indeed like magic wands that allow you to reuse pre-written code, enhance your projects, and make coding life so much more fun and efficient!
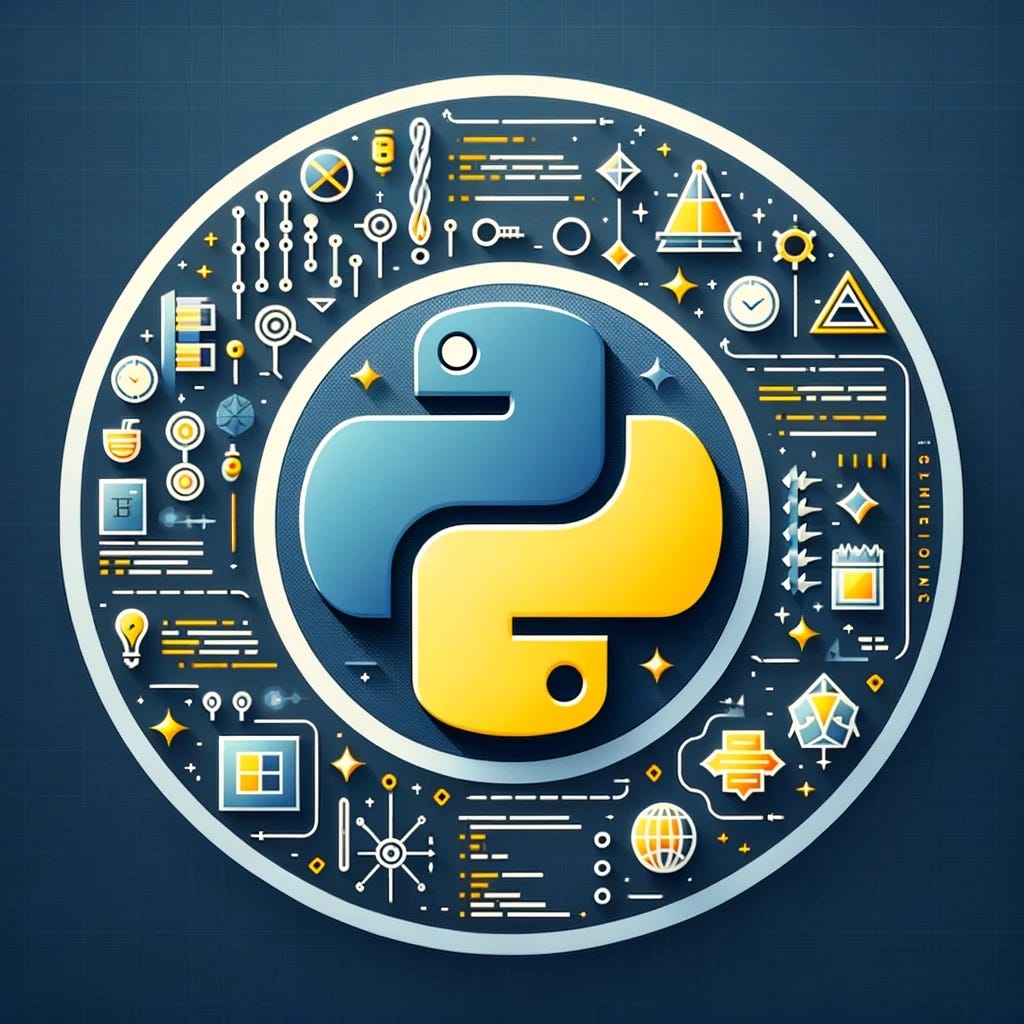
Why Libraries?
Let's face it; nobody likes reinventing the wheel. Whether you're working on a small script or a huge project, you want to focus on the unique aspects of your work, not spend countless hours writing boilerplate code. Python's extensive collection of libraries provides you with functionalities ranging from scientific computing to web development, data analytics, machine learning, and more.
These libraries are essentially bundles of pre-written Python code created by experts. And the best part? They're often open-source, meaning you can use, modify, and contribute back to them in the true spirit of collaboration!
Popular Python Libraries
To give you a better sense of how you can leverage libraries in your projects, let's explore some of the most popular ones:
1. NumPy
NumPy is the go-to library for numerical computing in Python. Its powerful N-dimensional array object allows for fast, flexible, and efficient operations across arrays. You’ll find NumPy indispensable for any kind of mathematical computation or data manipulation.
import numpy as np
a = np.array([1, 2, 3, 4])
b = np.array([2, 3, 4, 5])
c = a + b # array([3, 5, 7, 9])
print(c)
2. Pandas
Pandas brings the capability of easily handling data structures and performing data analysis operations in Python. You can think of it as Excel on steroids!
import pandas as pd
data = {'Name': ['John', 'Anna', 'Peter', 'Linda'],
'Age': [28, 24, 35, 32]}
df = pd.DataFrame(data)
print(df)
3. Matplotlib
Matplotlib is another must-have library for anyone looking to visualize data. From basic line graphs to pie charts and complex 3D plots, Matplotlib has got you covered.
import matplotlib.pyplot as plt
years = [1950, 1960, 1970, 1980, 1990, 2000, 2010]
gdp = [300.2, 543.3, 1075.9, 2862.5, 5979.6, 10289.7, 14958.3]
plt.plot(years, gdp, color='green') # line plot
plt.xlabel('Years')
plt.ylabel('GDP in Billions')
plt.title('GDP over Years')
plt.show()
4. Requests
If you ever need to make HTTP requests in Python, look no further than Requests. Whether you're pulling data from a web API or scraping the web, Requests makes the process straightforward and hassle-free.
import requests
response = requests.get('https://api.github.com')
print(response.status_code)
print(response.json())
5. TensorFlow
For those venturing into machine learning and AI, TensorFlow is the library you're likely to use. Created by Google, it’s a comprehensive framework for building machine learning models.
import tensorflow as tf
# Simple hello world in TensorFlow
hello = tf.constant('Hello, TensorFlow!')
sess = tf.Session()
print(sess.run(hello))
How to Install a Python Library
Installing these libraries is a piece of cake thanks to pip, Python’s package installer. To install any library, simply open your terminal and type:
pip install libraryname
For example, to install NumPy, you’d use:
pip install numpy
Making the Most of Libraries
Utilizing libraries not only speeds up development but also means your code is more reliable, since these libraries have often been thoroughly tested and optimized. Additionally, they allow for better collaboration; your colleagues or collaborators who are Python-savvy will likely be familiar with these libraries.
That being said, it's crucial to stay up-to-date with the versions of libraries you're using. Make sure to read the documentation, understand the functionalities, and new features. To help with this, you can check out PyPI, the Python Package Index, which lists all available libraries.
Community and Resources
One of Python's greatest strengths is its community. Websites like Stack Overflow and numerous coding forums have countless discussions, tutorials, and code snippets that can help you solve almost any problem you may encounter.
Additionally, most libraries have dedicated documentation websites that offer detailed instructions, examples, and best practices. Always check the documentation; it’s the ultimate resource for understanding how to leverage a library's full potential.
Conclusion
Incorporating libraries into your projects is like opening a door to a world of possibilities. These powerful tools enable you to focus on your unique work, while the heavy lifting is handled by pre-written, optimized, and meticulously tested code. So go ahead, explore, experiment, and don’t be afraid to dig into the code. Libraries are there to help you bring your ideas to life more efficiently than ever before.
Remember, the world of Python libraries is vast and always evolving. The more you explore, the better you'll get at identifying which tools can best meet your needs. So, keep coding, keep learning, and, most importantly, have fun doing it! If you found this post helpful, don't forget to share it and keep an eye out for more tips and tricks on maximizing your Python potential!
Until next time, happy coding!