Understanding Error Handling in GoLang: Managing Runtime Errors
Hey there, tech enthusiasts! Today, we're diving into a fascinating topic that is crucial for every GoLang developer: Error Handling. Errors are an inevitable part of software development, and the way we manage them can make or break our code. Go's approach to error handling is unique and offers many advantages once you get the hang of it. Let's break it down and explore how to handle runtime errors effectively in GoLang!
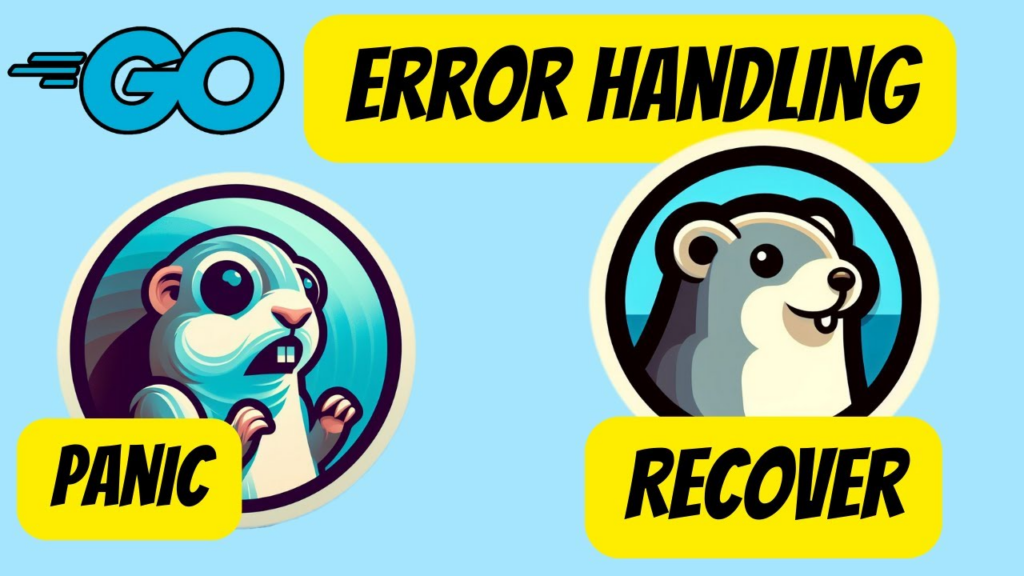
What Makes Error Handling in GoLang Different?
GoLang, often referred to as just "Go," stands out because it emphasizes simplicity and efficiency. Unlike other languages, Go does not use exceptions to handle errors. Instead, errors are treated as regular return values. This might seem strange at first, but as you get accustomed to it, you'll realize it promotes better error handling and cleaner code.
The Basics: Returning Errors from Functions
In Go, functions often return multiple values, where the last value is usually the error. Here's a basic example to illustrate this concept:
package main
import (
"errors"
"fmt"
)
func divide(a, b float64) (float64, error) {
if b == 0 {
return 0, errors.New("division by zero")
}
return a / b, nil
}
func main() {
result, err := divide(10, 0)
if err != nil {
fmt.Println("Error:", err)
} else {
fmt.Println("Result:", result)
}
}
In this example, the divide
function returns both the result of the division and an error. If the division by zero is attempted, an error is returned with a descriptive message. The caller then checks if an error occurred and handles it accordingly.
Custom Errors
Go also allows for the creation of custom error types. This can be extremely useful for more complex error handling scenarios. Here's how you can create and use custom errors:
package main
import (
"fmt"
)
type DivideError struct {
Dividend float64
Divisor float64
Message string
}
func (e *DivideError) Error() string {
return fmt.Sprintf("Cannot divide %.2f by %.2f: %s", e.Dividend, e.Divisor, e.Message)
}
func divide(a, b float64) (float64, error) {
if b == 0 {
return 0, &DivideError{a, b, "division by zero"}
}
return a / b, nil
}
func main() {
result, err := divide(10, 0)
if err != nil {
fmt.Println("Error:", err)
} else {
fmt.Println("Result:", result)
}
}
Here, we define a custom error type DivideError
with additional context about the error. This makes debugging easier and your code more informative.
Panic and Recover: Handling Unexpected Errors
While Go encourages returning errors, there are situations where you might want to handle truly unexpected scenarios differently, using panic
and recover
. A panic
typically indicates a programmer error that should not be caught and handled normally. You can use recover
to manage panics and allow the program to continue running.
package main
import (
"fmt"
)
func safeDivide(a, b float64) {
defer func() {
if err := recover(); err != nil {
fmt.Println("Recovered from panic:", err)
}
}()
if b == 0 {
panic("division by zero")
}
fmt.Println("Result:", a/b)
}
func main() {
safeDivide(10, 0)
fmt.Println("Execution continues!")
}
In the example above, the safeDivide
function uses defer
with an anonymous function to recover from a panic. This allows the program to handle the unexpected division by zero gracefully and continue execution.
Best Practices for Error Handling
Effective error management is key to robust and reliable applications. Here are some best practices to keep in mind when handling errors in Go:
- Check errors immediately: Always check and handle errors as soon as they are returned to avoid unexpected behavior further down the line.
- Use descriptive error messages: Provide detailed error messages to make debugging easier. Indicate the source and context of the error.
- Favor returning errors over panicking: Reserve
panic
for truly exceptional situations that cannot be handled gracefully through normal error handling. - Log errors: Always log errors. In production systems, this can be invaluable for diagnosing issues and understanding where things went wrong.
- Use custom error types when necessary: Custom errors provide richer context and make error handling more meaningful.
Conclusion
GoLang's approach to error handling may seem unusual at first, but it brings a number of benefits that contribute to building simple, clear, and reliable code. By treating errors as values and using idiomatic patterns for error checking, you can build applications that are robust and easy to maintain.
If you're looking to dive deeper into GoLang's error handling, don't miss out on this excellent guide from the official Go documentation. Keep experimenting, keep exploring, and remember: every error is just another opportunity to make your code better!
Until next time, keep coding and keep smiling!