Testing in Python: Verifying Code Functions Correctly
In the world of programming, the importance of testing your software cannot be overstated. Testing allows developers to ensure that their code is functioning as intended, and helps to catch any potential errors or bugs. In Python, there are a number of different ways to implement testing, and this guide will discuss why testing is essential, various types of tests, and best practices for testing in Python.
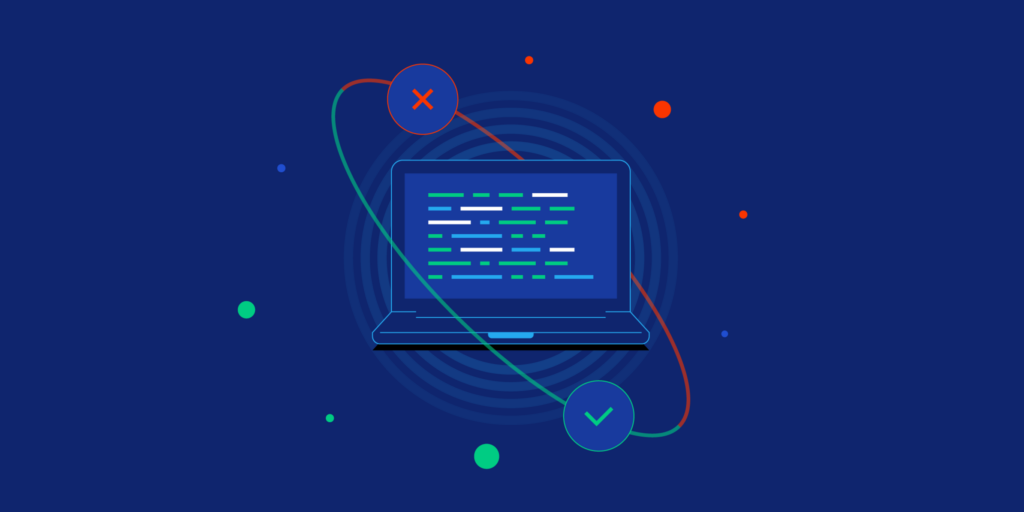
Why Should You Test Your Python Code?
The answer to “why testing?” is simple: Testing verifies that your code works as expected and helps prevent future bugs or issues. It’s no secret that even the most experienced developers can make mistakes. No one writes perfect code, and we are human after all. But, with adequate testing in place, those mistakes become far less damaging and easier to fix.
Testing also paves the way to allow others to contribute to your code. Think about it. Would you want to add to software that has a high risk of breakage or would you rather contribute to one where there are safety nets(checks) in place? It is a no-brainer really.
Other reasons for testing include that they make refactoring safer, provide better design, act as a form of documentation and lead to better user satisfaction because let’s face it, nobody likes a buggy application.
In many ways, testing is like an insurance policy for your code. Now, does the lightbulb make more sense? (You know, because they’re both great ideas!)
Types of Tests in Python Development
Python provides several levels of testing, ranging from low level (unit tests) to high level (integration and functional tests). Each are aimed at helping achieve different goals and ensuring the completeness of your application.
Python’s unit testing framework is an excellent example of a tool that can be utilized to assist this process.
Unit Testing
Unit Testing is essentially the ‘spell check’ of the coding world. Just like how a spell-check helps us catch pesky typos, unit testing pinpoints the smaller errors in our code that could potentially lead to bigger issues down the line.
Unit Tests verify that individual components of the software are working correctly. Check out the following code snippet for a simple example of a unit test in Python:
import unittest
class TestSum(unittest.TestCase):
def test_sum(self):
self.assertEqual(sum([1, 2, 3]), 6, "Should be 6")
def test_sum_tuple(self):
self.assertEqual(sum((1, 2, 2)), 6, "Should be 6")
if __name__ == '__main__':
unittest.main()
In this example, we’re testing the Python built-in function `sum()`
Integration Testing
While unit tests focus on the minutiae of your code, integration tests are there to ensure that multiple components can play nicely together.
Just like how a solo musician can sound flawless on their own, when added to an orchestra, how well they blend can be a different story. Integration tests ensure that the metaphorical ‘orchestra’ of your software sounds harmonious.
Functional Testing
Functional tests are the highest level of testing, and they aim to ensure the software behaves as intended from the user’s perspective. It’s like trying out your newly assembled IKEA furniture, to make sure it not just looks like a chair, but also functions as one.
Best Practices for Testing in Python
Testing can be daunting, especially for beginners, but the rewards are much worth it. When implementing tests in Python, here are a few tips to keep in mind:
– Consistency is key. Make testing a part of your regular development process.
– Test first, then code. This practice, known as Test-Driven Development, helps define clear objectives and prevents overdeveloping.
– Automate testing. Manually running tests can be tedious and time-consuming, so automating these processes can make life much easier.
Testing is an essential part of Python (or any) programming, and implementing it into your routine will greatly improve the quality and reliability of your code. Similar to how we forgot French fries from their Belgian beginnings, don’t forget to run those tests (you can thank me later).