Top 10 Data Structures Every Developer Should Know
Welcome, enthusiastic learners! As we dive into the fascinating world of data structures, you’ll find that understanding these essential building blocks is crucial for every developer. Whether you’re working on a simple application or a complex system, data structures help in organizing and managing data efficiently. Today, we’ll demystify the top 10 data structures – Array, Linked List, Stack, Queue, Binary Tree, Binary Search Tree, Heap, Hash Table, Graph, and Trie – so buckle up and get ready to level up your knowledge!
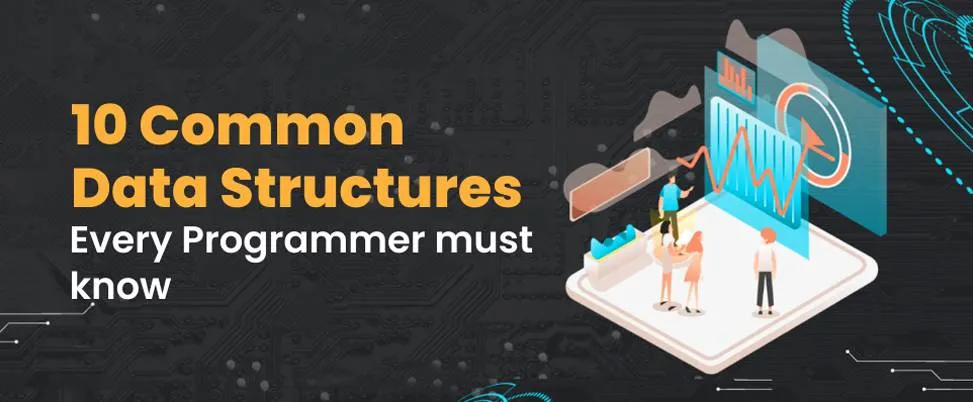
1. Array
The array is one of the simplest and most widely used data structures. It stores elements of the same type in a fixed-size sequential collection.
Advantages:
- Fast access to elements by index.
- Efficient use of memory.
Disadvantages:
- Fixed size – resizing is costly.
- Insertion and deletion are time-consuming unless at the end.
Example:
int[] numbers = {1, 2, 3, 4, 5};
2. Linked List
A Linked List is a collection of nodes where each node contains data and a reference to the next node in the sequence.
Advantages:
- Dynamic size – easy to grow and shrink at runtime.
- Efficient insertions and deletions.
Disadvantages:
- Sequential access – slower than arrays for indexed access.
- Extra memory for storing pointers.
Example:
class Node {
int data;
Node next;
Node(int data) { this.data = data; }
}
Node head = new Node(1);
head.next = new Node(2);
3. Stack
Stack follows Last In First Out (LIFO) principle. You can only insert or remove items from the top of the stack.
Advantages:
- Simple implementation.
- Efficient for algorithms that require temporary storage.
Disadvantages:
- Limited access – operations can only be performed at the top.
Example:
Stack<Integer> stack = new Stack<>();
stack.push(1);
stack.push(2);
int popped = stack.pop(); // 2
4. Queue
Queue follows First In First Out (FIFO) principle. The element that is inserted first is removed first.
Advantages:
- Efficient for scheduling and managing tasks.
Disadvantages:
- Limited access – operations can only be performed at the front or rear.
Example:
Queue<Integer> queue = new LinkedList<>();
queue.add(1);
queue.add(2);
int removed = queue.remove(); // 1
5. Binary Tree
A binary tree is a hierarchical data structure in which each node has at most two children, referred to as the left child and the right child.
Advantages:
- Facilitates hierarchical data representation.
- Efficient for insert, delete, and search operations.
Disadvantages:
- Complex implementation.
Example:
class TreeNode {
int val;
TreeNode left, right;
TreeNode(int x) { val = x; }
}
TreeNode root = new TreeNode(1);
root.left = new TreeNode(2);
root.right = new TreeNode(3);
6. Binary Search Tree (BST)
A BST is a type of binary tree where each node follows the ordering property: To the left of a node are smaller elements, and to the right are larger elements.
Advantages:
- Fast search, insert, and delete operations.
Disadvantages:
- Can become unbalanced, leading to degraded performance.
Example:
class TreeNode {
int val;
TreeNode left, right;
TreeNode(int x) { val = x; }
}
TreeNode root = new TreeNode(3);
root.left = new TreeNode(1);
root.right = new TreeNode(5);
7. Heap
A heap is a specialized binary tree that satisfies the heap property. In a max-heap, for any given node, the value is greater than or equal to its children.
Advantages:
- Efficient for priority queue operations.
Disadvantages:
- Complex implementation.
Example:
PriorityQueue<Integer> maxHeap = new PriorityQueue<>(Collections.reverseOrder());
maxHeap.add(3);
maxHeap.add(1);
int max = maxHeap.poll(); // 3
8. Hash Table
A hash table uses a hash function to map keys to values, allowing for fast data retrieval.
Advantages:
- Very fast data retrieval.
Disadvantages:
- Can suffer from collisions, requiring collision resolution strategies.
Example:
HashMap<String, Integer> hashTable = new HashMap<>();
hashTable.put("One", 1);
hashTable.put("Two", 2);
int value = hashTable.get("One"); // 1
9. Graph
A graph consists of nodes (vertices) and edges connecting pairs of nodes. It can be used to represent networks.
Advantages:
- Great for modeling relationships.
Disadvantages:
- Complex implementation and visualization.
Example:
class Graph {
private int V;
private LinkedList<Integer> adj[];
Graph(int v) {
V = v;
adj = new LinkedList[v];
for (int i = 0; i < v; ++i)
adj[i] = new LinkedList();
}
}
10. Trie
A Trie (pronounced “try”) is a tree-like data structure used to store associative data structures. It’s commonly used for predictive text and autocomplete features.
Advantages:
- Efficient for searching words and prefixes.
Disadvantages:
- Memory-intensive.
Example:
class TrieNode {
Map<Character, TrieNode> children = new HashMap<>();
boolean isEndOfWord = false;
}
class Trie {
private TrieNode root;
public Trie() {
root = new TrieNode();
}
public void insert(String word) {
TrieNode node = root;
for (char c : word.toCharArray()) {
node = node.children.computeIfAbsent(c, k -> new TrieNode());
}
node.isEndOfWord = true;
}
}
There you have it – the top 10 data structures every developer should become familiar with. Mastering these fundamental structures will make you a more efficient and effective coder. To learn more about data structures and algorithms, I highly recommend checking out GeeksforGeeks Data Structures. Keep coding, keep learning, and remember – every great developer was once a beginner. You’re doing fantastic!