Top 10 Algorithms for Beginners
Diving into the world of algorithms can be incredibly exciting! Whether you’re a beginner or have some experience, understanding these foundational algorithms can set you on a path to solve complex problems efficiently. Let’s explore the top 10 algorithms that every aspiring programmer should know.
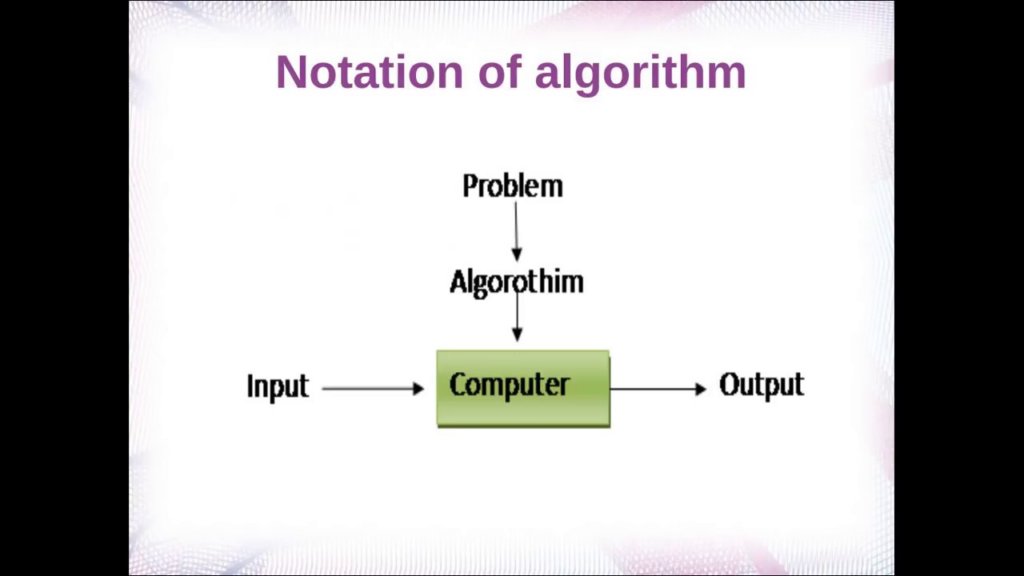
1. Binary Search
Binary Search is a fast and efficient algorithm to find an element in a sorted array. Instead of checking each element one by one, binary search divides the array in half repeatedly to zero in on the target value.
def binary_search(array, target):
low, high = 0, len(array) - 1
while low <= high:
mid = (low + high) // 2
if array[mid] == target:
return mid
elif array[mid] < target:
low = mid + 1
else:
high = mid - 1
return -1
2. Bubble Sort
Bubble Sort is one of the simpler sorting algorithms. It repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order. Though it’s not the most efficient sorting method, it’s a great way to learn about sorting concepts.
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
3. Merge Sort
Merge Sort is an efficient, stable, and comparison-based sorting algorithm. It works on the principle of divide and conquer, breaking the array into halves, sorting them, and merging the sorted halves back together.
def merge_sort(arr):
if len(arr) > 1:
mid = len(arr) // 2
left_half = arr[:mid]
right_half = arr[mid:]
merge_sort(left_half)
merge_sort(right_half)
i = j = k = 0
while i < len(left_half) and j < len(right_half):
if left_half[i] < right_half[j]:
arr[k] = left_half[i]
i += 1
else:
arr[k] = right_half[j]
j += 1
k += 1
while i < len(left_half):
arr[k] = left_half[i]
i += 1
k += 1
while j < len(right_half):
arr[k] = right_half[j]
j += 1
k += 1
return arr
4. QuickSort
QuickSort is another divide and conquer algorithm, but unlike Merge Sort, it works in-place to produce the sorted array. It selects a ‘pivot’ element and partitions the array around the pivot, with values less than the pivot on one side and greater on the other.
def quick_sort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quick_sort(left) + middle + quick_sort(right)
5. Dijkstra’s Algorithm
Dijkstra’s Algorithm finds the shortest path between nodes in a graph. It is widely used in mapping and geographical applications like GPS navigation systems. We wrong about it before here.
6. Floyd-Warshall Algorithm
The Floyd-Warshall Algorithm is another pathfinding algorithm but a bit more powerful. It computes the shortest paths between all pairs of nodes in a weighted graph. It’s useful in scenarios involving routing and network optimizing. also mentioned here.
7. Prim’s Algorithm
Prim’s Algorithm is primarily used for finding the Minimum Spanning Tree (MST) in a graph, ensuring that the total weight of the tree is minimized. This is particularly useful in network design.
8. Kruskal’s Algorithm
Kruskal’s Algorithm also finds the Minimum Spanning Tree of a graph but does so differently. It sorts all the edges of the graph in ascending order and picks the smallest edges, ensuring no cycles are formed. we wrote about it here.
9. Knapsack Problem
The Knapsack Problem is a popular optimization problem which can be solved using dynamic programming. It involves finding the most valuable combination of items without exceeding the weight limit of the knapsack. This relates to resource allocation and logistics.
Here’s a simple python implementation for the 0/1 Knapsack Problem:
def knapsack(weights, values, capacity):
n = len(values)
dp = [[0 for x in range(capacity + 1)] for x in range(n + 1)]
for i in range(n + 1):
for w in range(capacity + 1):
if i == 0 or w == 0:
dp[i][w] = 0
elif weights[i - 1] <= w:
dp[i][w] = max(values[i - 1] + dp[i - 1][w - weights[i - 1]], dp[i-1][w])
else:
dp[i][w] = dp[i - 1][w]
return dp[n][capacity]
10. Tower of Hanoi
The Tower of Hanoi is a classic problem involving three rods and a number of disks of different sizes. The objective is to move all the disks from one rod to another, obeying specific rules. It’s a great exercise in recursion and problem-solving.
def tower_of_hanoi(n , source, destination, auxiliary):
if n==1:
print(f"Move disk 1 from {source} to {destination}")
return
tower_of_hanoi(n-1, source, auxiliary, destination)
print(f"Move disk {n} from {source} to {destination}")
tower_of_hanoi(n-1, auxiliary, destination, source)
Conclusion
Understanding these ten algorithms will surely bolster your problem-solving toolkit. Each serves a unique purpose and getting comfortable with them can make tackling advanced topics much easier. Remember, algorithms are the key to writing efficient code and improving software performance. Happy coding, and may you never stop learning!
For further reading on algorithms and their applications, check out this comprehensive guide on GeeksforGeeks.