Data Types in GoLang: Classifying Types of Data
When delving into GoLang, one of the first concepts you’ll encounter is data types. Understanding data types is essential for writing efficient and error-free code. In this post, we’ll explore the different types of data in GoLang, providing you with hands-on instructions, code snippets, and a sprinkle of humor to keep things interesting!
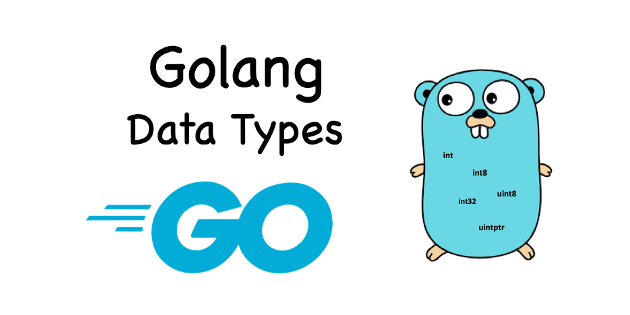
Why Data Types Matter
Data types are fundamental in programming languages as they define the kind of data a variable can hold. In GoLang, data types help in optimizing memory usage and ensuring that operations on variables are type-safe. For anyone coming from a dynamic language like JavaScript, this can feel like having training wheels on your bicycle again, but bear with us—you’ll thank us down the road!
Basic Data Types in GoLang
GoLang offers a variety of basic data types that are classified mainly into numeric, boolean, and string types.
Numeric Types
Numeric types in GoLang are further divided into integer, floating-point, and complex numbers. Here’s a quick rundown:
- Integer: `int`, `int8`, `int16`, `int32`, `int64`, `uint`, `uint8`, `uint16`, `uint32`, `uint64`
- Floating-point: `float32`, `float64`
- Complex numbers: `complex64`, `complex128`
Hands-On Example: Using Integer and Floating-Point Types
Would you rather negotiate with a floating-point number or a stubborn integer? Let’s see how to declare and use these in GoLang.
package main
import "fmt"
func main() {
var age int = 25
var pi float64 = 3.14159
fmt.Printf("Age: %d, Pi: %f\n", age, pi)
}
Save the code above into a file named `main.go` and run it using the following command:
go run main.go
Here’s a complete guide on how to install GoLang.
Boolean Types
Boolean data types can hold one of two values: `true` or `false`. It’s as simple as the chances of you finishing a stack of tutorials without procrastinating—either it happens, or it doesn’t.
Hands-On Example: Using Boolean Types
package main
import "fmt"
func main() {
var isValid bool = true
fmt.Printf("Is the statement valid? %t\n", isValid)
}
String Types
Strings in GoLang are a sequence of bytes and are immutable. You can create strings using double quotes. Concatenating strings feels just like collecting trophies—add one after another.
Hands-On Example: Using String Types
package main
import "fmt"
func main() {
var greeting string = "Hello, GoLang!"
fmt.Println(greeting)
}
Composite Data Types
GoLang also has composite data types for more complex data structures. These include arrays, slices, maps, and structs.
Arrays and Slices
Arrays have a fixed size, whereas slices are dynamically-sized. Just think of arrays as your old-fashioned landline and slices as your smartphone—both can make calls, but one is a lot more flexible.
Hands-On Example: Using Arrays and Slices
package main
import "fmt"
func main() {
var numbers [5]int = [5]int{1, 2, 3, 4, 5}
numbersSlice := numbers[1:3]
fmt.Println("Array:", numbers)
fmt.Println("Slice:", numbersSlice)
}
Maps
Maps are GoLang’s built-in hash table implementation, using key-value pairs for quick data retrieval. What’s quicker, a direct record from a hash table or finding Waldo? You get the point.
Hands-On Example: Using Maps
package main
import "fmt"
func main() {
var employee = map[string]int{"Alice": 25, "Bob": 30}
fmt.Println("Employee Age:", employee["Alice"])
}
Structs
Structs are used to group related data together. Think of them as the luggage compartments of an airplane—organized and everything neatly packed.
Hands-On Example: Using Structs
package main
import "fmt"
type Person struct {
Name string
Age int
}
func main() {
var person = Person{Name: "John", Age: 29}
fmt.Println("Person:", person)
}
Conclusion
Understanding data types is fundamental for programming in GoLang. From integers and booleans to arrays, slices, and structs, mastering these will make you a more effective programmer.
If Go data types were an exam, you’ve now got an A+! And remember, just like in life, always choose the right type for the right situation.
For a more comprehensive understanding, you can always refer to the official GoLang documentation.