Data Types in Python: Classifying Types of Data
Welcome, fellow Python enthusiasts! Ah, Python—the language that’s loved for its simplicity and power. One of the things that makes Python so intuitive and user-friendly is its robust system of data types. No matter if you’re a seasoned developer or someone just starting, understanding the various data types helps you write efficient and effective code. So, today, let’s embark on a fun journey through the diverse universe of data types in Python.
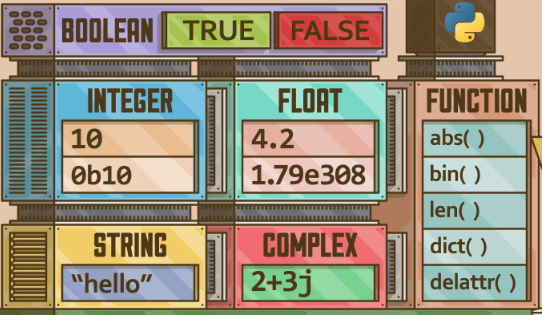
Before we dive in, if you’re new to Python or programming in general, don’t worry. This post is designed to be both informative and entertaining. So, grab your virtual popcorn (or maybe just a cup of coffee) and let’s get started!
1. Numeric Data Types
First up, we have Numeric data types. These are used to store numbers and play a critical role in computations. Python supports three distinct numeric types:
1.1 Integers (int)
Integers are whole numbers, both positive and negative, without any decimal point. They can range from a meager zero to the largest values your system can handle.
age = 25
temperature_on_mars = -120
1.2 Floating Point Numbers (float)
Floats are numbers that contain a decimal point or are expressed in exponential (scientific) notation. They are used when more precision is required.
pi = 3.14159
e = 2.71828
1.3 Complex Numbers (complex)
Python also supports complex numbers, a combination of real and imaginary numbers represented by adding a ‘j’ at the end.
complex_num = 1 + 2j
2. Sequence Data Types
Sequences are ordered collections of items and can include strings, lists, and tuples. They are incredibly versatile and built for efficiency.
2.1 Strings (str)
Strings in Python are arrays of bytes representing Unicode characters. They are used for text manipulation.
greeting = "Hello, World!"
2.2 Lists (list)
Lists are mutable sequences, which means they can be modified after their creation. You can store different data types within a single list.
shopping_list = ["milk", "bread", "eggs"]
2.3 Tuples (tuple)
Tuples are immutable sequences, meaning once created, they cannot be altered. They are often used to store related pieces of information.
person = ("John Doe", 30, "New York")
3. Mapping Data Types
Mapping types map hashable values to corresponding values. Python offers a built-in dictionary type for this purpose.
Dictionaries (dict)
Dictionaries are collections of key-value pairs, providing a way to map unique keys to their respective values.
phonebook = {"Alice": "123-456-7890", "Bob": "234-567-8901"}
4. Set Data Types
Sets are collections of unique elements. They are great for membership testing and eliminating duplicates.
4.1 Sets (set)
Sets are unordered collections with no duplicate elements.
fruits = {"apple", "banana", "cherry"}
4.2 Frozen Sets (frozenset)
Frozen sets are immutable versions of sets, used when you want a set that cannot be changed.
immutable_fruits = frozenset(["apple", "banana", "cherry"])
5. Boolean Data Type
Booleans represent one of two values: True or False. They are essential in controlling the flow of programs.
is_python_fun = True
6. None Type
None is a special data type in Python that represents the absence of a value or a null value.
no_value = None
Conclusion
Congratulations! You’ve just navigated the intriguing world of Python data types. Now knowing the varied data types available, you can categorize your data more efficiently and write programs that are both robust and elegant.
If you want to continue your Python journey, there’s a treasure trove of information available online. One excellent resource is the official Python documentation, which provides in-depth details on all the data types and much more.
Remember, the key to mastering programming is persistent practice. So, keep coding, keep experimenting, and most importantly, have fun!
Stay curious, stay inspired, and until next time—happy coding!