Unlocking the Power of Variables in Python: Storing Data for Manipulation
Hello, Python enthusiasts! Today, we’re diving into the world of variables in Python. These little data holders are the backbone of any programming language, acting like jars to store our data and manipulable items. Whether you’re a novice or a seasoned coder, understanding variables is crucial for writing clean, efficient, and effective code. So let’s break it down!
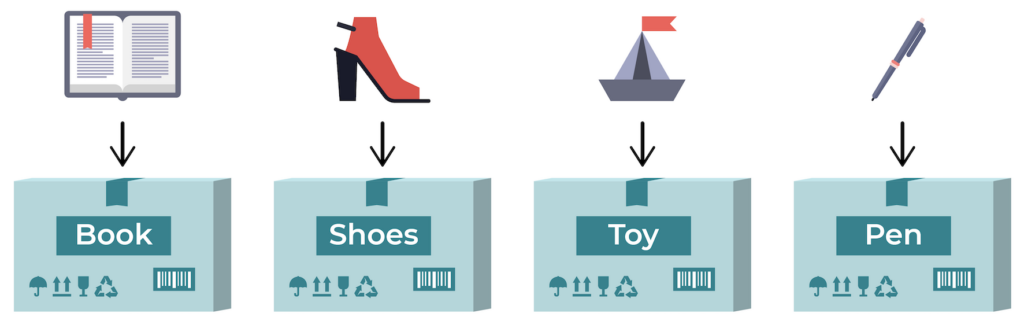
What are Variables in Python?
In simple terms, a variable in Python is a named location used to store data in the memory. When you create a variable, you reserve some space in the memory. Better yet, you can reuse this space to hold different types of data throughout the lifecycle of your program, making variables extremely versatile.
Why Use Variables?
Variables are fundamental in any coding effort for several reasons:
- Data Storage: Stores data that your program needs to function.
- Data Manipulation: Easily change, update, and manipulate data.
- Code Readability: Makes your code cleaner and easier to read.
- Efficiency: Reduces redundancy and keeps your code DRY (Don’t Repeat Yourself).
Declaring a Variable in Python
One of the coolest things about Python is how simple it is to declare a variable. There’s no need for a special keyword like var
or int
as you would find in other languages. Just use an assignment operator (=
) to get started. Let’s look at some examples:
name = "Alice"
age = 25
is_student = True
Data Types
Python is a dynamically-typed language, which means you don’t have to declare the type of variable explicitly. Here are some common data types you might use:
- Integers: Whole numbers, e.g.,
number = 42
- Floating-point numbers: Decimal numbers, e.g.,
pi = 3.14
- Strings: Text, e.g.,
greeting = "Hello, World!"
- Booleans: True/False values, e.g.,
is_active = True
- Lists: Ordered, mutable collections of items, e.g.,
colors = ["red", "blue", "green"]
Changing Variable Values
Variables in Python are mutable, meaning you can change their value as your program runs. This flexibility allows you to manipulate data effectively. Here’s an example:
counter = 10
print(counter) # Output: 10
counter = counter + 5
print(counter) # Output: 15
Multiple Assignment
Python also allows for multiple assignments in a single line, which can come in handy for initializing multiple variables at once. Check this out:
a, b, c = 1, 2, "Hello"
print(a) # Output: 1
print(b) # Output: 2
print(c) # Output: Hello
Constants: When to Use Them
While constants (variables meant to remain unchanged) are not natively enforced in Python, you can still follow naming conventions to signify that a variable should not change. This is usually done by using uppercase letters:
PI = 3.14159
MAX_USERS = 100
Scope of Variables
When dealing with variables, it’s essential to understand their scope. The scope of a variable determines where that variable can be accessed or modified. In Python, there are mainly two types of scopes:
- Local Scope: Variables declared within a function. They can only be used inside that function.
- Global Scope: Variables declared outside any function. They can be accessed anywhere in the program.
Here’s an example to illustrate the concept of variable scope:
def my_function():
local_var = "I'm local"
print(local_var)
global_var = "I'm global"
my_function()
print(global_var)
# This will cause an error
# print(local_var)
Best Practices for Naming Variables
Here are some best practices when it comes to naming variables in Python:
- Meaningful Names: Name your variables based on their purpose, e.g.,
user_age
instead ofua
. - Use underscores: Separate words with underscores for readability, e.g.,
total_price
. - Avoid reserved keywords: Don’t use Python’s reserved keywords or built-in function names (e.g.,
list
,str
). - Consistent Naming Conventions: Stick to one naming convention throughout your code.
Conclusion
Variables are the bread and butter of any programming language, and Python makes it incredibly easy to work with them. From storing data to manipulating it, they are indispensable tools that can significantly improve your coding efficiency and readability. Whether you’re a beginner or an experienced developer, mastering variables can elevate your Python skills to new heights.
Curious to learn more about Python? Check out Python’s official documentation for a deeper dive into this versatile language.
Until next time, happy coding!