Using Terraform to Create AWS Resources with Dependencies
If you’re stepping into the world of cloud infrastructure, you’ve likely heard of Terraform. This powerful open-source infrastructure-as-code (IaC) tool can automate the provisioning of your infrastructure in the cloud. Today, we’re diving into creating AWS resources with Terraform, focusing on scenarios where one resource depends on another.
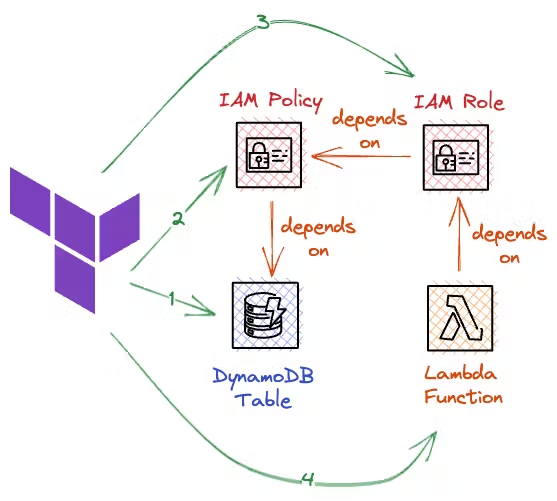
Why Use Terraform?
Let’s highlight a few key advantages of using Terraform:
- Consistency: Ensure your infrastructure is always in the desired state.
- Scalability: Manage complex environments with ease.
- Version Control: Track changes and collaborate efficiently.
- Provisioning Speed: Automate resource allocation to save time.
Alright, enough talking! Let’s jump into how to use Terraform to create AWS resources, with a focus on resource dependencies.
Setting Up Your Terraform Environment
First, ensure you have Terraform installed on your machine. If not, follow the installation guide from the official Terraform documentation.
Creating the Main Configuration File
We start by creating a directory for our project and a main configuration file.
mkdir terraform-project
cd terraform-project
touch main.tf
In the main.tf
file, let’s set up our AWS provider:
provider "aws" {
region = "us-west-2"
}
Defining Resources with Dependencies
Let’s consider a scenario where you need to create an S3 bucket and an IAM user with access to that bucket. Here’s how you can achieve this with Terraform:
1. Create an S3 Bucket
resource "aws_s3_bucket" "example_bucket" {
bucket = "my-unique-bucket-name"
acl = "private"
}
2. Create an IAM User
resource "aws_iam_user" "example_user" {
name = "example-user"
}
3. Attach a Policy to the IAM User
We now create a policy that allows the IAM user to interact with the S3 bucket. However, the policy resource must depend on both the bucket and the user being created first. Here’s how to do it:
resource "aws_iam_policy" "example_policy" {
name = "example-policy"
description = "A policy to access S3 bucket"
policy = jsonencode({
Version = "2012-10-17"
Statement = [
{
Action = ["s3:*"]
Effect = "Allow"
Resource = [
aws_s3_bucket.example_bucket.arn,
"${aws_s3_bucket.example_bucket.arn}/*"
]
}
]
})
depends_on = [
aws_s3_bucket.example_bucket,
aws_iam_user.example_user
]
}
4. Attach the Policy to the User
Finally, attach the policy to the user:
resource "aws_iam_user_policy_attachment" "user_policy_attachment" {
user = aws_iam_user.example_user.name
policy_arn = aws_iam_policy.example_policy.arn
}
Applying the Configuration
Let’s apply these configurations! First, initialize your Terraform project:
terraform init
Next, generate an execution plan:
terraform plan
Finally, apply the plan to create your resources:
terraform apply
Type yes
when prompted to confirm the operation. Congratulations! You’ve just provisioned an S3 bucket with an IAM user that has permission to access the bucket, all while ensuring that the IAM policy depends on the successful creation of the other resources.
Cleaning Up Resources
When you’re done testing, you can clean up all the resources by running:
terraform destroy
Type yes
to confirm the deletion of all resources defined in your main.tf
file.
Conclusion
Using Terraform to manage AWS resources can significantly boost your productivity and ensure your infrastructure is reliable and replicable. By defining dependencies explicitly, you can avoid common pitfalls and ensure that your infrastructure is provisioned in the right order. For more detailed information, check the official AWS Terraform provider documentation.
Remember, the only limit is your imagination! Keep exploring, keep automating, and happy building!