Using Terraform to Create MS Azure Resources with Dependencies
If you’ve ever played Jenga, you know that building something stable takes planning; it’s no different when creating resources in Azure using Terraform. Today, we’re going to dive deep into using Terraform to create Azure resources with dependencies between them. We’ll walk through the code, provide clear instructions, and even throw in a joke or two to keep things light. Ready? Let’s Jenga-fy our Azure infrastructure!
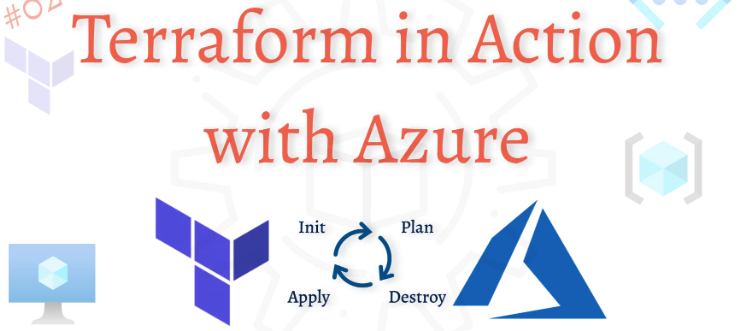
What is Terraform?
Terraform by HashiCorp is an open-source tool that allows you to define and provision infrastructure as code. Think of Terraform as your infrastructure’s version of IKEA instructions. Only, it won’t make you lose random screws under the couch.
Why Use Terraform for Azure?
Why Terraform and Azure are like peanut butter and jelly:
– Consistency: Keep your infrastructure consistent across environments.
– Speed: Deploy complex infrastructure with fewer mistakes in a fraction of the time.
– Version Control: Track changes over time, just like you would with any source code.
Getting Started with Terraform
Before diving in, you’ll need to install Terraform and configure it to talk to Azure.
Step 1: Install Terraform
Head over to [Terraform Downloads](https://www.terraform.io/downloads.html) to get the installation package for your operating system. For instance, on macOS, you could use Homebrew:
brew install terraform
Step 2: Authenticate Terraform with Azure
First, install the Azure CLI:
curl -sL https://aka.ms/InstallAzureCLIDeb | sudo bash
Next, log in to your Azure account:
az login
To ensure everything is working, you can run:
az account show
Creating Resources with Dependencies
Let’s say we want to create an Azure Resource Group and then deploy a Virtual Network within that Resource Group—classic dependency scenario!
Step 1: Define Resource Group
Create a new directory for your Terraform project and navigate into it:
mkdir terraform-azure-demo && cd terraform-azure-demo
Create a file named `main.tf` and add the following code to define the Resource Group:
provider "azurerm" {
features {}
}
resource "azurerm_resource_group" "example" {
name = "example-resources"
location = "West Europe"
}
Step 2: Define Virtual Network Dependent on Resource Group
Add the code for the Virtual Network, ensuring it depends on the Resource Group:
resource "azurerm_virtual_network" "example" {
name = "example-virtual-network"
address_space = ["10.0.0.0/16"]
location = azurerm_resource_group.example.location
resource_group_name = azurerm_resource_group.example.name
}
Voila! You’ve just defined dependencies where the Virtual Network depends on the Resource Group.
Step 3: Initialize and Apply Configuration
First, let’s initialize Terraform in your directory:
terraform init
Now, let’s apply the configuration:
terraform apply
Review the plan generated by Terraform and type `yes` to confirm the action. Now sit back and watch as Terraform does the heavy lifting.
Advanced Dependencies
Maybe you’re planning to build a house of cards on top of your stack, like adding Subnets and Network Security Groups. Let’s add those in!
Adding Subnets
resource "azurerm_subnet" "example" {
name = "example-subnet"
resource_group_name = azurerm_resource_group.example.name
virtual_network_name = azurerm_virtual_network.example.name
address_prefixes = ["10.0.1.0/24"]
}
Adding a Network Security Group
resource "azurerm_network_security_group" "example" {
name = "example-nsg"
location = azurerm_resource_group.example.location
resource_group_name = azurerm_resource_group.example.name
}
Linking Subnets with Network Security Group
resource "azurerm_subnet_network_security_group_association" "example" {
subnet_id = azurerm_subnet.example.id
network_security_group_id = azurerm_network_security_group.example.id
}
Now you’ve got an expanded configuration with various dependencies neatly tied together. Isn’t it satisfying to see everything just click into place? Like finding that last piece of the puzzle.
Debugging and Tips
Working with Terraform sometimes feels like wrestling with a bear, but there are ways to make it smoother:
– Use `terraform plan` frequently: Always preview changes before applying them.
– State Management: Understand and manage your Terraform state. For complex setups, consider using a remote state back-end.
– Modularity: Break your configurations into modules to keep them manageable.
Conclusion
Congratulations! You’ve now learned how to use Terraform to create Azure resources with dependencies. By splitting your tasks and understanding how resources interlink, you make your configurations more robust and easier to manage. And remember, Terraform, like a good joke, is all about the setup and the punchline. Keep exploring, keep automating, and never stop building—that’s the true spirit of DevOps.
For more insights and advanced techniques, check out the Terraform Documentation.
So what’s next? Maybe you’re ready to inject some CI/CD pipelines into your Terraform workflows. Or perhaps, you’re curious about multi-cloud setups. Whatever it is, I’ll be here—sharing, coding, and deploying, one laugh at a time!