Variables in GoLang: Storing Data for Manipulation
Welcome, fellow tech enthusiasts! Today, we’re diving into the world of GoLang, particularly focusing on one of the fundamental building blocks of any programming language—variables. Variables are essential in all programming languages as they allow you to store and manipulate data. In this post, we’ll uncover how to declare, assign, and work with variables in GoLang with plenty of examples to get you started on your coding journey. Let’s dive in!
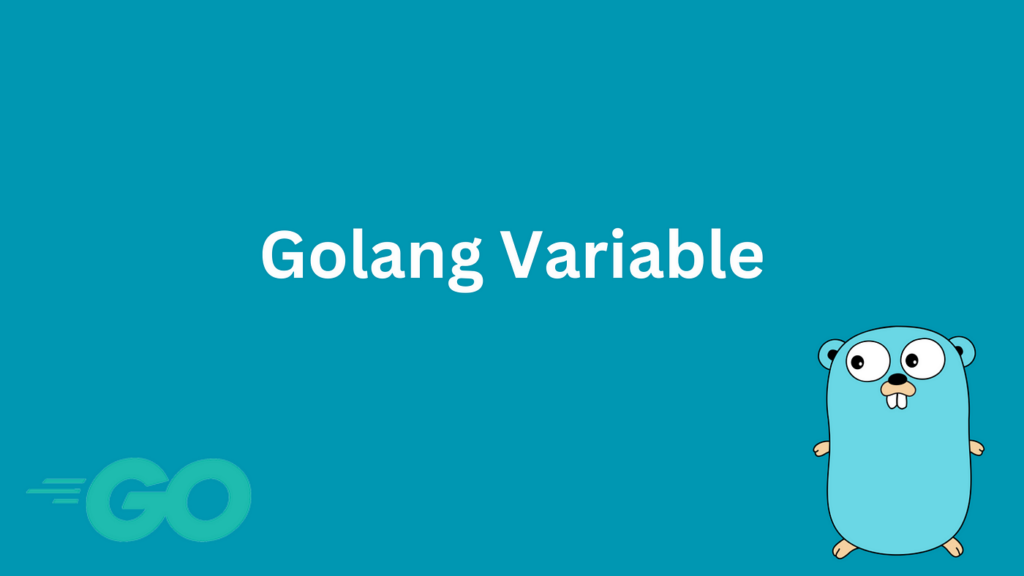
Understanding Variables
Variables are placeholders for storing data values. They have a name (identifier) and a data type that determines what kind of data they can hold. In GoLang, variables can hold data types like integers, floats, strings, and Booleans, to name a few. Let’s see how we can declare and initialize variables in GoLang.
Declaring Variables
There are several ways to declare variables in GoLang. The most common method is to use the var
keyword followed by the variable name and the data type. Here’s an example:
var age int
var name string
var isVerified bool
This snippet declares three variables: age
as an integer, name
as a string, and isVerified
as a Boolean. Note that these variables are declared but not initialized; they hold the zero value for their data type (0 for int, an empty string for string, and false for bool).
Initializing Variables
To assign an initial value to a variable, you can follow the declaration with an assignment operator =
and the value. For instance:
var age int = 25
var name string = "Thomas"
var isVerified bool = true
GoLang also supports type inference, meaning you can skip specifying the type when initializing the variable with a value. GoLang will automatically determine the variable type based on the assigned value:
var age = 25
var name = "Thomas"
var isVerified = true
Short Variable Declaration
When you’re inside a function, GoLang offers a shorthand for variable declaration and initialization using the :=
operator. This method is concise and commonly used:
age := 25
name := "Thomas"
isVerified := true
Note that the short declaration can only be used within function bodies. Attempting to use it at the package level will result in an error.
Multiple Variable Declarations
GoLang allows you to declare multiple variables in a single line, separated by commas. This can be particularly convenient and helps keep your code clean and readable:
var age, height int = 25, 170
var name, city = "Thomas", "New York"
Using the shorthand syntax:
age, height := 25, 170
name, city := "Thomas", "New York"
Constants
Sometimes, you may need a variable whose value won’t change throughout the program. For this purpose, GoLang provides const
for declaring constants:
const pi float64 = 3.14159
const hello = "Hello, World!"
Constants are a great way to make your code more self-explanatory and less error-prone. They also increase its maintainability.
Variable Scope
In GoLang, the scope of a variable defines where in the code the variable is accessible and modifiable. We have three types of scopes:
1. Package Level Scope
Variables declared outside of any function have package-level scope, meaning they’re accessible from any function within the same package.
2. Function Level Scope
Variables declared inside a function are only accessible within that function. They are not visible or accessible to other functions.
3. Block Level Scope
Variables declared inside a block (defined by curly braces {}
) are only accessible within that block. Blocks can be part of functions, loops, or conditionals.
Best Practices
As you work with variables in GoLang, keep these best practices in mind:
- Use meaningful names: Opt for variable names that convey their purpose. Avoid vague names like
x
ory
. - Avoid global variables: Unless absolutely necessary, try to minimize the use of package-level variables to prevent unintended side effects.
- Leverage constants: Use
const
for values that shouldn’t change. It improves code readability and maintainability. - Variable shadowing: Be cautious of variable shadowing, where a variable declared inside a block (inner scope) hides a variable with the same name in an outer scope.
Conclusion
And there you have it! A comprehensive look at variables in GoLang. Variables are the core of any program, enabling you to store, manage, and manipulate data efficiently. Whether you’re a novice or a seasoned coder, mastering variables is a crucial step in becoming proficient in GoLang. Keep exploring, experimenting, and, of course, coding!
If you’re keen to learn more about GoLang, make sure to check out the official GoLang tutorial. Happy coding!
Stay tuned for more exciting content as we continue exploring the fascinating world of GoLang and other tech innovations. Until next time, keep coding and stay curious!